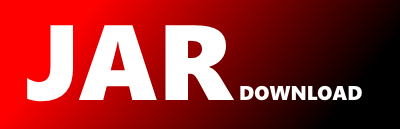
net.sf.jguiraffe.gui.builder.action.SimplePopupMenuHandler Maven / Gradle / Ivy
Show all versions of jguiraffe Show documentation
/*
* Copyright 2006-2010 The JGUIraffe Team.
*
* Licensed under the Apache License, Version 2.0 (the "License")
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.sf.jguiraffe.gui.builder.action;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import net.sf.jguiraffe.gui.builder.components.ComponentBuilderData;
/**
*
* A specialized implementation of a {@link PopupMenuHandler},
* which can be used out of the box for creating not too complex, mostly static
* popup menus.
*
*
* An instance of this class is initialized with a collection defining the
* content of a context menu. This collection can contain the following
* elements:
*
* - Action objects (i.e. objects implementing the
* {@link FormAction} interface): Actions are directly added to the
* context menu constructed by this object.
* - Further
SimplePopupMenuHandler
implementations: If another
* SimplePopupMenuHandler
instance is encountered, a sub menu is
* created, and then the handler is invoked to populate it. In order to provide
* the properties required for a sub menu, this class
* extends {@code ActionDataImpl}. So things like the menu text or its icon can
* be set as properties. There is also a method to initialize all properties
* from another {@link ActionData} object.
* - null elements: They are used for defining menu separators.
*
*
*
* One advantage of this class is that instances can be fully defined in builder
* scripts (using the facilities provided by the dependency injection
* framework). As long as the context menus are static (i.e. they always display
* the same menu items) no programming is required, but this class can be used
* directly and fully defined in the application's configuration scripts.
*
*
* There are some hooks allowing subclasses to influence the menu construction
* process: Specific methods are called before items are added to the menu under
* construction. Derived classes can intercept here and for instance suppress
* actions under certain circumstances.
*
*
* Implementation note: This class is intended to be used by the event handling
* system of the GUI framework, i.e. to be invoked on the event dispatch thread.
* It is not safe to call it on multiple concurrent threads.
*
*
* @author Oliver Heger
* @version $Id: SimplePopupMenuHandler.java 192 2010-08-22 16:15:22Z oheger $
*/
public class SimplePopupMenuHandler extends ActionDataImpl implements
PopupMenuHandler
{
/** A list with the content of the menu. */
private final List> menuItems;
/** Stores the last constructed menu. */
private Object constructedMenu;
/**
* Creates a new instance of SimplePopupMenuHandler
and
* initializes it with the content of the menu. The passed in collection
* must not be null and must contain only valid objects, i.e. (per
* default)
*
* - objects implementing the
{@link FormAction}
interface or
*
* - further
SimplePopupMenuHandler
objects.
*
* The constructor does not check the content of the collection for
* validity. This can be done by invoking the checkMenuItems()
* method by hand.
*
* @param items a collection with the content of the menu to construct
* @throws IllegalArgumentException if the collection is null
* @see #checkMenuItems()
*/
public SimplePopupMenuHandler(Collection> items)
{
if (items == null)
{
throw new IllegalArgumentException(
"Item collection must not be null!");
}
menuItems = new ArrayList