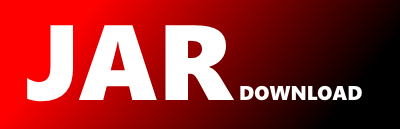
JSci.tests.TestProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsci Show documentation
Show all versions of jsci Show documentation
JSci is a set of open source Java packages. The aim is to encapsulate scientific methods/principles in the most natural way possible. As such they should greatly aid the development of scientific based software.
It offers: abstract math interfaces, linear algebra (support for various matrix and vector types), statistics (including probability distributions), wavelets, newtonian mechanics, chart/graph components (AWT and Swing), MathML DOM implementation, ...
Note: some packages, like javax.comm, for the astro and instruments package aren't listed as dependencies (not available).
The newest version!
package JSci.tests;
import java.util.Iterator;
import java.util.HashMap;
import java.util.Map;
import junit.framework.Test;
import junit.framework.TestResult;
import junitx.extensions.TestSetup;
/**
* A TestSetup which provides additional fixture state in the form of thread-local properties.
* A TestCase can use these properties in its setUp() method to customize its fixture.
*
* public static Test suite() {
* TestSuite suite = new TestSuite();
* Map test1Properties = new HashMap();
* test1Properties.put("test.object", new MyFirstTestObject());
* suite.addTest(new TestProperties(new TestSuite(MyTestCase.class), test1Properties));
*
* Map test2Properties = new HashMap();
* test2Properties.put("test.object", new MySecondTestObject());
* suite.addTest(new TestProperties(new TestSuite(MyTestCase.class), test2Properties));
* return suite;
* }
*
* protected void setUp() throws Exception {
* Map properties = TestProperties.getProperties();
* Object obj = properties.get("test.object");
* // do fixture setup with obj
* }
*
* @author Mark Hale
*/
public class TestProperties extends TestSetup {
/** Thread-local properties. */
private static final ThreadLocal PROPERTIES = new ThreadLocal() {
protected Object initialValue() {
return new HashMap();
}
};
/**
* Returns the properties for this fixture.
*/
public static Map getProperties() {
return (Map) PROPERTIES.get();
}
private final Map properties;
/**
* @param properties the properties to be made available at setUp()
time.
*/
public TestProperties(Test test, Map properties) {
super(test);
this.properties = properties;
}
/**
* Adds this
properties to the fixture properties.
*/
protected void setUp() throws Exception {
getProperties().putAll(properties);
}
/**
* Removes this
properties from the fixture properties.
*/
protected void tearDown() throws Exception {
final Map threadLocalProperties = getProperties();
for(Iterator iter = properties.keySet().iterator(); iter.hasNext(); ) {
threadLocalProperties.remove(iter.next());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy