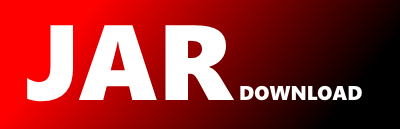
com.ibm.as400.micro.ConnectionHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jt400 Show documentation
Show all versions of jt400 Show documentation
The Open Source version of the IBM Toolbox for Java
The newest version!
///////////////////////////////////////////////////////////////////////////////
//
// JTOpen (IBM Toolbox for Java - OSS version)
//
// Filename: ConnectionHandler.java
//
// The source code contained herein is licensed under the IBM Public License
// Version 1.0, which has been approved by the Open Source Initiative.
// Copyright (C) 1997-2001 International Business Machines Corporation and
// others. All rights reserved.
//
///////////////////////////////////////////////////////////////////////////////
package com.ibm.as400.micro;
import java.io.*;
import java.sql.*;
import com.ibm.as400.access.Trace;
/**
* The ConnectionHandler class is designed to handle all interactions
* needed by the JDBC-ME driver with the JDBC Connection interface.
**/
class ConnectionHandler
{
private static final String copyright = "Copyright (C) 1997-2001 International Business Machines Corporation and others.";
private JdbcMeService service_;
private MicroDataInputStream in_;
private MicroDataOutputStream out_;
/**
Constructor. Creates a JDBC-ME handler for Connection objects.
* @param jdbcme
* @param in
* @param out
**/
public ConnectionHandler(JdbcMeService jdbcme, MicroDataInputStream in, MicroDataOutputStream out)
{
service_ = jdbcme;
in_ = in;
out_ = out;
}
/**
The process function routes the function id and the Connection
to the proper handler.
* @param connection
* @param funcId
* @throws IOException
**/
public void process(Connection connection, int funcId) throws IOException
{
switch (funcId)
{
case MEConstants.CONN_CLOSE:
close(connection);
break;
case MEConstants.CONN_CREATE_STATEMENT:
createStatement(connection);
break;
case MEConstants.CONN_CREATE_STATEMENT2:
createStatement2(connection);
break;
case MEConstants.CONN_PREPARE_STATEMENT:
prepareStatement(connection);
break;
case MEConstants.CONN_SET_AUTOCOMMIT:
setAutoCommit(connection);
break;
case MEConstants.CONN_SET_TRANSACTION_ISOLATION:
setTransactionIsolation(connection);
break;
case MEConstants.CONN_COMMIT:
commit(connection);
break;
case MEConstants.CONN_ROLLBACK:
rollback(connection);
break;
default:
// TODO: This is an exception condition...
if (Trace.isTraceOn())
Trace.log(Trace.ERROR, "Connection Function ID not recognized - function code: " + funcId);
break;
}
}
/**
Closes the Connection object. This function has the side effect
of closing all Statements and ResultSets that are open under the
connection as well. Unlike most of the methods of this class,
if an exception occurs while closing the Connection, this method
will not report it back to the caller in any way. It is simply
swollowed and a message is logged concerning the failure.
The data flow is as follows:
- nothing more
© 2015 - 2025 Weber Informatics LLC | Privacy Policy