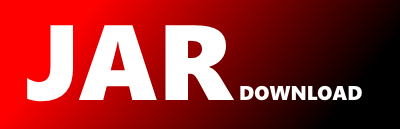
jtopenlite.com.ibm.jtopenlite.database.jdbc.JDBCClob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jt400 Show documentation
Show all versions of jt400 Show documentation
The Open Source version of the IBM Toolbox for Java
package com.ibm.jtopenlite.database.jdbc;
import com.ibm.jtopenlite.Conv;
import java.io.*;
import java.sql.*;
public class JDBCClob implements Clob
{
private final byte[] data_;
private final int offset_;
private int length_;
private final int ccsid_;
public JDBCClob(byte[] data, int offset, int len, int ccsid)
{
data_ = data;
offset_ = offset;
length_ = len;
ccsid_ = ccsid;
}
private static final class JDBCClobOutputStream extends OutputStream
{
private final JDBCClob clob_;
private int next_;
JDBCClobOutputStream(JDBCClob clob, int start) // 0-based.
{
clob_ = clob;
next_ = start+clob_.offset_;
}
public void write(int b) throws IOException
{
if (next_ < clob_.offset_+clob_.length_)
{
clob_.data_[next_++] = (byte)b;
}
}
};
private static final class JDBCClobWriter extends Writer
{
private final JDBCClob clob_;
private int next_;
JDBCClobWriter(JDBCClob clob, int start) // 0-based.
{
clob_ = clob;
next_ = start+clob_.offset_;
}
public void close()
{
}
public void flush()
{
}
public void write(char[] buf, int off, int len) throws IOException
{
for (int i=off; i length) total = length;
byte[] data = new byte[total];
System.arraycopy(data_, offset_+ipos-1, data, 0, total);
try
{
return Conv.ebcdicByteArrayToString(data_, offset_+ipos-1, total, ccsid_);
}
catch (UnsupportedEncodingException uee)
{
SQLException sql = new SQLException(uee.toString());
sql.initCause(uee);
throw sql;
}
}
public long length() throws SQLException
{
return length_;
}
public long position(Clob pattern, long start) throws SQLException
{
if (start <= 0) throw new SQLException("Bad start: "+start);
String patternString = pattern.getSubString(0, (int)(pattern.length() & 0x7FFFFFFF));
return position(patternString, start);
}
public long position(String patternString, long start) throws SQLException
{
if (start <= 0) throw new SQLException("Bad start: "+start);
try
{
final byte[] pattern = Conv.stringToEBCDICByteArray(patternString, ccsid_);
for (int i=(int)(start & 0x7FFFFFFF)+offset_-1; i len) total = len;
try
{
final byte[] bytes = Conv.stringToEBCDICByteArray(str, ccsid_);
System.arraycopy(bytes, offset, data_, offset_+ipos-1, total);
return total;
}
catch (UnsupportedEncodingException uee)
{
SQLException sql = new SQLException(uee.toString());
sql.initCause(uee);
throw sql;
}
}
public void truncate(long len) throws SQLException
{
length_ = (len < 0) ? 0 : (int)(len & 0x7FFFFFFF);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy