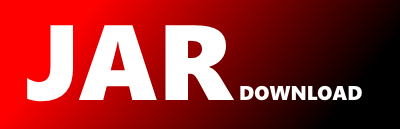
JSci.instruments.RectangularROI Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsci Show documentation
Show all versions of jsci Show documentation
JSci is a set of open source Java packages. The aim is to encapsulate scientific methods/principles in the most natural way possible. As such they should greatly aid the development of scientific based software.
It offers: abstract math interfaces, linear algebra (support for various matrix and vector types), statistics (including probability distributions), wavelets, newtonian mechanics, chart/graph components (AWT and Swing), MathML DOM implementation, ...
Note: some packages, like javax.comm, for the astro and instruments package aren't listed as dependencies (not available).
The newest version!
package JSci.instruments;
import java.awt.*;
import java.awt.event.*;
import java.awt.geom.*;
/** A rectangular Region Of Interest
*/
public class RectangularROI extends ROIAdapter {
private static final int LW = 3;
private int minx,maxx,miny,maxy;
public RectangularROI(int x0,int y0,int x1,int y1) {
minx=x0;
maxx=x1;
miny=y0;
maxy=y1;
}
public Shape getShape() {
return new Rectangle(minx,miny,maxx-minx+1,maxy-miny+1);
}
private Component comp = null;
public void setComponent(Component c) {
if (comp!=null) {
comp.removeMouseListener((MouseListener)this);
comp.removeMouseMotionListener((MouseMotionListener)this);
}
comp=c;
if (comp!=null) {
comp.addMouseListener((MouseListener)this);
comp.addMouseMotionListener((MouseMotionListener)this);
}
}
public void paint(Graphics g) {
g.setColor(Color.GREEN);
((Graphics2D)g).draw(getShape());
}
private int region(Point p) {
if (p.xmaxx || p.ymaxy) return 0;
if (p.y<=miny+LW) {
if (p.x<=minx+LW) return 1;
if (p.xmax) return max;
return x;
}
public void mouseDragged(MouseEvent event) {
int fX,fY,dX,dY;
fX=event.getX();
fY=event.getY();;
dX=fX-iX;
dY=fY-iY;
switch (state) {
case 0:
minx=Math.min(fX,iX);
maxx=Math.max(fX,iX);
miny=Math.min(fY,iY);
maxy=Math.max(fY,iY);
break;
case 1:
minx=clip(0,vminx+dX,maxx);
miny=clip(0,vminy+dY,maxy);
break;
case 2:
miny=clip(0,vminy+dY,maxy);
break;
case 3:
maxx=clip(minx,vmaxx+dX,comp.getWidth()-1);
miny=clip(0,vminy+dY,maxy);
break;
case 4:
minx=clip(0,vminx+dX,maxx);
break;
case 5:
if (vminx+dX<0) dX=-vminx;
if (vmaxx+dX>=comp.getWidth()) dX=comp.getWidth()-vmaxx-1;
if (vminy+dY<0) dY=-vminy;
if (vmaxy+dY>=comp.getHeight()) dY=comp.getHeight()-vmaxy-1;
minx=vminx+dX;
maxx=vmaxx+dX;
miny=vminy+dY;
maxy=vmaxy+dY;
break;
case 6:
maxx=clip(minx,vmaxx+dX,comp.getWidth()-1);
break;
case 7:
minx=clip(0,vminx+dX,maxx);
maxy=clip(miny,vmaxy+dY,comp.getHeight()-1);
break;
case 8:
maxy=clip(miny,vmaxy+dY,comp.getHeight()-1);
break;
case 9:
maxx=clip(minx,vmaxx+dX,comp.getWidth()-1);
maxy=clip(miny,vmaxy+dY,comp.getHeight()-1);
break;
}
comp.repaint();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy