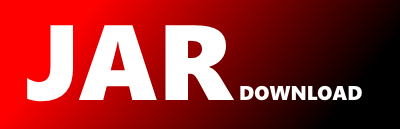
com.ibm.as400.util.html.HTMLTreeElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jt400 Show documentation
Show all versions of jt400 Show documentation
The Open Source version of the IBM Toolbox for Java
///////////////////////////////////////////////////////////////////////////////
//
// JTOpen (IBM Toolbox for Java - OSS version)
//
// Filename: HTMLTreeElement.java
//
// The source code contained herein is licensed under the IBM Public License
// Version 1.0, which has been approved by the Open Source Initiative.
// Copyright (C) 1997-2002 International Business Machines Corporation and
// others. All rights reserved.
//
///////////////////////////////////////////////////////////////////////////////
package com.ibm.as400.util.html;
import java.util.Vector;
import java.util.Properties;
import java.text.Collator;
import java.beans.PropertyChangeSupport;
import java.beans.PropertyChangeListener;
import java.beans.PropertyVetoException;
import com.ibm.as400.access.Trace;
import com.ibm.as400.access.ExtendedIllegalStateException;
import com.ibm.as400.util.servlet.ServletHyperlink;
/**
* The HTMLTreeElement class represents an hierarchial element within an HTMLTree or other
* HTMLTreeElements.
*
* This example creates an HTMLTreeElement object.
*
*
*
* // Create parent HTMLTreeElement.
* HTMLTreeElement parentElement = new HTMLTreeElement();
* parentElement.setTextUrl(new HTMLHyperlink("http://myWebPage", "My Web Page"));
*
* // Create HTMLTreeElement Child.
* HTMLTreeElement childElement = new HTMLTreeElement();
* childElement.setTextUrl(new HTMLHyperlink("http://anotherWebPage", "Another Web Page"));
* parentElement.addElement(childElement);
*
*
*
*
* Once the elements are added to an HTMLTree object and the elements are expanded, the
* HTMLTreeElements will look like this:
*
*
*
*
*
* -
*
*
* My Web Page
*
*
*
*
*
*
* -
*
*
* Another Web Page
*
*
*
*
*
*
* HTMLTreeElement objects generate the following events:
*
* - ElementEvent - The events fired are:
*
* - elementAdded
*
- elementRemoved
*
* - PropertyChangeEvent
*
*
* @see com.ibm.as400.util.html.HTMLTree
* @see com.ibm.as400.util.html.URLParser
**/
public class HTMLTreeElement implements HTMLTagElement, java.io.Serializable
{
private static final String copyright = "Copyright (C) 1997-2002 International Business Machines Corporation and others.";
static final long serialVersionUID = -2067619739727758210L;
private HTMLVector branches_; //@P2C
private boolean expanded_ = false;
private HTMLHyperlink textUrl_ = null;
private HTMLHyperlink iconUrl_ = null;
private HTMLTagElement elementData_ = null;
private boolean sort_ = true; // @A1A
transient private Collator collator_;
private static String expandedGif_ = null;
private static String collapsedGif_ = null;
private static String docGif_ = null;
private static final String std = "\n"; // The start table definition tag. // @B2C
private static final String etd = " \n"; // The end table definition tag. // @B2C
transient PropertyChangeSupport changes_; //@P2C
transient private Vector elementListeners_; // The list of element listeners @P2C
/**
* Constructs a default HTMLTreeElement object.
**/
public HTMLTreeElement()
{
// @B2A
// If the locale is Korean, then this throws
// an ArrayIndexOutOfBoundsException. This is
// a bug in the JDK. The workarond in that case
// is just to use String.compareTo().
try // @B2A
{
collator_ = Collator.getInstance (); // @B2A
collator_.setStrength (Collator.PRIMARY); // @B2A
}
catch (Exception e) // @B2A
{
collator_ = null; // @B2A
}
branches_ = new HTMLVector(); //@P2C
}
/**
* Constructs an HTMLTreeElement with the specified text.
*
* @param text The text.
**/
public HTMLTreeElement(String text)
{
this();
setText(text);
}
/**
* Constructs an HTMLTreeElement with the specified text.
*
* @param text The text.
**/
public HTMLTreeElement(HTMLTagElement text)
{
this();
setText(text);
}
/**
* Constructs an HTMLTreeElement with the specified textUrl.
*
* @param textUrl The HTMLHyperlink.
**/
public HTMLTreeElement(HTMLHyperlink textUrl)
{
this();
setTextUrl(textUrl);
}
/**
* Adds a child element to the parent HTMLTreeElement
*
* @param element The HTMLTreeElement.
**/
public void addElement(HTMLTreeElement element)
{
if (element == null)
throw new NullPointerException("element");
branches_.addElement(element);
if (elementListeners_ != null) fireElementEvent(ElementEvent.ELEMENT_ADDED); //@P2C
}
/**
* Adds an addElementListener.
* The specified addElementListeners elementAdded method will
* be called each time a HTMLTreeElement is added.
* The addElementListener object is added to a list of addElementListeners
* managed by this HTMLTreeElement. It can be removed with removeElementListener.
*
* @see #removeElementListener
*
* @param listener The ElementListener.
**/
public void addElementListener(ElementListener listener)
{
if (listener == null)
throw new NullPointerException ("listener");
if (elementListeners_ == null) elementListeners_ = new Vector(); //@P2A
elementListeners_.addElement(listener);
}
/**
* Adds a PropertyChangeListener. The specified
* PropertyChangeListener's propertyChange
* method is called each time the value of any
* bound property is changed.
*
* @see #removePropertyChangeListener
* @param listener The PropertyChangeListener.
**/
public void addPropertyChangeListener(PropertyChangeListener listener)
{
if (listener == null)
throw new NullPointerException("listener");
if (changes_ == null) changes_ = new PropertyChangeSupport(this); //@P2A
changes_.addPropertyChangeListener(listener);
}
/**
* Fires the element event.
**/
private void fireElementEvent(int evt)
{
Vector targets;
targets = (Vector) elementListeners_.clone();
ElementEvent elementEvt = new ElementEvent(this, evt);
for (int i = 0; i < targets.size(); i++)
{
ElementListener target = (ElementListener)targets.elementAt(i);
if (evt == ElementEvent.ELEMENT_ADDED)
target.elementAdded(elementEvt);
else if (evt == ElementEvent.ELEMENT_REMOVED)
target.elementRemoved(elementEvt);
}
}
/**
* Returns the collapsed gif.
*
* @return The collapsed gif.
**/
public String getCollapsedGif()
{
return collapsedGif_;
}
/**
* Returns the document gif.
*
* @return The document gif.
**/
public String getDocGif()
{
return docGif_;
}
/**
* Returns the expanded gif.
*
* @return The expanded gif.
**/
public String getExpandedGif()
{
return expandedGif_;
}
/**
* Returns the icon URL.
*
* @return The icon URL.
**/
public HTMLHyperlink getIconUrl()
{
return iconUrl_;
}
/**
* Returns the visible text of the HTMLTreeElement.
*
* @return text The text.
**/
public HTMLTagElement getText()
{
return elementData_;
}
/**
* Returns a comment tag.
* This method should not be called. There is no XSL-FO support for this class.
* @return The comment tag.
**/
public String getFOTag() //@C1A
{
Trace.log(Trace.ERROR, "Attempting to getFOTag() for an object that doesn't support it.");
return "";
}
/**
* Returns the HTMLTreeElement tag.
*
* @return The tag.
**/
public String getTag()
{
if (iconUrl_ != null)
setIconUrl(iconUrl_);
else
throw new ExtendedIllegalStateException("iconUrl", ExtendedIllegalStateException.PROPERTY_NOT_SET);
if (elementData_ == null)
throw new ExtendedIllegalStateException("text", ExtendedIllegalStateException.PROPERTY_NOT_SET );
StringBuffer buf = new StringBuffer(); // @B2C
buf.append("\n");
if (isLeaf())
{
if (Trace.isTraceOn())
Trace.log(Trace.INFORMATION, " Element is a leaf.");
buf.append(std);
if (docGif_ != null)
{
buf.append("
\n"); // @B3C
}
else
buf.append(">");
buf.append(etd);
buf.append(std);
buf.append(elementData_.getTag()); // @B2C
buf.append("\n"); // @B2C
buf.append(etd);
buf.append(" \n");
}
else
{
String hcStr = com.ibm.as400.util.html.URLEncoder.encode(Integer.toString(this.hashCode()));
buf.append(std);
StringBuffer iconTag = new StringBuffer();
if (isExpanded())
{
if (expandedGif_ != null)
{ // @B2C
iconTag.append("
"); // @B3C
}
else // @B2C
iconTag.append("-");
}
else
{
if (collapsedGif_ != null)
{
iconTag.append( "
"); // @B3C
}
else
iconTag.append("+");
}
if (iconUrl_ != null)
{
try
{
iconUrl_.setName(hcStr);
Properties iconProp = iconUrl_.getProperties();
if (iconProp == null)
iconProp = new Properties();
iconProp.put("hashcode", hcStr); // @B1C
if (expanded_)
iconProp.put("action", "contract"); // @B1C
else
iconProp.put("action", "expand"); // @B1C
iconUrl_.setProperties(iconProp);
URLParser parser = new URLParser(iconUrl_.getLink());
iconUrl_.setLink(parser.getURI()); //$A3C
iconUrl_.setText(iconTag.toString());
iconUrl_.setLocation(hcStr); //$A3A
}
catch (PropertyVetoException e)
{ /* Ignore */
}
buf.append(iconUrl_.getTag());
}
else
{
buf.append(iconTag);
}
buf.append("\n");
buf.append(etd);
buf.append(std);
// If the text URL has been set
if (textUrl_ != null)
{
try
{
if (textUrl_.getText() == null)
textUrl_.setText(elementData_.getTag() + "\n");
}
catch (PropertyVetoException e)
{ /* Ignore */
}
buf.append(textUrl_.getTag());
}
else // If the text URL has NOT been set.
{
buf.append(elementData_.getTag()); // @B2C
buf.append("\n"); // @B2C
}
buf.append(etd); // @B2C
buf.append("\n");
if (isExpanded())
{
buf.append(" \n\n"); //@P2C
if (sort_) HTMLTree.sort(collator_, branches_); // @A1A // @B2C @P2C
int size = branches_.getCount(); //@P2A
Object[] data = branches_.getData(); //@P2A
for (int i=0; i\n\n"); //@P2C
//@P2D buf.append("\n");
}
}
return buf.toString();
}
/**
* Returns the text URL.
*
* @return The text URL.
**/
public HTMLHyperlink getTextUrl()
{
return textUrl_;
}
/**
* Indicates if the HTMLTreeElement is expanded.
*
* @return true if expanded, false otherwise.
**/
public boolean isExpanded()
{
return expanded_;
}
/**
* Indicates if the HTMLTreeElement is a leaf.
*
* @return true if the element is a leaf, false otherwise.
**/
public boolean isLeaf()
{
return branches_.isEmpty();
}
/**
* Deserializes and initializes transient data.
**/
private void readObject(java.io.ObjectInputStream in)
throws java.io.IOException, ClassNotFoundException
{
// @B2A
// If the locale is Korean, then this throws
// an ArrayIndexOutOfBoundsException. This is
// a bug in the JDK. The workarond in that case
// is just to use String.compareTo().
try // @B2A
{
collator_ = Collator.getInstance (); // @B2A
collator_.setStrength (Collator.PRIMARY); // @B2A
}
catch (Exception e) // @B2A
{
collator_ = null; // @B2A
}
in.defaultReadObject();
//@P2D changes_ = new PropertyChangeSupport(this);
//@P2D elementListeners_ = new Vector();
}
/**
* Removes a child element from the parent HTMLTreeElement
*
* @param element The HTMLTreeElement.
**/
public void removeElement(HTMLTreeElement element)
{
if (element == null)
throw new NullPointerException("element");
if (branches_.removeElement(element) && elementListeners_ != null) //@P2C
fireElementEvent(ElementEvent.ELEMENT_REMOVED);
}
/**
* Removes this ElementListener from the internal list.
* If the ElementListener is not on the list, nothing is done.
*
* @see #addElementListener
*
* @param listener The ElementListener.
**/
public void removeElementListener(ElementListener listener)
{
if (listener == null)
throw new NullPointerException ("listener");
if (elementListeners_ != null) elementListeners_.removeElement(listener); //@P2C
}
/**
* Removes the PropertyChangeListener from the internal list.
* If the PropertyChangeListener is not on the list, nothing is done.
*
* @see #addPropertyChangeListener
* @param listener The PropertyChangeListener.
**/
public void removePropertyChangeListener(PropertyChangeListener listener)
{
if (listener == null)
throw new NullPointerException("listener");
if (changes_ != null) changes_.removePropertyChangeListener(listener); //@P2C
}
/**
* Indicates which HTMLTreeElement is selected. The hashcode is used
* to determine which element within the tree to expand or collapse.
*
* @param hashcode The hashcode.
**/
public void selected(int hashcode)
{
if (!isLeaf())
{
if (this.hashCode() == hashcode)
{
boolean old = expanded_;
expanded_ = !expanded_;
if (changes_ != null) changes_.firePropertyChange("selected", Boolean.valueOf(old), Boolean.valueOf(expanded_)); //@P2C
}
else
{
int size = branches_.getCount(); //@P2A
Object[] data = branches_.getData(); //@P2A
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy