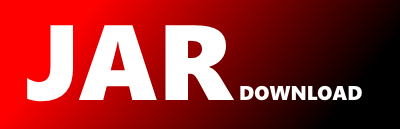
org.igfay.util.MavenFig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jfig Show documentation
Show all versions of jfig Show documentation
A Configuration utility that provides simple, flexible and powerful functionality for managing one or more configurations in a java environment. It uses a combination of a hierarchy of configuration files, substitution variables and property variables.
The newest version!
package org.igfay.util;
import org.apache.log4j.Logger;
import org.igfay.jfig.JFig;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
/**
* @author conrad4
*
* This class brings the power and flexibility of JFig to Maven.
* It takes advantage of the JFig functionality to automatically load selected values as properties.
* You can put your Maven properties in your config file, then use MavenFig to process configuration and call Maven.
*
* This gives you the advantage of storing all your configuration in one central location in
* addition to the additional JFig functionality.
*/
public class MavenFig {
private static Logger logger = Logger.getLogger(MavenFig.class);
private static final String FOREHEAD_CONF_FILE = "forehead.conf.file";
private static final String MAVEN_HOME = "maven.home";
private static final String MAVEN_MAIN_CLASS = "maven.main.class";
private static final String JAVA_ENDORSED_DIRS = "java.endorsed.dirs";
private static final String JAVAX_XML_PARSERS_SAXPARSERFACTORY = "javax.xml.parsers.SAXParserFactory";
private static final String JAVAX_XML_PARSERS_DOCUMENTBUILDERFACTORY = "javax.xml.parsers.DocumentBuilderFactory";
private static final String TOOLS_JAR = "tools.jar";
public static void main(String[] args) {
JFig.getInstance();
String mavenClass = System.getProperty(MAVEN_MAIN_CLASS,"com.werken.forehead.Forehead");
if (!requiredPropertiesAreDefined()) {
logger.fatal("ERROR: You must define certain system properties for MavenFig to operate correctly. " +
"You can use the mavenfig script provided in the jFig distribution to automatically set them, " +
"or you can refer to the maven.config.xml if you want to set these properties via jFig and invoke this class directly. " +
" These properties are required: "
+ FOREHEAD_CONF_FILE + ", "
+ MAVEN_HOME + ", "
+ MAVEN_MAIN_CLASS
+ JAVA_ENDORSED_DIRS + ", "
+ JAVAX_XML_PARSERS_SAXPARSERFACTORY + ", "
+ JAVAX_XML_PARSERS_DOCUMENTBUILDERFACTORY + ", "
+ TOOLS_JAR + ", "
);
return;
}
try {
// Run maven via reflection.
// The Maven bat file has the maven class name parameterized so we maintain that functionality here.
Class clazz = Class.forName(mavenClass);
Object object = clazz.newInstance();
Class[] parameterTypes = new Class[] { String[].class };
Method mainMethod = clazz.getMethod("main", parameterTypes);
Object[] arguments = new Object[] { args };
mainMethod.invoke(object, arguments);
} catch (SecurityException e) {
logger.debug("Exception",e);
} catch (IllegalArgumentException e) {
logger.debug("Exception",e);
} catch (NoSuchMethodException e) {
logger.debug("Exception",e);
} catch (InvocationTargetException e) {
logger.debug("Exception",e);
} catch (InstantiationException e) {
logger.debug("Exception",e);
} catch (IllegalAccessException e) {
logger.debug("Exception",e);
} catch (ClassNotFoundException e) {
logger.debug("Exception",e);
}
}
/**
* Checks if the system properties required by maven are all defined correctly.
*
* @return true if they are defined correctly, false if not.
*/
private static boolean requiredPropertiesAreDefined() {
if (!isPropertyDefined(FOREHEAD_CONF_FILE)) {
return false;
}
if (!isPropertyDefined(JAVAX_XML_PARSERS_DOCUMENTBUILDERFACTORY)) {
return false;
}
if (!isPropertyDefined(JAVAX_XML_PARSERS_SAXPARSERFACTORY)) {
return false;
}
if (!isPropertyDefined(MAVEN_HOME)) {
return false;
}
if (!isPropertyDefined(TOOLS_JAR)) {
return false;
}
return true;
}
/**
* Check a single property for existence.
*
* @param propertyToCheck
* @return true if the property is set, false if not
*/
private static boolean isPropertyDefined(String propertyToCheck) {
if (System.getProperty(propertyToCheck) == null) {
logger.fatal("ERROR: Missing property - " + propertyToCheck);
return false;
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy