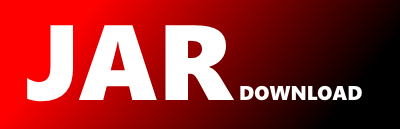
junit.extensions.xml.XMLTestCase Maven / Gradle / Ivy
The newest version!
package junit.extensions.xml;
import junit.framework.Test;
import junit.framework.TestCase;
import org.w3c.dom.Attr;
import org.w3c.dom.Element;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.NodeList;
/**
* Test Case for running XML Script based testing.
*
* @author Kevin Wilson
* @author Vijay Aravamudhan : ThoughtWorks Inc.
*/
public class XMLTestCase extends TestCase implements IXMLTestCase,
XMLConstants {
/**
* The element to be processed.
*/
private Element m_element;
/**
* Parent test.
*/
private IXMLTest m_parent;
/**
* The name of the test xml script file - found from the classpath.
*/
private String m_filename;
/**
* Map of the procedures for this test case.
*/
private XMLObjectCache m_procedures = new XMLObjectCache();
/**
* A Map of all objects that have been found - keyed by the string name.
*/
private XMLPropertyCache m_properties = new XMLPropertyCache();
/**
* The default constructor that is needed to createa a test case.
*
* @param filename The name of the test xml script file
* (found from the classpath)
* @param element The Element to be processed
*/
public XMLTestCase(final String filename, final Element element) {
super(filename + ":" + XMLUtil.getPath(element));
m_filename = filename;
m_element = element;
}
/**
* Get the debug state.
* @return true if debug is enabled.
*/
public final boolean getDebug() {
Object str = getProperty(DEBUG);
if (str instanceof String) {
return Boolean.valueOf((String) str).booleanValue();
} else if (str instanceof Boolean) {
return ((Boolean) str).booleanValue();
}
return (m_properties.get(DEBUG) != null);
}
/**
* Set the parent of the test case.
* @param parent of the test case.
*/
public final void setParent(final IXMLTest parent) {
this.m_parent = parent;
m_properties.setParent(((IXMLTest) parent).getPropertyCache());
m_procedures.setParent(((IXMLTest) parent).getProcedureCache());
}
/**
* Get a procedure definition.
* @param name Name of the procedure.
* @return IXMLProcedure defined by name.
*/
public final IXMLProcedure getProcedure(final String name) {
return (IXMLProcedure) m_procedures.get(name);
}
/**
* Get the procedure cache.
* @return XMLObjectCache which contains the procedure.
*/
public final XMLObjectCache getProcedureCache() {
return m_procedures;
}
/**
* Retrieve the object that was found previously.
*
* @param name The name of the object that was found
* @return The object to be retrieved
*/
public final Object getProperty(final String name) {
return m_properties.get(name);
}
/**
* Get the property cache.
* @return XMLObjectCache containing the properties.
*/
public final XMLObjectCache getPropertyCache() {
return m_properties;
}
/**
* Get the name of a component which has been found.
* @param comp Component to locate
* @return The name of the component.
*/
public final String getPropertyName(final Object comp) {
return m_properties.getName(comp);
}
/**
* Get the names of the found objects.
* @return array containing the names of the objects.
*/
public final String[] getPropertyNames() {
return m_properties.getNames();
}
/**
* Default setUp which does nothing. Override this to set up the environment
* for your test.
*
* @exception Exception An instance of java.lang.Exception can be thrown
* @see junit.framework.TestCase#setUp()
*/
public void setUp() throws Exception {
super.setUp();
}
/**
* Add a procedure definition.
* @param proc Procedure to be added.
*/
public final void addProcedure(final IXMLProcedure proc) {
m_procedures.put(
proc.getName(),
proc);
}
/**
* Call a procedure by name.
* @param name Name of the procedure to be called.
* @param element Element of the call
* @throws XMLException may be thrown.
*/
public final void callProcedure(final String name, final Element element)
throws XMLException {
XMLPropertyCache cache = m_properties;
m_properties = new XMLPropertyCache();
m_properties.setParent(cache);
NamedNodeMap atts = element.getAttributes();
int size = atts.getLength();
for (int i = 0; i < size; i++) {
Attr node = (Attr) atts.item(i);
String an = node.getName();
if (!CALL.equals(an)) {
String value = XMLUtil.getAttribute(element, an);
value = this.resolveProperties(value);
addProperty(an, value);
}
}
IXMLProcedure proc = getProcedure(name);
this.processChildren(proc.getElement());
m_properties.setParent(null);
m_properties = cache;
}
/**
* Process the child XML Elements.
* @param element Element which has children to be processed.
* @throws XMLException may be thrown during processing.
*/
public final void processChildren(final Element element)
throws XMLException {
// Get the children and add to the suite.
NodeList children = element.getChildNodes();
Element child;
for (int i = 0; i < children.getLength(); i++) {
if (children.item(i) instanceof Element) {
child = (Element) children.item(i);
String name = child.getTagName();
try {
XMLTagResourceBundle.getTagHandler(child, this, name)
.processElement();
} catch (XMLException xe) {
throw xe;
} catch (Throwable t) {
throw new XMLException(
t.getMessage(),
t,
child,
getPropertyCache());
}
if (child != children.item(i)) {
for (;
(child != children.item(i))
&& (i < children.getLength()); i++) {
// Nothing to do;
}
if (i == children.getLength()) {
throw new junit.extensions.xml.XMLException("Lost where we were at current node was removed.",
null,
m_element,
getPropertyCache());
}
}
}
}
}
/**
* Remove the object with the name given.
*
* @param name Name of the object to be removed
*/
public final void removeProperty(final String name) {
m_properties.remove(name);
}
/**
* Resolve embeded property names withing a string.
* @param s String to be resolved.
* @return String without property names.
*/
public final String resolveProperties(final String s) {
return m_properties.resolve(s);
}
/**
* Sets up, executes and then tears down a test.
*
* @exception Throwable exceptions thrown by code.
*/
public void runBare() throws Throwable {
setUp();
try {
runTest();
} finally {
tearDown();
}
}
/**
* This method is the one that actually performs the test by processing the elements.
*
* @exception Exception An instance of java.lang.Exception can be thrown.
*/
public final void runXMLTest() throws Exception {
clearProperties();
boolean debug = XMLUtil.getBooleanAttributeValue(m_element, DEBUG);
if (debug) {
m_properties.put(DEBUG, Boolean.TRUE);
} else {
m_properties.remove(DEBUG);
}
processChildren(m_element);
// XMLTagResourceBundle.getTagHandler(m_element, this, TEST).processElement();
}
/**
* Returns an empty suite.
*
* @return Test An empty XMLTestSuite is returned by default.
*/
public static Test suite() {
return new XMLTestSuite();
}
/**
* Add each found object into the cache map.
*
* @param name The name of the found object.
* @param obj The actual object that was found.
*/
public final void addProperty(final String name, final Object obj) {
m_properties.put(name, obj);
}
/**
* For each test (method) element, the found objects has to be cleared.
*/
public final void clearProperties() {
m_properties.clear();
}
/**
* Default tearDown which does nothing. Override this to tear down the
* environment for your test.
*
* @exception Exception An instance of java.lang.Exception can be thrown
* @see junit.framework.TestCase#setUp()
*/
public void tearDown() throws Exception {
super.tearDown();
}
/**
* Executes a test.
*
* @exception Throwable exceptions thrown by code.
*/
protected void runTest() throws Throwable {
runXMLTest();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy