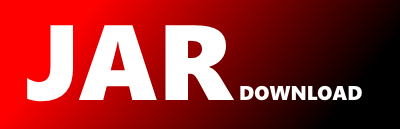
org.igfay.util.Metric Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jfig Show documentation
Show all versions of jfig Show documentation
A Configuration utility that provides simple, flexible and powerful functionality for managing one or more configurations in a java environment. It uses a combination of a hierarchy of configuration files, substitution variables and property variables.
The newest version!
package org.igfay.util;
import java.text.DateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import org.apache.log4j.Level;
import org.apache.log4j.Logger;
import org.igfay.jfig.JFigUtility;
/**
* Capture metrics Creation date: (12/12/2001 9:35:23 AM)
*
*@author bconrad
*@created December 12, 2001
*@author: Bruce Conrad
*/
public class Metric {
private static Logger log = Logger.getLogger(Metric.class);
public final static String DEFAULT = "DEFAULT";
public final static String BENCHMARK = "BENCHMARK";
public final static String DB = "DB";
public final static String EVENT = "EVENT";
public final static String LOGIN = "LOGIN";
public final static String QUERY = "QUERY";
public final static String DOWNLOAD = "DOWNLOAD";
public final static String UPLOAD = "UPLOAD";
public final static String ACCEPTANCE_MANAGER = "ACCEPTANCE_MANAGER";
public final static String NOTIFY_ACTION = "NOTIFY_ACTION";
public final static String RULES_ENGINE = "RULES_ENGINE";
private static java.util.HashMap allGroups;
private long startTime;
private long endTime;
private long pauseStartTime;
private long pauseElapsedTime;
private java.lang.String groupName;
private java.lang.String metricName;
private boolean isDebugEnabled;
/**
* Metric constructor comment.
*/
public Metric() {
this(false);
}
/**
* Metric constructor comment.
*
*@param groupName Description of Parameter
*/
public Metric(String groupName) {
this(groupName, false);
}
/**
* Metric constructor comment.
*
*@param groupName Description of Parameter
*@param metricName Description of Parameter
*/
public Metric(String groupName, String metricName) {
this(groupName, metricName, false);
}
/**
* Metric constructor comment.
*
*@param groupName Description of Parameter
*@param metricName Description of Parameter
*@param isStart Description of Parameter
*/
public Metric(String groupName, String metricName, boolean isStart) {
this(groupName, metricName, isStart, false);
}
public Metric(String groupName, String metricName, boolean isStart, boolean isDebugEnabled) {
this.groupName = groupName;
this.metricName = metricName;
this.setIsDebugEnabled(isDebugEnabled);
// Don't add to group until we have a use for this (and a way to clear the group)
// otw, it will never get garbage collected
//addToListForGroup(groupName, this);
if (isStart) {
start();
}
}
/**
* Metric constructor comment.
*
*@param groupName Description of Parameter
*@param isStart Description of Parameter
*/
public Metric(String groupName, boolean isStart) {
this(groupName, "unnamed", isStart);
}
/**
* Metric constructor comment.
*
*@param isStart Description of Parameter
*/
public Metric(boolean isStart) {
this(DEFAULT, isStart);
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:38:44
* AM)
*
*@param groupName The feature to be added to the ToListForGroup attribute
*@param metric The feature to be added to the ToListForGroup attribute
*/
public static void addToListForGroup(String groupName, Metric metric) {
getListForGroup(groupName).add(metric);
}
/**
* Insert the method's description here. Creation date: (12/12/2001
* 11:03:27 AM)
*
*@param args java.lang.String[]
*/
public static void main(String[] args) {
testSimple();
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:46:19
* AM)
*
*@param groupName Description of Parameter
*/
public static void printGroup(String groupName) {
List list = getListForGroup(groupName);
for (int i = 0; i < list.size(); i++) {
Metric metric = (Metric) list.get(i);
log.info(metric.toStringLong());
}
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:46:19
* AM)
*/
public static void test() {
Metric metric = new Metric(Metric.LOGIN, "BRUCE1");
Metric bruce2 = new Metric(Metric.LOGIN, "BRUCE2");
Metric bruce3 = new Metric(Metric.LOGIN, "BRUCE3");
metric.start();
bruce2.start();
bruce3.start();
try {
Thread.sleep(300);
}
catch (InterruptedException e) {
}
metric.end();
bruce2.pause();
try {
Thread.sleep(300);
}
catch (InterruptedException e) {
}
bruce2.resume();
bruce2.end();
bruce3.end();
Metric bruce4 = new Metric(Metric.LOGIN, "BRUCE4", true);
printGroup(Metric.LOGIN);
bruce4.end();
log.info(bruce4.toStringLong());
bruce4.clear();
bruce4.start();
for (int i = 0; i < 1000000; i++) {
}
bruce4.end();
log.info(bruce4.toStringLong());
// if (isMetricLoggingEnabled())
bruce4.print();
testIsDebugEnabled(bruce4);
}
public static void testSimple() {
Metric metric = new Metric(Metric.LOGIN, "BRUCE1");
metric.start();
try {
Thread.sleep(300);
}
catch (InterruptedException e) {
}
metric.endAndPrint();
}
public static void testIsDebugEnabled(Metric bruce4) {
log.info("");
bruce4.setIsDebugEnabled(true);
log.setLevel(Level.INFO);
log.info("should not print");
bruce4.print();
bruce4.setIsDebugEnabled(false);
log.info("should print");
bruce4.print();
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:38:44
* AM)
*
*@param groupName Description of Parameter
*@return int
*/
public static List getListForGroup(String groupName) {
List list = (List) getAllGroups().get(groupName);
if (list == null) {
list = new ArrayList();
getAllGroups().put(groupName, list);
}
return list;
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:46:19
* AM)
*
*@return The MetricLoggingEnabled value
*/
public static boolean isMetricLoggingEnabled() {
/*
if (isMetricLoggingEnabled == null) {
isMetricLoggingEnabled = new Boolean(com.bc.config.BCConfigImpl.getConfig().getValue("Logging", "metricLogging", "false").equalsIgnoreCase("true"));
}
return isMetricLoggingEnabled.booleanValue();
*/
return true;
}
/**
* Insert the method's description here. Creation date: (12/12/2001
* 10:07:54 AM)
*
*@return java.util.HashMap
*/
private static HashMap getAllGroups() {
if (allGroups == null) {
allGroups = new HashMap();
}
return allGroups;
}
/**
* Insert the method's description here. Creation date: (12/12/2001
* 10:07:54 AM)
*
*@param newAllGroups java.util.HashMap
*/
private static void setAllGroups(HashMap newAllGroups) {
allGroups = newAllGroups;
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:46:19
* AM)
*/
public void clear() {
setStartTime(0);
setEndTime(0);
setPauseElapsedTime(0);
setPauseStartTime(0);
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:46:19
* AM)
*/
public void end() {
setEndTime(System.currentTimeMillis());
}
public void stop() {
end();
}
public void endAndPrint() {
end();
print();
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:49:00
* AM)
*/
public void pause() {
setPauseStartTime(System.currentTimeMillis());
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:46:19
* AM)
*/
public void print() {
if (isMetricLoggingEnabled() ) {
if (isDebugEnabled() && !log.isDebugEnabled())
return;
log.info(toStringLong());
}
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:49:00
* AM)
*/
public void resume() {
if (getPauseStartTime() > 0) {
setPauseElapsedTime(getPauseElapsedTime() + System.currentTimeMillis() - getPauseStartTime());
setPauseStartTime(0);
}
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:46:19
* AM)
*/
public void start() {
clear();
setStartTime(System.currentTimeMillis());
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:38:44
* AM)
*
*@return int
*/
public String toString() {
return toStringBuffer().toString();
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:38:44
* AM)
*
*@return int
*/
public StringBuffer toStringBuffer() {
StringBuffer buffer = new StringBuffer();
String blank = " ";
String elapsedTimeString = "---";
// if (isComplete()) {
elapsedTimeString = Long.toString(getElapsedTime());
// }
buffer.append(getGroupName()).append(blank).append(getMetricName()).append(blank).append(elapsedTimeString);
return buffer;
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:38:44
* AM)
*
*@return int
*/
public String toStringLong() {
StringBuffer buffer = toStringBuffer();
DateFormat dateFormat = JFigUtility.getDateFormat();
String startDate = dateFormat.format(new Date(getStartTime()));
String endDate = dateFormat.format(new Date(getEndTime()));
String blank = " ";
buffer.append(blank).append(startDate).append(blank).append(endDate).append(blank).
append(getPauseElapsedTime()).append(blank);
return buffer.toString();
}
/**
* Insert the method's description here. Creation date: (12/12/2001
* 10:01:21 AM)
*
*@return java.lang.String
*/
public String getGroupName() {
return groupName;
}
/**
* Insert the method's description here. Creation date: (12/12/2001
* 10:01:55 AM)
*
*@return java.lang.String
*/
public String getMetricName() {
return metricName;
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:38:44
* AM)
*
*@return int
*/
public long getElapsedTime() {
return getEndTime() - getStartTime() - getPauseElapsedTime();
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:36:34
* AM)
*
*@return java.lang.Long
*/
protected long getEndTime() {
return endTime;
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:50:37
* AM)
*
*@return long
*/
protected long getPauseElapsedTime() {
return pauseElapsedTime;
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:49:46
* AM)
*
*@return long
*/
protected long getPauseStartTime() {
return pauseStartTime;
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:36:14
* AM)
*
*@return java.lang.Long
*/
protected long getStartTime() {
return startTime;
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:36:34
* AM)
*
*@param newEndTime java.lang.Long
*/
public void setEndTime(long newEndTime) {
endTime = newEndTime;
}
/**
* Insert the method's description here. Creation date: (12/12/2001
* 10:01:21 AM)
*
*@param newGroup java.lang.String
*/
public void setGroupName(String newGroup) {
groupName = newGroup;
}
/**
* Insert the method's description here. Creation date: (12/12/2001
* 10:01:55 AM)
*
*@param newName java.lang.String
*/
public void setMetricName(String newName) {
metricName = newName;
}
public void setMetricNameFailed() {
metricName += "-failed";
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:36:14
* AM)
*
*@param newStartTime java.lang.Long
*/
public void setStartTime(long newStartTime) {
startTime = newStartTime;
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:50:37
* AM)
*
*@param newPauseElapsedTime long
*/
protected void setPauseElapsedTime(long newPauseElapsedTime) {
pauseElapsedTime = newPauseElapsedTime;
}
/**
* Insert the method's description here. Creation date: (12/12/2001 9:49:46
* AM)
*
*@param newPauseStartTime long
*/
protected void setPauseStartTime(long newPauseStartTime) {
pauseStartTime = newPauseStartTime;
}
/**
* Returns the isDebugEnabled.
* @return boolean
*/
protected boolean isDebugEnabled() {
return isDebugEnabled;
}
/**
* Sets the isDebugEnabled.
* @param isDebugEnabled The isDebugEnabled to set
*/
public void setIsDebugEnabled(boolean isDebugEnabled) {
this.isDebugEnabled = isDebugEnabled;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy