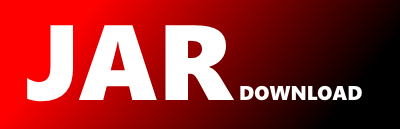
com.ibm.as400.util.html.HTMLForm Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jt400 Show documentation
Show all versions of jt400 Show documentation
The Open Source version of the IBM Toolbox for Java
///////////////////////////////////////////////////////////////////////////////
//
// JTOpen (IBM Toolbox for Java - OSS version)
//
// Filename: HTMLForm.java
//
// The source code contained herein is licensed under the IBM Public License
// Version 1.0, which has been approved by the Open Source Initiative.
// Copyright (C) 1997-2001 International Business Machines Corporation and
// others. All rights reserved.
//
///////////////////////////////////////////////////////////////////////////////
package com.ibm.as400.util.html;
import com.ibm.as400.access.Trace;
import com.ibm.as400.access.ExtendedIllegalStateException;
import com.ibm.as400.access.ExtendedIllegalArgumentException;
import java.util.Vector;
import java.util.Enumeration;
import java.util.Properties;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.beans.VetoableChangeSupport;
import java.beans.VetoableChangeListener;
import java.beans.PropertyVetoException;
/**
* The HTMLForm class represents an HTML form.
*
* HTMLForm objects generate the following events:
*
* - ElementEvent - The events fired are:
*
* - elementAdded
*
- elementRemoved
*
* - PropertyChangeEvent
*
- VetoableChangeEvent
*
*
* This examples creates an HTMLForm object and adds some form input types to it.
*
* // Create a text input form element for the system.
* LabelFormElement sysPrompt = new LabelFormElement("System:");
* TextFormInput system = new TextFormInput("System");
*
* // Create a text input form element for the userId.
* LabelFormElement userPrompt = new LabelFormElement("User:");
* TextFormInput user = new TextFormInput("User");
*
* // Create a password input form element for the password.
* LabelFormElement passwordPrompt = new LabelFormElement("Password:");
* PasswordFormInput password = new PasswordFormInput("Password");
*
* // Create a properties object.
* Properties prop = new Properties();
*
* // Add customer name and ID values to the properties object.
* prop.put("custName", "Mr. Toolbox");
* prop.put("custID", "12345");
*
* // Create the submit button to the form.
* SubmitFormInput logonButton = new SubmitFormInput("logon", "Logon");
*
* // Create HTMLForm object and add the panel to it.
* HTMLForm form = new HTMLForm(servletURI);
* form.setHiddenParameterList(prop);
* form.addElement(sysPrompt);
* form.addElement(system);
* form.addElement(userPrompt);
* form.addElement(user);
* form.addElement(passwordPrompt);
* form.addElement(password);
* form.addElement(logonButton);
*
*
* Here is an example of an HTMLForm tag:
*
* <form action="servletURI" method="get">
* System: <input type="text" name="System" />
* User: <input type="text" name="User" />
* Password: <input type="password" name="Password" />
* <input type="submit" name="logon" value="Logon" />
* <input type="hidden" name="custName" value="Mr. Toolbox" />
* <input type="hidden" name="custID" value="12345" />
* </form>
*
**/
public class HTMLForm extends HTMLTagAttributes implements HTMLConstants, java.io.Serializable // @Z1C
{
private static final String copyright = "Copyright (C) 1997-2001 International Business Machines Corporation and others.";
static final long serialVersionUID = -7610016051219431008L;
/**
HTTP GET Method for sending form contents to the server.
This is the default method used.
**/
public static final int METHOD_GET = 0;
/**
HTTP POST Method for sending form contents to the server.
**/
public static final int METHOD_POST = 1;
private Vector list_; // The list of FormElements.
private String url_; // The ACTION url address.
private String target_; // The target frame for the link resource.
private Properties parms_; // The hidden parameter list.
private boolean useGet_ = true; //
private int method_ = METHOD_GET; // The HTTP method used.
private String lang_; // The primary language used to display the tags contents. //$B1A
private String dir_; // The direction of the text interpretation. //$B1A
transient private VetoableChangeSupport vetos_; //@CRS
transient private Vector elementListeners; // The list of element listeners @CRS
/**
* Constructs a default HTMLForm object.
**/
public HTMLForm()
{
list_ = new Vector();
}
/**
* Constructs an HTMLForm object with the specified URL.
* @param url The URL address.
**/
public HTMLForm(String url)
{
this();
try
{
setURL(url);
}
catch (PropertyVetoException e)
{
}
}
/**
* Adds a form element to the HTMLForm.
* @param element The form element.
**/
public void addElement(HTMLTagElement element)
{
//@C1D
if (element == null)
throw new NullPointerException("element");
list_.addElement(element);
fireElementEvent(ElementEvent.ELEMENT_ADDED);
}
/**
* Adds an ElementListener.
* The ElementListener object is added to an internal list of ElementListeners;
* it can be removed with removeElementListener.
* @see #removeElementListener
* @param listener The ElementListener.
**/
public void addElementListener(ElementListener listener)
{
if (listener == null)
throw new NullPointerException("listener");
if (elementListeners == null) elementListeners = new Vector(); //@CRS
elementListeners.addElement(listener);
}
/**
Adds the VetoableChangeListener. The specified
VetoableChangeListener's vetoableChange
method is called each time the value of any
constrained property is changed.
@see #removeVetoableChangeListener
@param listener The VetoableChangeListener.
**/
public void addVetoableChangeListener(VetoableChangeListener listener)
{
if (listener == null)
throw new NullPointerException ("listener");
if (vetos_ == null) vetos_ = new VetoableChangeSupport(this); //@CRS
vetos_.addVetoableChangeListener(listener);
}
/**
* Fires the element event.
**/
private void fireElementEvent(int evt)
{
if (elementListeners == null) return; //@CRS
Vector targets;
targets = (Vector) elementListeners.clone();
ElementEvent elementEvt = new ElementEvent(this, evt);
for (int i = 0; i < targets.size(); i++)
{
ElementListener target = (ElementListener)targets.elementAt(i);
if (evt == ElementEvent.ELEMENT_ADDED)
target.elementAdded(elementEvt);
else if (evt == ElementEvent.ELEMENT_REMOVED)
target.elementRemoved(elementEvt);
}
}
/**
* Returns the direction of the text interpretation.
* @return The direction of the text.
**/
public String getDirection() //$B1A
{
return dir_;
}
/**
* Returns the form's hidden parameter list.
* @return The parameter list.
**/
public Properties getHiddenParameterList()
{
return parms_;
}
/**
* Returns the language of the input element.
* @return The language of the input element.
**/
public String getLanguage() //$B1A
{
return lang_;
}
/**
* Returns the HTTP method used for sending form contents to the server.
* @return The HTTP method.
**/
public int getMethod()
{
return method_;
}
/**
* Returns a comment tag.
* This method should not be called. There is no XSL-FO support for this class.
* @return The comment tag.
**/
public String getFOTag() //@D1A
{
Trace.log(Trace.ERROR, "Attempting to getFOTag() for an object that doesn't support it.");
return "";
}
/**
* Returns the HTML form tag.
* @return The tag.
**/
public String getTag()
{
//@C1D
if (url_ == null)
{
Trace.log(Trace.ERROR, "Attempting to get tag before setting the action URL address.");
throw new ExtendedIllegalStateException(
"url", ExtendedIllegalStateException.PROPERTY_NOT_SET );
}
StringBuffer s = new StringBuffer("
© 2015 - 2025 Weber Informatics LLC | Privacy Policy