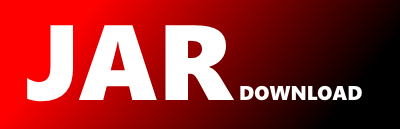
org.igfay.util.PropertyUtility Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jfig Show documentation
Show all versions of jfig Show documentation
A Configuration utility that provides simple, flexible and powerful functionality for managing one or more configurations in a java environment. It uses a combination of a hierarchy of configuration files, substitution variables and property variables.
The newest version!
package org.igfay.util;
import java.net.InetAddress;
import java.net.UnknownHostException;
import java.util.Enumeration;
import java.util.Properties;
import org.apache.log4j.Logger;
/**
* Description of the Class
*
*@author bconrad
*@created March 27, 2001
*/
public class PropertyUtility {
private static Logger log = Logger.getLogger(PropertyUtility.class);
/**
* Constructor for the PropertyUtility object
*/
public PropertyUtility() {
super();
}
/**
* addCommandLineProperties: Add properties from -D flags on the command
* line.
*
*@param args The feature to be added to the CommandLineProperties
* attribute
*/
public static void addCommandLineProperties(String[] args) {
// Loop over command line args.
// Stop on each arg that begins with "-D" and assume it has the format
// 'some-name=some-value'. Parse the name and value tokens,
// and add them to the System.properties object.
log.debug("");
if (args == null) {
log.debug("No properties specified. Return.");
return;
}
String name = null;
String value = null;
for (int ix = 0; ix < args.length; ix++) {
if (args[ix].length() > 2) {
String swChars = args[ix].substring(0, 2);
if (swChars.equalsIgnoreCase("-D")) {
String sw = args[ix].substring(2);
int indexOfEqualSign = sw.indexOf('=');
if (indexOfEqualSign > 0) {
name = sw.substring(0, indexOfEqualSign);
value = sw.substring(indexOfEqualSign + 1);
} else {
name = sw;
value = "";
}
setProperty(name, value);
}
// -D
}
// length > 2
}
// for
processSelectedProperties();
//^^^
}
/**
* addPropertiesFromParameters Add properties from applet tags
*
*@param applet The feature to be added to the PropertiesFromParameters
* attribute
*/
public static void addPropertiesFromParameters(java.applet.Applet applet) {
log.debug("Adding applet parameters");
String parameters[] = {"DEBUG", "VDTROOT", "PARM", "PARMS", "REDIRECT", "SERVLETHOST", "SERVLETPORT", "DBHOST"};
String value = null;
for (int i = 0; i < parameters.length; i++) {
log.debug("i " + i + " Key: " + parameters[i]);
value = applet.getParameter(parameters[i]);
log.debug(" value: " + value);
if (value != null) {
setProperty(parameters[i], value);
log.debug("addPropertiesFromParameters(" + parameters[i] + ", " + value + ")");
}
}
processSelectedProperties();
}
// end getProperty()
/**
* listProperties List System Properties for debugging purposes.
*/
public static void listProperties() {
try {
Properties pr = System.getProperties();
Enumeration enumeration = pr.propertyNames();
String enum_key;
log.info("listProperties(): ----------------------------");
while (enumeration.hasMoreElements()) {
enum_key = (String) enumeration.nextElement();
log.info(
"Key: {" + enum_key + "} Value: {" + pr.getProperty(enum_key) + "}");
}
} catch (Exception e) {
log.warn("*** Exception " + e);
}
}
/**
* Description of the Method
*/
public static void processSelectedProperties() {
}
/**
* propertyEquals
*
*@param propertyName Description of Parameter
*@param value Description of Parameter
*@return Description of the Returned Value
*/
public static boolean propertyEquals(String propertyName, String value) {
try {
String xx = System.getProperty(propertyName.toUpperCase(), value);
if (xx.equalsIgnoreCase(value)) {
return true;
}
} catch (Exception e) {
}
return false;
}
/**
* replaceProperty replace a user passed string to the System Properties
* hashtable.
*
*@param keyWord Description of Parameter
*@param valueString Description of Parameter
*/
public static void replaceProperty(String keyWord, String valueString) {
try {
Properties pr = System.getProperties();
log.debug("Adding keyword: "
+ keyWord
+ " Value: "
+ valueString);
pr.remove(keyWord);
// for replace
pr.put(keyWord, valueString);
} catch (Exception e) {
log.info("*** Exception adding property. keyword: "
+ keyWord
+ " Value: "
+ valueString
+ "\n e "
+ e);
}
}
/**
* getProperty() General utility to return the value of a given property.
* Returns null if not found.
*
*@param propertyName Description of Parameter
*@return The Property value
*/
public static String getProperty(String propertyName) {
try {
String defaultValue = "X$X$X";
String xx = getProperty(propertyName, defaultValue);
if (xx.equals(defaultValue)) {
return null;
}
return xx;
} catch (Exception e) {
log.info("getProperty(S) *** Exception getting property: "
+ propertyName
+ "\n e: "
+ e);
}
return null;
}
// end getProperty()
/**
* getProperty General utility to return the value of a given property.
* Returns default value if not found.
*
*@param propertyName Description of Parameter
*@param defaultValue Description of Parameter
*@return The Property value
*/
public static String getProperty(String propertyName, String defaultValue) {
try {
// First see if this property exists.
String xx = System.getProperty(propertyName);
if (xx != null) {
return xx;
}
// Now see if this property exists in a non case-dependent way
Properties pr = System.getProperties();
Enumeration enumeration = pr.propertyNames();
String enumKey;
while (enumeration.hasMoreElements()) {
enumKey = (String) enumeration.nextElement();
if (enumKey.equalsIgnoreCase(propertyName)) {
return pr.getProperty(enumKey);
}
}
// while
} catch (Exception e) {
log.info("*** Exception getting property. propertyName: "
+ propertyName + " defaultValue: " + defaultValue + "\n e: " + e);
}
return defaultValue;
}
/*
* Utility to get local host name
*
* @return The HostName value
*/
public static String getHostName() {
String HostName;
InetAddress localHost = null;
try {
localHost = InetAddress.getLocalHost();
} catch (UnknownHostException e) {
//error message
}
HostName = new String(localHost.getHostName());
return HostName;
}
public static String getOSString() {
if (getProperty("os.name").toUpperCase().indexOf("WIN") >= 0) {
return "win";
} else if (getProperty("os.name").toUpperCase().indexOf("SUN") >= 0 ||
getProperty("os.name").toUpperCase().indexOf("LIN") >= 0) {
return "unix";
} else {
return "mac";
}
}
public static String getShortHostName() {
String shortHostName = getHostName();
if (shortHostName.indexOf(".") > 0) {
shortHostName = shortHostName.substring(0, shortHostName.indexOf("."));
}
return shortHostName;
}
/**
* Gets the FileSeparator attribute of the Utility class
*
*@return The FileSeparator value
*/
public static String getFileSeparator() {
return getProperty("file.separator");
}
public static boolean isNeedingWindowsFileSeparator(String aDirectory) {
return(isWindows() && !aDirectory.endsWith("/") && !aDirectory.endsWith("\\"));
}
public static boolean isWindows() {
return getProperty("os.name").toUpperCase().indexOf("WIN") >= 0;
}
/**
* Set a user passed string to the System Properties hashtable.
*
*@param keyWord The new Property value
*@param value The new Property value
*/
public static void setProperty(String keyWord, String value) {
Properties pr = System.getProperties();
log.debug("Setting keyword: " + keyWord + " Value: " + value);
if (value == null) {
pr.remove(keyWord);
} else {
pr.put(keyWord, value);
}
}
public static void main(String[] args){
listProperties();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy