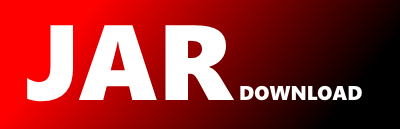
ubc.cs.JLog.Terms.jTerm Maven / Gradle / Ivy
/*
This file is part of JLog.
Created by Glendon Holst for Alan Mackworth and the
"Computational Intelligence: A Logical Approach" text.
Copyright 1998, 2000, 2002 by University of British Columbia and
Alan Mackworth.
This notice must remain in all files which belong to, or are derived
from JLog.
Check or
for further information
about JLog, or to contact the authors.
JLog is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 2 of the License, or
(at your option) any later version.
JLog is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with JLog; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
URLs: or
*/
//#########################################################################
// Term
//#########################################################################
package ubc.cs.JLog.Terms;
import java.lang.*;
import java.util.*;
import ubc.cs.JLog.Foundation.*;
/**
* This is the abstract base class for Prolog terms.
*
* @author Glendon Holst
* @version %I%, %G%
*/
abstract public class jTerm extends jType implements iTerm
{
public jTerm()
{
};
public jTerm getValue()
{
return this;
};
/**
* Returns the non-evaluated jTerm
representation of this term.
* Variables return the non-evaluated terms they are bound to.
*
* @return jTerm
of the non-evaluated term bound to this
* instance.
*/
public jTerm getTerm()
{
return this;
};
public String getName()
{
return "_unnamed_";
};
/**
* The public interface for term comparision, it calls the protected
* compare
that sub-classes must override.
*
* @param term the jTerm
to compare with this instance of
* jTerm
.
* @param var_equal if var_equal
is true
, then unbound
* variables are considered equal.
* var_equal
= false
is standard prolog
* behavior.
* @return LESS_THAN
if this instance is less than
* term
,
* EQUAL
if the two terms are equal, and
* GREATER_THAN
if
* this instance is greater than term
.
*/
public int compare(jTerm term,boolean var_equal)
{
return compare(term,true,var_equal);
};
/**
* The private member function for term comparision. Compares this instance to the
* provided jTerm
. sub-classes must override.
*
* @param term the jTerm
to compare with this instance of
* jTerm
. if
* term
is an unknown type then, if
* first_call
is
* true
, call term.compare(this,false) and return
* the negative result, otherwise return EQUAL
.
* @param first_call true
if just invoked by public
* compare
member function.
* false
otherwise.
* @param var_equal if var_equal
is true
, then unbound
* variables are considered equal.
* var_equal
= false
is standard prolog
* behavior.
* @return LESS_THAN
if this instance is less than
* term
,
* EQUAL
if the two terms are equal, and
* GREATER_THAN
if
* this instance is greater than term
.
*/
abstract protected int compare(jTerm term,boolean first_call,boolean var_equal);
/**
* Call to determine if enclosing rule should record all variable states. A rules
* goal only tracks variables which were unified, and relies on stack unwinding
* to unbind all variables. Terms such as cut
, which jump back to the
* containing rules goal require that all variables are restored.
*
* @return true
if all variable bindings must be recorded by
* the rule containing this term. false
otherwise.
* Normally returns false
*/
public boolean requiresCompleteVariableState()
{
return false;
};
/**
* Adds any unbound variables belonging to this term (or belonging to any sub-part
* of this term) to the jUnifiedVector
*
* @param v The jUnifiedVector
where unbound variables are
* added to. This parameter is used to as output to the caller, not as input.
*/
public void registerUnboundVariables(jUnifiedVector v)
{
};
/**
* Adds all variables belonging to this term (or belonging to any sub-part
* of this term) to the jUnifiedVector
* Should be called during the consultation phase by rules for their owned terms
* both head and base. all variables encountered should be unbound.
* After call, this term should not be modified in any way.
*
* @param v The jUnifiedVector
where variables are
* added to. This parameter is used to as output to the
* caller, not as input.
*/
abstract public void registerVariables(jVariableVector v);
/**
* Adds variables belonging to this term (or belonging to any sub-part
* of this term) to the jVariableVector
*
* @param v The jVariabeVector
where variables are
* added to. This parameter is used to as output to the
* caller, not as input.
* @param all If true
, then all variables should register,
* otherwise only add non-existentially qualified variables.
*/
abstract public void enumerateVariables(jVariableVector v,boolean all);
abstract public boolean equivalence(jTerm term,jEquivalenceMapping v);
abstract public boolean unify(jTerm term,jUnifiedVector v);
/**
* Creates a complete and entirely independant duplicate of this term. User should
* call only for terms for which registerVariables
has already been
* invoked.
* Within duplicate
, any other calls to duplicate should pass along
* the same vars
array produced by registerVariables
* since the same duplication path previously taken by registerVariables
* should be taken by duplicate
. This call is designed only for terms
* which belong to rules and are templates for instantiation. Any variables in the
* term should be unbound. As implied by the modification restrictions on
* registerVariables
, terms and their children cannot change
* (especially during call!)
*
* @param vars The user passes in a duplicate of the variable vector produced
* from the previous call to registerVariables
.
* vars
is produced from the
* jVariableVector
by creating a single duplicate
* variable for each variable. Since this is created in the same
* order as the registerVariables
, it is now
* efficient for jVariables
to return their
* unique duplicate.
* @return jTerm
which is an instantiated duplicate of this
* term.
*/
abstract public jTerm duplicate(jVariable[] vars);
/**
* Public member function which creates a copy of this term. This member function is
* designed to duplicate a term, but without the restrictions of the
* duplicate
function.
* Sub-classes need not override, only implement the abstract
* copy(vars)
member function. copy is not as efficient as
* duplicate
.
*
* @return jTerm
which is an instantiated copy of this
* term.
*/
public jTerm copy()
{jVariableRegistry vars = new jVariableRegistry();
return copy(vars);
};
/**
* Internal member function which creates a copy of this term. This member function
* is designed to duplicate a term, but without the restrictions of the
* duplicate
function. Should only be invoked by copy()
* or other copy(vars)
. Should only invoke other
* copy(vars)
functions.
* Bound variables should return a copy of the bound term.
*
* @param vars The registry of variables and their duplicates. Initially this
* is empty. As variables generate copies, they add themselves
* and their copy to the jVariableRegistry
, and this
* is output from the function call. Any further calls with the
* same vars
ensures that the same variable
* (in a different term) returns the same copy.
* @return jTerm
which is an instantiated copy of this
* term.
*/
abstract public jTerm copy(jVariableRegistry vars);
public void consult(jKnowledgeBase kb)
{
};
public void consultReset()
{
};
public boolean isConsultNeeded()
{
return true;
};
/**
* Produces a string identifying this term, suitable for display to the console.
*
* param usename determines whether to display variables by name or identity.
* false
is the default for displaying the term,
* true
for displaying this term in a user query.
* @return String
which is a textual representation of this
* term.
*/
abstract public String toString(boolean usename);
public String toString()
{
return toString(false);
};
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy