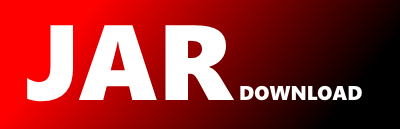
ubc.cs.JLog.Terms.jPredicateTerms Maven / Gradle / Ivy
/*
This file is part of JLog.
Created by Glendon Holst for Alan Mackworth and the
"Computational Intelligence: A Logical Approach" text.
Copyright 1998, 2000, 2002 by University of British Columbia and
Alan Mackworth.
This notice must remain in all files which belong to, or are derived
from JLog.
Check or
for further information
about JLog, or to contact the authors.
JLog is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 2 of the License, or
(at your option) any later version.
JLog is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with JLog; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
URLs: or
*/
//#########################################################################
// PredicateTerms
//#########################################################################
package ubc.cs.JLog.Terms;
import java.lang.*;
import java.util.*;
import ubc.cs.JLog.Foundation.*;
import ubc.cs.JLog.Builtins.*;
/**
* This class represents a collection of predicates.
*
* @author Glendon Holst
* @version %I%, %G%
*/
public class jPredicateTerms extends jCompoundTerm
{
public jPredicateTerms()
{
super();
type = TYPE_PREDICATETERMS;
};
protected jPredicateTerms(Vector t)
{
super(t);
type = TYPE_PREDICATETERMS;
};
public void addTerm(jTerm t)
{
addPredicate((iPredicate) t);
};
public void removeTerm(jTerm t)
{
removePredicate((iPredicate) t);
};
public void addPredicate(iPredicate p)
{
super.addTerm(p);
};
public void removePredicate(iPredicate p)
{
super.removeTerm(p);
};
public jTerm duplicate(jVariable[] vars)
{
return new jPredicateTerms(internal_duplicate(vars));
};
public jTerm copy(jVariableRegistry vars)
{
return new jPredicateTerms(internal_copy(vars));
};
public void addGoals(jGoal g,jVariable[] vars,iGoalStack goals)
{int i;
for (i = terms.size() - 1; i >= 0; i--)
((iPredicate) terms.elementAt(i)).addGoals(g,vars,goals);
};
public void addGoals(jGoal g,iGoalStack goals)
{int i;
for (i = terms.size() - 1; i >= 0; i--)
((iPredicate) terms.elementAt(i)).addGoals(g,goals);
};
protected String getStartingSymbol()
{
return "";
};
protected String getEndingSymbol()
{
return "";
};
/**
* Makes this a representation of the provided jTerm
, which may include
* conjunction and disjunction operators. Invokes makePredicateTerms
.
*
* @param t jTerm
using jCons
to separate
* terms.
*/
public void make(jTerm t)
{
removeAllTerms();
makePredicateTerms(t);
};
/**
* Add jCons
and jOr
separated jTerm
s
* to this jPredicateTerms
.
*
* @param t jTerm
using jCons
and/or
* jOr
to separate terms.
*/
public void makePredicateTerms(jTerm t)
{
if (t instanceof jPredicateTerms)
{jPredicateTerms pterm = (jPredicateTerms) t;
Enumeration e = pterm.enumTerms();
while (e.hasMoreElements())
addPredicate((iPredicate) e.nextElement());
}
else if (t instanceof jOr)
{jOrPredicate jop = new jOrPredicate();
addPredicate(jop);
makeOrPredicateTerms(jop,t);
}
else
makeConsPredicateTerms(t);
};
/**
* Add jOr
separated jTerm
s to the provided
* jOrPredicate
.
*
* @param orpred The jOrPredicate
to add the jOr
* separated jTerm
s to.
* @param term jTerm
using jOr
to separate terms.
*/
protected void makeOrPredicateTerms(jOrPredicate orpred,jTerm term)
{jTerm current = term;
jPredicateTerms pterm;
while (current instanceof jOr)
{jTerm t = ((jOr) current).getLHS();
if (t instanceof jOr)
makeOrPredicateTerms(orpred,t);
else
{
pterm = new jPredicateTerms();
pterm.makeConsPredicateTerms(t);
orpred.addPredicateTerms(pterm);
}
current = ((jOr) current).getRHS();
}
if (current != null)
{
pterm = new jPredicateTerms();
pterm.makeConsPredicateTerms(current);
orpred.addPredicateTerms(pterm);
}
};
/**
* Add jCons
separated jTerm
s to this
* jPredicateTerms
instance.
*
* @param term jTerm
using jOr
to separate terms.
*/
protected void makeConsPredicateTerms(jTerm term)
{
if (term instanceof jCons)
{
makePredicateTerms(((jCons) term).getLHS());
makePredicateTerms(((jCons) term).getRHS());
}
else if (term instanceof iPredicate)
addPredicate((iPredicate) term);
else if (term instanceof jVariable)
addPredicate(new jCall(term));
else if (term != null)
throw new PredicateExpectedException("Expected term to be a predicate or variable.",term);
};
/**
* Creates a jCons
and/or jOr
separated jTerm
s
* to represent this jPredicateTerms
. Invokes
* unmakePredicateTerms
to perform work.
*
* @return jTerm
using jCons
and jOr
* to separate terms. Duplicates this jPredicateTerms
.
*/
public jTerm unmake()
{
return unmakePredicateTerms();
};
/**
* Creates a jCons
and/or jOr
separated jTerm
s
* to represent this jPredicateTerms
.
*
* @return jTerm
using jCons
and jOr
* to separate terms. Duplicates this jPredicateTerms
.
*/
public synchronized jTerm unmakePredicateTerms()
{int i;
jTerm prev = null;
for (i = terms.size() - 1; i >= 0; i--)
{iPredicate curr = (iPredicate) terms.elementAt(i);
jTerm next;
if (curr instanceof iMakeUnmake)
next = ((iMakeUnmake) curr).unmake();
else
next = curr;
if (prev == null)
prev = next;
else
prev = new jCons(next,prev);
}
return prev;
};
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy