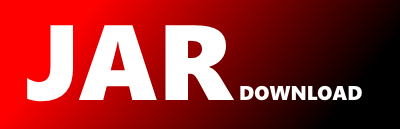
JSci.instruments.PTCroquette Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsci Show documentation
Show all versions of jsci Show documentation
JSci is a set of open source Java packages. The aim is to encapsulate scientific methods/principles in the most natural way possible. As such they should greatly aid the development of scientific based software.
It offers: abstract math interfaces, linear algebra (support for various matrix and vector types), statistics (including probability distributions), wavelets, newtonian mechanics, chart/graph components (AWT and Swing), MathML DOM implementation, ...
Note: some packages, like javax.comm, for the astro and instruments package aren't listed as dependencies (not available).
The newest version!
package JSci.instruments;
import JSci.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.awt.geom.*;
import java.io.*;
import java.text.*;
import javax.swing.*;
import javax.swing.event.*;
import javax.swing.border.*;
/** an Effect that does the particle tracking in 3D, for symmetric images */
public class PTCroquette extends ImageFilterAdapter implements ParticleTracker, ROI {
/** Length of the arms of the cross */
public static int CROSS_L=32;
/** Width of the arms of the cross */
public static int CROSS_H=3;
/** Steps in z for calibration (microns) */
public static double CALIBR_STEP=0.5;
/** While calibrating, averages this number of frames */
public static int CALIBR_AVR=3;
public String getName() { return "PT 3D symmetric"; };
private PTCroquetteCross cross1,cross2;
private PositionControl pc = null;
private ParticleTrackerListener ptl = null;
public void setListener(ParticleTrackerListener ptl) {
this.ptl=ptl;
}
/** @param p the device that will control the motion of the microscope
*/
public PTCroquette(PositionControl p) {
cross1 = new PTCroquetteCross(CROSS_L,CROSS_H,new Point(50,50),Color.MAGENTA);
cross2 = new PTCroquetteCross(CROSS_L,CROSS_H,new Point(50,120),Color.CYAN);
pc = p;
controlComponent = new PTControlComponent( new Control[] {pc,cross1,cross2} );
pc.addChangeListener((ChangeListener)cross1.getControlComponent());
pc.addChangeListener((ChangeListener)cross2.getControlComponent());
controlComponent = new PTControlComponent( new Control[] {pc,cross1,cross2} );
}
/** processes a frame. Do not call this directly.
* @param f the image that must be processed
*/
public void filter(Image f) {
cross1.setBBox(new Rectangle(new Point(0,0),getSize()));
cross2.setBBox(new Rectangle(new Point(0,0),getSize()));
cross1.findXY(f);
cross2.findXY(f);
if (!calibrating) {
cross1.findZ(f);
cross2.findZ(f);
}
else {
cross1.calibrationSendImage(f);
cross2.calibrationSendImage(f);
}
if (ptl!=null)
ptl.receivePosition(f.getTimeStamp(),
new int[] {0,1},
new double[] {cross1.getLocation().getX(),cross2.getLocation().getX()},
new double[] {cross1.getLocation().getY(),cross2.getLocation().getY()},
new double[] {cross1.getZ()-pc.getPosition(),cross2.getZ()-pc.getPosition()}
);
}
/* ------------------------------------------------------- */
/* ROI */
/* ------------------------------------------------------- */
public Shape getShape() { return cross2; }
public void paint(java.awt.Graphics g) {
Graphics2D g2= (Graphics2D)g;
g2.setColor(cross1.getColor());
g2.draw(cross1);
Point2D p = cross1.getLocation();
g2.drawString("Ref",(int)p.getX()+CROSS_H,(int)p.getY()+CROSS_L);
g2.setColor(cross2.getColor());
g2.draw(cross2);
}
private Component mouseComponent;
/** Set the component that displays the image, so that
* the crosses can be moved with the mouse.
* @param c the component from which we want to get the mouse actions
*/
public void setComponent(Component c) {
mouseComponent=c;
c.addMouseListener(new MouseHandler());
c.addMouseMotionListener(new MouseMotionHandler());
c.addMouseWheelListener(new MouseWheelHandler());
}
/* ------------------------------------------------------- */
/* Calibration */
/* ------------------------------------------------------- */
private boolean calibrating = false;
private void doCalibration(int zNum,int lNum,double minZ,double maxZ) {
if (zNum<=0 || minZ>=maxZ) return;
calibrating=true;
cross1.calibrationStart(zNum,minZ,maxZ);
cross2.calibrationStart(zNum,minZ,maxZ);
for (int j=0;j
© 2015 - 2025 Weber Informatics LLC | Privacy Policy