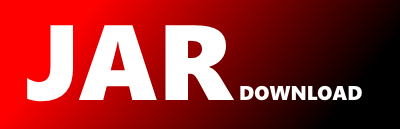
net.sf.jsefa.xml.XmlIOFactoryImpl Maven / Gradle / Ivy
Show all versions of jsefa-android Show documentation
/*
* Copyright 2007 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.sf.jsefa.xml;
import java.util.HashMap;
import java.util.Map;
import net.sf.jsefa.IOFactoryException;
import net.sf.jsefa.common.mapping.TypeMapping;
import net.sf.jsefa.common.validator.Validator;
import net.sf.jsefa.common.validator.traversal.TraversingValidatorFactory;
import net.sf.jsefa.xml.config.XmlConfiguration;
import net.sf.jsefa.xml.lowlevel.XmlLowLevelIOFactory;
import net.sf.jsefa.xml.mapping.ElementDescriptor;
import net.sf.jsefa.xml.mapping.ElementMapping;
import net.sf.jsefa.xml.mapping.ElementMappingsBuilder;
import net.sf.jsefa.xml.mapping.XmlEntryPoint;
import net.sf.jsefa.xml.mapping.XmlTypeMappingUtil;
import net.sf.jsefa.xml.namespace.QName;
/**
* Default implementation of {@link XmlIOFactory}.
*
* Instances of this class are immutable and thread-safe.
*
* @author Norman Lahme-Huetig
*/
public class XmlIOFactoryImpl extends XmlIOFactory {
private final XmlConfiguration config;
private final Map entryElementMappingsByElementDescriptor;
private final Map, ElementMapping> entryElementMappingsByObjectType;
private final XmlLowLevelIOFactory lowLevelIOFactory;
/**
* Creates a new XmlIOFactory
for XmlSerializer
s and
* XmlDeserializer
s using the given configuration.
*
* @param config the configuration object.
* @return a XmlIOFactory
factory
* @throws IOFactoryException
*/
public static XmlIOFactory createFactory(XmlConfiguration config) {
return new XmlIOFactoryImpl(config);
}
XmlIOFactoryImpl(XmlConfiguration config) {
this.config = config;
this.entryElementMappingsByElementDescriptor = createEntryElementMappingsByElementDescriptor();
this.entryElementMappingsByObjectType = createEntryElementMappingsByObjectType();
this.lowLevelIOFactory = XmlLowLevelIOFactory.createFactory(config.getLowLevelConfiguration());
}
/**
* {@inheritDoc}
*/
public XmlSerializer createSerializer() {
return new XmlSerializerImpl(this.config, this.entryElementMappingsByObjectType, this.lowLevelIOFactory
.createSerializer());
}
/**
* {@inheritDoc}
*/
public XmlDeserializer createDeserializer() {
return new XmlDeserializerImpl(this.config, entryElementMappingsByElementDescriptor,
this.lowLevelIOFactory.createDeserializer());
}
private Map createEntryElementMappingsByElementDescriptor() {
ElementMappingsBuilder elementMappingsBuilder = new ElementMappingsBuilder();
TraversingValidatorFactory deepValidatorFactory = new TraversingValidatorFactory(config
.getTypeMappingRegistry(), config.getObjectAccessorProvider());
for (XmlEntryPoint entryPoint : config.getEntryPoints()) {
TypeMapping> typeMapping = config.getTypeMappingRegistry().get(entryPoint.getDataTypeName());
if (typeMapping == null) {
throw new IOFactoryException("Unknown data type: " + entryPoint.getDataTypeName());
}
ElementDescriptor elementDescriptor = new ElementDescriptor(entryPoint.getDesignator(), entryPoint
.getDataTypeName());
Validator validator = deepValidatorFactory.create(entryPoint.getDataTypeName(), entryPoint
.getValidator());
elementMappingsBuilder.addMapping(entryPoint.getDataTypeName(), elementDescriptor, typeMapping
.getObjectType(), null, validator, null);
}
return XmlTypeMappingUtil.createNodeMappingsByNodeDescriptorMap(elementMappingsBuilder.getResult());
}
private Map, ElementMapping> createEntryElementMappingsByObjectType() {
Map, ElementMapping> elementMappings = new HashMap, ElementMapping>();
for (ElementMapping elementMapping : this.entryElementMappingsByElementDescriptor.values()) {
elementMappings.put(elementMapping.getObjectType(), elementMapping);
}
return elementMappings;
}
}