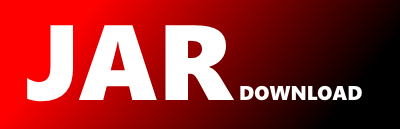
jpathwatch-java.src.name.pachler.nio.file.impl.BSD Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jpathwatch Show documentation
Show all versions of jpathwatch Show documentation
jpathwatch is a Java library for monitoring directories for changes. It
uses the host platform's native OS functions to achive this to avoid
polling.
The newest version!
/*
* Copyright 2008-2011 Uwe Pachler
*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. This particular file is
* subject to the "Classpath" exception as provided in the LICENSE file
* that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
*/
package name.pachler.nio.file.impl;
/**
*
* @author count
*/
public abstract class BSD extends Unix {
public static final short EV_ADD;
public static final short EV_ENABLE;
public static final short EV_DISABLE;
public static final short EV_DELETE;
public static final short EV_ONESHOT;
public static final short EV_CLEAR;
public static final short EV_EOF;
public static final short EV_ERROR;
public static final short EVFILT_VNODE;
public static final short EVFILT_PROC;
// for EVFILT_VNODE
public static final int NOTE_DELETE;
public static final int NOTE_WRITE;
public static final int NOTE_EXTEND;
public static final int NOTE_ATTRIB;
public static final int NOTE_LINK;
public static final int NOTE_RENAME;
public static final int NOTE_REVOKE;
// for EVFILT_PROC
public static final int NOTE_EXIT;
public static final int NOTE_FORK;
public static final int NOTE_EXEC;
public static final int NOTE_TRACK;
public static final int NOTE_TRACKERR;
// for EVFILT_NETDEV
// OSX doesn't have it :(
//public static final int NOTE_LINKUP;
//public static final int NOTE_LINKDOWN;
//public static final int NOTE_LINKINV;
static
{
NativeLibLoader.loadLibrary("jpathwatch-native");
EV_ADD = (short)getIntDefine("EV_ADD");
EV_ENABLE = (short)getIntDefine("EV_ENABLE");
EV_DISABLE = (short)getIntDefine("EV_DISABLE");
EV_DELETE = (short)getIntDefine("EV_DELETE");
EV_ONESHOT = (short)getIntDefine("EV_ONESHOT");
EV_CLEAR = (short)getIntDefine("EV_CLEAR");
EV_EOF = (short)getIntDefine("EV_EOF");
EV_ERROR = (short)getIntDefine("EV_ERROR");
EVFILT_VNODE = (short)getIntDefine("EVFILT_VNODE");
EVFILT_PROC = (short)getIntDefine("EVFILT_PROC");
NOTE_DELETE = getIntDefine("NOTE_DELETE");
NOTE_WRITE = getIntDefine("NOTE_WRITE");
NOTE_EXTEND = getIntDefine("NOTE_EXTEND");
NOTE_ATTRIB = getIntDefine("NOTE_ATTRIB");
NOTE_LINK = getIntDefine("NOTE_LINK");
NOTE_RENAME = getIntDefine("NOTE_RENAME");
NOTE_REVOKE = getIntDefine("NOTE_REVOKE");
// for EVFILT_PROC
NOTE_EXIT = getIntDefine("NOTE_EXIT");
NOTE_FORK = getIntDefine("NOTE_FORK");
NOTE_EXEC = getIntDefine("NOTE_EXEC");
NOTE_TRACK = getIntDefine("NOTE_TRACK");
NOTE_TRACKERR = getIntDefine("NOTE_TRACKERR");
// for EVFILT_NETDEV
// OSX doesn't have it :(
//NOTE_LINKUP = getIntDefine("NOTE_LINKUP");
//NOTE_LINKDOWN = getIntDefine("NOTE_LINKDOWN");
//NOTE_LINKINV = getIntDefine("NOTE_LINKINV");
}
public static native int kqueue();
/**
*
struct kevent {
uintptr_t ident; // identifier for this event
short filter; // filter for event
u_short flags; // action flags for kqueue
u_int fflags; // filter flag value
intptr_t data; // filter data value
void *udata; // opaque user data identifier
};
*/
public static class kevent{
static class IntStringMapping{
int i;
String s;
IntStringMapping(int i, String s){
this.i = i;
this.s = s;
}
}
IntStringMapping [] flagsMapping = {
new IntStringMapping(BSD.EV_ADD, "EV_ADD"),
new IntStringMapping(BSD.EV_ENABLE, "EV_ENABLE"),
new IntStringMapping(BSD.EV_DISABLE, "EV_DISABLE"),
new IntStringMapping(BSD.EV_DELETE, "EV_DELETE"),
new IntStringMapping(BSD.EV_CLEAR, "EV_CLEAR"),
new IntStringMapping(BSD.EV_ONESHOT, "EV_ONESHOT"),
new IntStringMapping(BSD.EV_EOF, "EV_EOF"),
new IntStringMapping(BSD.EV_ERROR, "EV_ERROR"),
};
IntStringMapping [] vnodeNoteMapping = {
new IntStringMapping(BSD.NOTE_DELETE, "NOTE_DELETE"),
new IntStringMapping(BSD.NOTE_WRITE, "NOTE_WRITE"),
new IntStringMapping(BSD.NOTE_EXTEND, "NOTE_EXTEND"),
new IntStringMapping(BSD.NOTE_ATTRIB, "NOTE_ATTRIB"),
new IntStringMapping(BSD.NOTE_LINK, "NOTE_LINK"),
new IntStringMapping(BSD.NOTE_RENAME, "NOTE_RENAME"),
new IntStringMapping(BSD.NOTE_REVOKE, "NOTE_REVOKE"),
};
IntStringMapping [] procNoteMapping = {
new IntStringMapping(BSD.NOTE_EXIT, "NOTE_EXIT"),
new IntStringMapping(BSD.NOTE_FORK, "NOTE_FORK"),
new IntStringMapping(BSD.NOTE_EXEC, "NOTE_EXEC"),
new IntStringMapping(BSD.NOTE_TRACK, "NOTE_TRACK"),
new IntStringMapping(BSD.NOTE_TRACKERR, "NOTE_TRACKERR"),
};
/* IntStringMapping [] netdevNoteMapping = {
new IntStringMapping(BSD.NOTE_LINKUP, "NOTE_LINKUP"),
new IntStringMapping(BSD.NOTE_LINKDOWN, "NOTE_LINKDOWN"),
new IntStringMapping(BSD.NOTE_LINKINV, "NOTE_LINKINV"),
};
*/
private long peer = createPeer();
private static native void initNative();
private static native long createPeer();
private static native void destroyPeer(long peer);
static
{
initNative();
}
@Override
protected void finalize() throws Throwable {
try {
destroyPeer(peer);
peer = 0;
}finally{
super.finalize();
}
}
public native long get_ident();
public native void set_ident(long ident);
public native short get_filter();
public native void set_filter(short filter);
public native short get_flags();
public native void set_flags(short flags);
public native int get_fflags();
public native void set_fflags(int fflags);
public native long get_data();
public native void set_data(long data);
public native Object get_udata();
public native void set_udata(Object udata);
private String bitmaskToString(int value, IntStringMapping[] mapping){
String bitmaskString = "";
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy