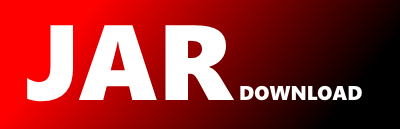
net.sf.jcmdlineparser.options.AbstractOption Maven / Gradle / Ivy
Show all versions of jcmdlineparser Show documentation
/**********************************************************************
Copyright (c) 2009-2010 Alexander Kerner. All rights reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
***********************************************************************/
package net.sf.jcmdlineparser.options;
import java.util.LinkedHashSet;
import java.util.Set;
import net.sf.jcmdlineparser.ParserException;
/**
*
* An {@code AbstractOption} represents a command line option.
*
*
* @author Alexander Kerner
* @version 2010-11-24
*
*/
public abstract class AbstractOption {
/**
*
* String based identifier for this {@code AbstractOption}.
*
*/
protected final String identifierLong;
/**
*
* Character based identifier for this {@code AbstractOption}.
*
*/
protected final char identifierShort;
/**
*
* Description for this {@code AbstractOption}.
*
*/
protected final String description;
/**
*
* Return type of option value(s).
*
*/
protected final Class returnType;
/**
*
* This-option-is-required-flag.
*
*/
protected volatile boolean required;
/**
*
* This-option-is-set-flag.
*
*/
protected volatile boolean set = false;
/**
*
* Other {@code AbstractOption}s that clash (cannot coexist) with this
* {@code AbstractOption}.
*
*/
protected final Set> clashOptions = new LinkedHashSet>();
/**
*
* Other {@code AbstractOptions} that are required by this
* {@code AbstractOption}.
*
*/
protected final Set> requiredOptions = new LinkedHashSet>();
/**
* Construct a new {@code AbstractOption} that has no options it requires
* and no options that are clashing.
*
* @param returnType return type of value(s)
* @param identifierShort character based identifier for this option
* @param identifierLong string based identifier for this option
* @param description description for this option
* @param required true, if this option is required; false otherwise
*/
protected AbstractOption(Class returnType, char identifierShort,
String identifierLong, String description, boolean required) {
this(returnType, identifierShort, identifierLong, description,
required, null, null);
}
/**
* Construct a new {@code AbstractOption}.
*
* @param returnType return type of value(s)
* @param identifierShort character based identifier for this option
* @param identifierLong string based identifier for this option
* @param description description for this option
* @param required true, if this option is required; false otherwise
* @param clashOptions options that are clashing; may be null
* @param requiredOptions options that are required; may be null
*/
protected AbstractOption(Class returnType, char identifierShort,
String identifierLong, String description, boolean required,
Set> clashOptions,
Set> requiredOptions) {
synchronized (AbstractOption.class) {
if (returnType == null)
throw new NullPointerException();
if (!Character.isLetterOrDigit(identifierShort))
throw new IllegalArgumentException(
"identifier must be a letter or digit");
this.returnType = returnType;
this.identifierShort = identifierShort;
if (identifierLong != null)
this.identifierLong = identifierLong;
else
this.identifierLong = Character.toString(identifierShort);
this.description = description;
this.required = required;
if (clashOptions != null && !clashOptions.isEmpty())
this.clashOptions.addAll(clashOptions);
if (requiredOptions != null && !requiredOptions.isEmpty())
this.requiredOptions.addAll(requiredOptions);
}
}
// Override //
public synchronized int hashCode() {
int r = 17;
r = 31 * r + identifierShort;
return r;
}
public synchronized String toString() {
return identifierLong + "(" + identifierShort + ")";
}
public synchronized boolean equals(Object o) {
if(this == o)
return true;
if(o == null)
return false;
if (o instanceof AbstractOption>) {
AbstractOption> cp = (AbstractOption>) o;
if (cp.identifierLong.equals(this.identifierLong)
|| cp.identifierShort == this.identifierShort)
return true;
}
return false;
}
// Public //
/**
*
*
* Defines another {@code AbstractOption}, that will exclude this
* {@code AbstractOption} to be set and vice versa. Calling this method will
* also call {@link AbstractOption#addClashOption(AbstractOption)} on
* {@code clashOption}.
*
*
* @param clashOption
* {@code AbstractOption} that clashes with this option
*/
public synchronized void addClashOption(AbstractOption> clashOption) {
if (clashOptions.add(clashOption))
clashOption.addClashOption(this);
}
/**
*
* Gets all clashing {@code AbstractOption}s for this {@code AbstractOption}
* , that means all {@code AbstractOption} that cannot coexist with this
* {@code AbstractOption}.
*
*
* @return a {@code Set} of {@code AbstractOption}'s that clash with this
* {@code AbstractOption}
*/
public synchronized Set> getClashOptions() {
return new LinkedHashSet>(clashOptions);
}
/**
*
* Gets all required {@code AbstractOption} for this {@code AbstractOption},
* that means all {@code AbstractOption}s that must be set if this
* {@code AbstractOption} is set.
*
*
* @return a {@code Set} of {@code AbstractOption}'s that are required by
* this option
*/
public synchronized Set> getRequiredOptions() {
return new LinkedHashSet>(requiredOptions);
}
/**
*
*
* Defines another {@code AbstractOption} that is required by this
* {@code AbstractOption}.
*
*
* @param requiredOption
* {@code AbstractOption} that is required by this
* {@code AbstractOption}
*/
public synchronized void addRequiredOption(AbstractOption> requiredOption) {
if (requiredOptions.add(requiredOption)) {
if(this.isSet())
requiredOption.setRequired(true);
} else {
System.err.println("required option \"" + requiredOption
+ "\" was already registered");
if (!requiredOption.isRequired())
throw new RuntimeException("Something went badly wrong.");
}
}
/**
*
* @return the long identifier ({@code String} representation) for this
* {@code AbstractOption}
*/
public String getKeyLong() {
return identifierLong;
}
/**
*
* @return the short identifier ({@code character} representation) for this
* {@code AbstractOption}
*/
public char getKeyShort() {
return identifierShort;
}
/**
*
* @return the description for this {@code AbstractOption}
*/
public String getDescription() {
return description;
}
/**
*
* @return true, if this {@code AbstractOption} was set on the command line;
* false otherwise
*/
public boolean isSet() {
return set;
}
/**
*
* Marks this {@code AbstractOption} as set on the command line
*
*
* @param set
* true if this {@code AbstractOption} was set on the command
* line, false otherwise
*/
protected void setSet(boolean set) {
this.set = set;
}
protected void setRequired(boolean required) {
this.required = required;
}
/**
*
* @return true, if this {@code AbstractOption} has been declared to be
* explicitly required
*/
public boolean isRequired() {
return required;
}
/**
* Retrieves return type of this option's value(s).
*/
public Class getReturnType() {
return returnType;
}
// Abstract //
/**
*
* Parses value(s) for this {@code AbstractOption}.
*
*
* @param string
* String that is parsed
* @throws NullPointerException
* if given String is null
* @throws ParserException
* if no valid values can be parsed from this string
*/
public abstract void parse(String string) throws ParserException;
}