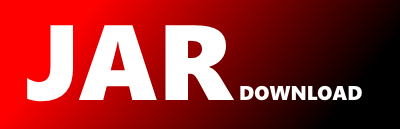
src.com.ibm.as400.util.html.SelectFormElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jt400 Show documentation
Show all versions of jt400 Show documentation
The Open Source version of the IBM Toolbox for Java
///////////////////////////////////////////////////////////////////////////////
//
// JTOpen (IBM Toolbox for Java - OSS version)
//
// Filename: SelectFormElement.java
//
// The source code contained herein is licensed under the IBM Public License
// Version 1.0, which has been approved by the Open Source Initiative.
// Copyright (C) 1997-2001 International Business Machines Corporation and
// others. All rights reserved.
//
///////////////////////////////////////////////////////////////////////////////
package com.ibm.as400.util.html;
import com.ibm.as400.access.Trace;
import com.ibm.as400.access.ExtendedIllegalStateException;
import com.ibm.as400.access.ExtendedIllegalArgumentException;
import java.util.*;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.beans.VetoableChangeSupport;
import java.beans.VetoableChangeListener;
import java.beans.PropertyVetoException;
/**
* The SelectFormElement class represents a select input type in an HTML form.
* The trailing slash "/" on the SelectFormElement tag allows it to
* conform to the XHTML specification.
*
*
* This example creates a SelectFormElement object with three options and prints out the HTML tag.
* The first two options added specify the option text, name, and select attributes. The third
* option added is defined by a SelectOption object.
*
*
* SelectFormElement list = new SelectFormElement("list1");
* SelectOption option1 = list.addOption("Option1", "opt1");
* SelectOption option2 = list.addOption("Option2", "opt2", false);
* SelectOption option3 = new SelectOption("Option3", "opt3", true);
* list.addOption(option3);
* System.out.println(list.getTag());
*
*
* Here is the output of the SelectFormElement tag:
*
* <select name="list1">
* <option value="opt1">Option1</option>
* <option value="opt2">Option2</option>
* <option value="opt3" selected="selected">Option3</option>
* </select>
*
*
* SelectFormElement objects generate the following events:
*
* - ElementEvent - The events fired are:
*
* - elementAdded
*
- elementRemoved
*
* - PropertyChangeEvent
*
- VetoableChangeEvent
*
*
* @see com.ibm.as400.util.html.SelectOption
**/
public class SelectFormElement extends HTMLTagAttributes implements java.io.Serializable // @Z1C
{
private static final String copyright = "Copyright (C) 1997-2001 International Business Machines Corporation and others.";
static final long serialVersionUID = -5409799783351050576L;
private String name_; // The select element name.
private int size_; // The number of visible choices.
private boolean multiple_; // Whether multiple selections can be made.
private boolean optionSelected_;// Whether at least one option is marked as selected.
private String lang_; // The primary language used to display the tags contents. //$B1A
private String dir_; // The direction of the text interpretation. //$B1A
private Vector list_; // List of options.
transient private VetoableChangeSupport vetos_; //@CRS
transient private Vector elementListeners; // The list of element listeners @CRS
/**
* Constructs a default SelectFormElement object.
**/
public SelectFormElement()
{
super();
size_ = 0;
multiple_ = false;
list_ = new Vector();
}
/**
* Constructs a SelectFormElement with the specified control name.
* @param name The control name of the select element.
**/
public SelectFormElement(String name)
{
this();
try
{
setName(name);
}
catch (PropertyVetoException e)
{
}
}
/**
Adds an addElementListener.
The specified addElementListeners elementAdded method will
be called each time a radioforminput is added to the group.
The addElementListener object is added to a list of addElementListeners
managed by this RadioFormInputGroup. It can be removed with removeElementListener.
@see #removeElementListener
@param listener The ElementListener.
**/
public void addElementListener(ElementListener listener)
{
if (listener == null)
throw new NullPointerException ("listener");
if (elementListeners == null) elementListeners = new Vector(); //@CRS
elementListeners.addElement(listener);
}
/**
* Adds an option to the select form element.
* @param option The select option.
**/
public void addOption(SelectOption option)
{
//@C1D
if (option == null)
throw new NullPointerException("option");
if ((option.isSelected()) && (optionSelected_) && !(multiple_))
{
Trace.log(Trace.ERROR, "Multiple options marked as 'selected' but multiple attribute not set.");
throw new ExtendedIllegalArgumentException("selected", ExtendedIllegalArgumentException.PARAMETER_VALUE_NOT_VALID);
}
else if ((option.isSelected()) && !(optionSelected_))
optionSelected_ = true;
list_.addElement(option);
fireElementEvent(ElementEvent.ELEMENT_ADDED);
}
/**
* Adds an option with the specified viewable text and initial input
* value to the select form element.
* @param text The viewable option text.
* @param value The option input value.
*
* @return A SelectOption object.
**/
public SelectOption addOption(String text, String value)
{
return addOption(text, value, false);
}
/**
* Adds an option with the specified viewable text, initial input value,
* and initial selected value to the select form element. Only one option can be
* selected in the select form element at a time.
* @param text The viewable option text.
* @param value The option input value.
* @param selected true if the option defaults as being selected; false otherwise.
*
* @return A SelectOption object.
**/
public SelectOption addOption(String text, String value, boolean selected)
{
//@C1D
if (text == null)
throw new NullPointerException("text");
if (value == null)
throw new NullPointerException("value");
if ((selected) && (optionSelected_) && !(multiple_))
{
Trace.log(Trace.ERROR, "Multiple options marked as 'selected' but multiple attribute not set.");
throw new ExtendedIllegalArgumentException("selected", ExtendedIllegalArgumentException.PARAMETER_VALUE_NOT_VALID);
}
else if ((selected) && !(optionSelected_))
optionSelected_ = true;
SelectOption option = new SelectOption(text, value, selected);
list_.addElement(option);
fireElementEvent(ElementEvent.ELEMENT_ADDED);
return option;
}
/**
Adds the VetoableChangeListener. The specified VetoableChangeListener's
vetoableChange method will be called each time the value of any
constrained property is changed.
@see #removeVetoableChangeListener
@param listener The VetoableChangeListener.
**/
public void addVetoableChangeListener(VetoableChangeListener listener)
{
if (listener == null)
throw new NullPointerException ("listener");
if (vetos_ == null) vetos_ = new VetoableChangeSupport(this); //@CRS
vetos_.addVetoableChangeListener(listener);
}
/**
* Fires the element event.
**/
private void fireElementEvent(int evt)
{
if (elementListeners == null) return; //@CRS
Vector targets;
targets = (Vector) elementListeners.clone();
ElementEvent elementEvt = new ElementEvent(this, evt);
for (int i = 0; i < targets.size(); i++)
{
ElementListener target = (ElementListener)targets.elementAt(i);
if (evt == ElementEvent.ELEMENT_ADDED)
target.elementAdded(elementEvt);
else if (evt == ElementEvent.ELEMENT_REMOVED)
target.elementRemoved(elementEvt);
}
}
/**
* Returns the direction of the text interpretation.
* @return The direction of the text.
**/
public String getDirection() //$B1A
{
return dir_;
}
/**
* Returns the language of the input element.
* @return The language of the input element.
**/
public String getLanguage() //$B1A
{
return lang_;
}
/**
* Returns the control name of the select element.
* @return The control name.
**/
public String getName()
{
return name_;
}
/**
* Returns the number of elements in the option layout.
* @return The number of elements.
**/
public int getOptionCount()
{
return list_.size();
}
/**
* Returns the number of visible options.
* @return The number of options.
**/
public int getSize()
{
return size_;
}
/**
* Returns a comment tag.
* This method should not be called. There is no XSL-FO support for this class.
* @return The comment tag.
**/
public String getFOTag() //@D1A
{
Trace.log(Trace.ERROR, "Attempting to getFOTag() for an object that doesn't support it.");
return "";
}
/**
* Returns the select form element tag.
* @return The tag.
**/
public String getTag()
{
//@C1D
if (name_ == null)
{
Trace.log(Trace.ERROR, "Attempting to get tag before setting name.");
throw new ExtendedIllegalStateException(
"name", ExtendedIllegalStateException.PROPERTY_NOT_SET );
}
StringBuffer s = new StringBuffer("
© 2015 - 2025 Weber Informatics LLC | Privacy Policy