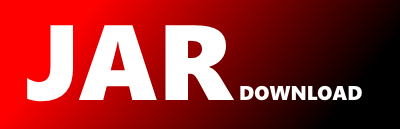
junit.extensions.xml.XMLWriter.bak Maven / Gradle / Ivy
The newest version!
/*
* This XML writer was adapted from the Apache sample software, and
* has undergone the following corrections:
* 1. XML comments are now preserved.
* 2. main method has been removed.
* 3. the package has been changed.
*/
/*
* The Apache Software License, Version 1.1
*
*
* Copyright (c) 1999-2002 The Apache Software Foundation. All rights
* reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in
* the documentation and/or other materials provided with the
* distribution.
*
* 3. The end-user documentation included with the redistribution,
* if any, must include the following acknowledgment:
* "This product includes software developed by the
* Apache Software Foundation (http://www.apache.org/)."
* Alternately, this acknowledgment may appear in the software itself,
* if and wherever such third-party acknowledgments normally appear.
*
* 4. The names "Xerces" and "Apache Software Foundation" must
* not be used to endorse or promote products derived from this
* software without prior written permission. For written
* permission, please contact [email protected].
*
* 5. Products derived from this software may not be called "Apache",
* nor may "Apache" appear in their name, without prior written
* permission of the Apache Software Foundation.
*
* THIS SOFTWARE IS PROVIDED ``AS IS'' AND ANY EXPRESSED OR IMPLIED
* WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE APACHE SOFTWARE FOUNDATION OR
* ITS CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF
* USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT
* OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
* SUCH DAMAGE.
* ====================================================================
*
* This software consists of voluntary contributions made by many
* individuals on behalf of the Apache Software Foundation and was
* originally based on software copyright (c) 1999, International
* Business Machines, Inc., http://www.apache.org. For more
* information on the Apache Software Foundation, please see
* .
*/
package junit.extensions.xml;
import org.w3c.dom.Attr;
import org.w3c.dom.Document;
import org.w3c.dom.DocumentType;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.io.UnsupportedEncodingException;
import java.io.Writer;
/**
* A sample DOM writer. This sample program illustrates how to
* traverse a DOM tree in order to print a document that is parsed.
*
* @author Andy Clark, IBM
*
* @version $Id: XMLWriter.java,v 1.2 2004/05/31 04:50:15 kwilson227 Exp $
*/
public class XMLWriter {
//
// Constants
//
// feature ids
/** Namespaces feature id (http://xml.org/sax/features/namespaces). */
protected static final String NAMESPACES_FEATURE_ID = "http://xml.org/sax/features/namespaces";
/**
* Validation feature id
* (http://xml.org/sax/features/validation).
*/
protected static final String VALIDATION_FEATURE_ID = "http://xml.org/sax/features/validation";
/**
* Schema validation feature id
* (http://apache.org/xml/features/validation/schema).
*/
protected static final String SCHEMA_VALIDATION_FEATURE_ID = "http://apache.org/xml/features/validation/schema";
/**
* Schema full checking feature id
* (http://apache.org/xml/features/validation/schema-full-checking).
*/
protected static final String SCHEMA_FULL_CHECKING_FEATURE_ID = "http://apache.org/xml/features/validation/schema-full-checking";
/**
* Lexical handler property id
* (http://xml.org/sax/properties/lexical-handler).
*/
protected static final String LEXICAL_HANDLER_PROPERTY_ID = "http://xml.org/sax/properties/lexical-handler";
/** Default parser name. */
protected static final String DEFAULT_PARSER_NAME = "dom.wrappers.Xerces";
/** Default namespaces support (true). */
protected static final boolean DEFAULT_NAMESPACES = true;
/** Default validation support (false). */
protected static final boolean DEFAULT_VALIDATION = false;
/** Default Schema validation support (false). */
protected static final boolean DEFAULT_SCHEMA_VALIDATION = false;
/** Default Schema full checking support (false). */
protected static final boolean DEFAULT_SCHEMA_FULL_CHECKING = false;
/** Default canonical output (false). */
protected static final boolean DEFAULT_CANONICAL = false;
//
// Data
//
/** Print writer. */
private PrintWriter m_fOut;
/** Canonical output. */
private boolean m_fCanonical;
//
// Constructors
//
/** Default constructor. */
public XMLWriter() {
}
// ()
/**
* Write the XML file in cannonical form.
* @param canonical true if the file is to be written in cannonical form
*/
public XMLWriter(final boolean canonical) {
m_fCanonical = canonical;
}
/**
* set canonical form of writting.
* @param canonical true if canonical.
*/
public void setCanonical(final boolean canonical) {
m_fCanonical = canonical;
}
/**
* Get the canonical state of the writer.
* @return true if the canonical state is set.
*/
public boolean getCanonical() {
return m_fCanonical;
}
/**
* Sets the output writer.
* @param writer The writer to be used.
**/
public void setOutput(final Writer writer) {
if (writer instanceof PrintWriter) {
m_fOut = (PrintWriter) writer;
} else {
m_fOut = new PrintWriter(writer);
}
}
// setOutput(java.io.Writer)
/**
* Sets the output stream for printing.
* @param stream The output stream the XML will be written to.
* @param encoding The encoding to be used for the output stream.
* @throws UnsupportedEncodingException if the encoding passed is
* not supported.
**/
public void setOutput(final OutputStream stream, final String encoding)
throws UnsupportedEncodingException {
String enc = encoding;
if (enc == null) {
enc = "UTF8";
}
java.io.Writer writer = new OutputStreamWriter(stream, enc);
setOutput(writer);
}
// setOutput(OutputStream,String)
/**
* Writes the specified node, recursively.
* @param node Node to be written.
**/
public void write(final Node node) {
// is there anything to do?
if (node == null) {
return;
}
short type = node.getNodeType();
switch (type) {
case Node.DOCUMENT_NODE:
processDocument(node);
break;
case Node.DOCUMENT_TYPE_NODE:
processDocumentType(node);
break;
case Node.ELEMENT_NODE:
processElement(type, node);
break;
case Node.ENTITY_REFERENCE_NODE:
processEntityRef(node);
break;
case Node.CDATA_SECTION_NODE:
processCData(node);
break;
case Node.TEXT_NODE:
processText(node);
break;
case Node.COMMENT_NODE:
processComment(node);
break;
case Node.PROCESSING_INSTRUCTION_NODE:
processInstructions(node);
break;
default:
processOther(node);
break;
}
}
/**
* Write the string after normalization.
* @param s String to be normalized and printed.
*/
protected void normalizeAndPrint(final String s) {
int len = 0;
if (s != null) {
len = s.length();
}
for (int i = 0; i < len; i++) {
char c = s.charAt(i);
normalizeAndPrint(c);
}
}
// normalizeAndPrint(String)
/**
* Normalizes and print the given character.
* Normalizing converts the special HTML characters to
* there &..; mapping.
*
* @param c The character to be printed.
**/
protected void normalizeAndPrint(final char c) {
switch (c) {
case '<':
m_fOut.print("<");
break;
case '>':
m_fOut.print(">");
break;
case '&':
m_fOut.print("&");
break;
case '"':
m_fOut.print(""");
break;
case '\r':
case '\n':
if (m_fCanonical) {
m_fOut.print("");
m_fOut.print(Integer.toString(c));
m_fOut.print(';');
break;
}
default:
m_fOut.print(c);
}
}
// normalizeAndPrint(char)
/**
* Returns a sorted list of attributes.
* @param attrs The array of attributes to be sorted.
* @return Attr[] The attrs in sorted order.
**/
protected Attr[] sortAttributes(final NamedNodeMap attrs) {
int len = 0;
if (attrs != null) {
len = attrs.getLength();
}
Attr[] array = new Attr[len];
for (int i = 0; i < len; i++) {
array[i] = (Attr) attrs.item(i);
}
for (int i = 0; i < (len - 1); i++) {
String name = array[i].getNodeName();
int index = i;
for (int j = i + 1; j < len; j++) {
String curName = array[j].getNodeName();
if (curName.compareTo(name) < 0) {
name = curName;
index = j;
}
}
if (index != i) {
Attr temp = array[i];
array[i] = array[index];
array[index] = temp;
}
}
return array;
}
// sortAttributes(NamedNodeMap):Attr[]
/**
* Process CDATA nodes.
* @param node Node to be processed.
*/
private void processCData(final Node node) {
if (m_fCanonical) {
normalizeAndPrint(node.getNodeValue());
} else {
m_fOut.print("");
}
m_fOut.flush();
}
/**
* Process Comment Nodes.
* @param node Node to be processed.
*/
private void processComment(final Node node) {
m_fOut.print("");
}
/**
* Process Document Nodes.
* @param node Node to be processed.
*/
private void processDocument(final Node node) {
Document document = (Document) node;
if (!m_fCanonical) {
m_fOut.println("");
m_fOut.flush();
write(document.getDoctype());
}
NodeList list = document.getChildNodes();
for (int i = 0; i < list.getLength(); i++) {
write(list.item(i));
}
}
/**
* Process Document Type nodes.
* @param node Node to be processed.
*/
private void processDocumentType(final Node node) {
DocumentType doctype = (DocumentType) node;
m_fOut.print("');
}
/**
* Process Element node.
* @param type Type of the node.
* @param node Node to be processed.
*/
private void processElement(final short type, final Node node) {
m_fOut.print('<');
m_fOut.print(node.getNodeName());
Attr[] attrs = sortAttributes(node.getAttributes());
for (int i = 0; i < attrs.length; i++) {
Attr attr = attrs[i];
String name = attr.getNodeName();
if (!XMLConstants.JFCFILELOC.equals(name)) {
m_fOut.print(' ');
m_fOut.print(attr.getNodeName());
m_fOut.print("=\"");
normalizeAndPrint(attr.getNodeValue());
m_fOut.print('"');
}
}
NodeList children = node.getChildNodes();
if (children.getLength() == 0) {
m_fOut.print("/>");
m_fOut.flush();
} else {
m_fOut.print('>');
m_fOut.flush();
Node child = node.getFirstChild();
while (child != null) {
write(child);
child = child.getNextSibling();
}
if (type == Node.ELEMENT_NODE) {
m_fOut.print("");
m_fOut.print(node.getNodeName());
m_fOut.print('>');
m_fOut.flush();
}
}
}
/**
* Process EntityRef nodes.
* @param node Node to be processed.
*/
private void processEntityRef(final Node node) {
if (m_fCanonical) {
Node child = node.getFirstChild();
while (child != null) {
write(child);
child = child.getNextSibling();
}
} else {
m_fOut.print('&');
m_fOut.print(node.getNodeName());
m_fOut.print(';');
m_fOut.flush();
}
}
/**
* Process Instruction nodes.
* @param node Node to be processed
*/
private void processInstructions(final Node node) {
m_fOut.print("");
m_fOut.print(node.getNodeName());
String data = node.getNodeValue();
if ((data != null) && (data.length() > 0)) {
m_fOut.print(' ');
m_fOut.print(data);
}
m_fOut.println("?>");
m_fOut.flush();
}
/**
* Process nodes which are not handled any other way.
* @param node Node to be processed.
*/
private void processOther(final Node node) {
}
/**
* Process Text nodes.
* @param node Node to be processed.
*/
private void processText(final Node node) {
normalizeAndPrint(node.getNodeValue());
m_fOut.flush();
}
}
// class Writer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy