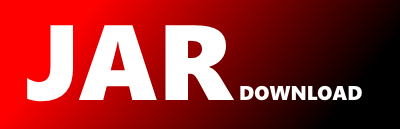
net.sf.jcmdlineparser.parser.CmdLineParser Maven / Gradle / Ivy
Show all versions of jcmdlineparser Show documentation
/**********************************************************************
Copyright (c) 2009-2010 Alexander Kerner. All rights reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*********************************************************************/
package net.sf.jcmdlineparser.parser;
import java.io.OutputStream;
import net.sf.jcmdlineparser.ParserException;
import net.sf.jcmdlineparser.options.AbstractOption;
import net.sf.jcmdlineparser.options.DuplicateOptionIdentifierException;
import net.sf.jcmdlineparser.strategy.Strategy;
/**
*
* An implementation of CmdLineParser
will provide command line
* argument parsing functionality.
*
*
* Parsing logic is delegated to given Strategy
s
*
*
* @author Alexander Kerner
* @version 2010-08-10
* @see Strategy
*
*
*/
public interface CmdLineParser {
/**
*
* Adds a command line option that will be parsed for.
*
*
* @param
* Implementation type of Option. Could be an String
* option or Integer
option, for instance.
* @param o
* {@code AbstractOption} that is parsed for.
* @throws DuplicateOptionIdentifierException
* if provided option is already registered
* @see AbstractOption
*/
void registerOption(AbstractOption o)
throws DuplicateOptionIdentifierException;
/**
*
* Add a (custom) Strategy
for parsing. Most common parsing
* strategies are included by default, such as parsing strategies for
* expressions like this:
* --key=value
*
* --key value1 [value2] [value3] [..]
*
* -v1[v2][v3][..]
*
* Therefore, in most cases, there will be no need to add a further
* strategy.
*
*
* @param s
* Strategy
for parsing.
* @see Strategy
*/
void addStrategy(S s);
/**
*
* Removes all registered parsing strategies from this
* CmdLineParser
.
*
*
* @see Strategy
*/
void removeAllStrategies();
/**
*
* Parses command line arguments. All registered strategies will be used to
* support different command line argument formating.
*
*
* @param args
* Array of String
that is parsed.
* @return Remaining command line arguments, that could not be recognized.
* @throws ParserException
*/
String[] parse(String[] args) throws ParserException;
/**
*
* Parses command line arguments. All registered strategies will be used, to
* support different command line argument formating.
*
*
* @param args
* Single string that is parsed.
* @return Remaining command line arguments, that could not be recognized.
* @throws ParserException
*/
String parse(String args) throws ParserException;
/**
*
* Prints a formatted help text to STDOUT.
*
*/
void printHelp(String usageString);
/**
*
* Prints a formatted help text to out.
*
*
* @param out
* OutputStream help text is printed to.
*/
void printHelp(String usageString, OutputStream out);
}