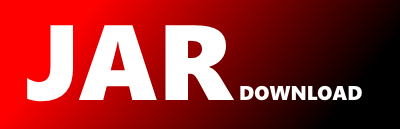
src.com.ibm.as400.access.doc-files.LDRWExample.html Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jt400 Show documentation
Show all versions of jt400 Show documentation
The Open Source version of the IBM Toolbox for Java
IBM Toolbox for Java Example: Using LineDataRecordWriter
Example: Using LineDataRecordWriter to create a line data spooled file
Note: Read the Code example disclaimer
for important legal information.
/////////////////////////////////////////////////////////////////////
//
// LineDataRecordWriter example. This program uses the line data
// record writer access class to create a line data spooled file
// on the system.
//
// This source is an example of using the IBM Toolbox for Java
// "LineDataRecordWriter" class.
//
////////////////////////////////////////////////////////////////////////
import com.ibm.as400.access.*;
import java.io.*;
import java.math.BigDecimal;
public class TestA {
//Private
private static int ccsid_ = -1; // local ccsid variable
private static AS400 system_ = null; // the system
private static SequentialFile file_ = null; // the file
/**
** Create the record field descriptions and record format.
**/
public static RecordFormat initializeRecordFormat()
{
// Create the record format.
RecordFormat qcustcdt = new RecordFormat();
// Create record field descriptions for the record format.
ZonedDecimalFieldDescription customerNumber =
new ZonedDecimalFieldDescription(new AS400ZonedDecimal(6,0),
"CUSNUM");
CharacterFieldDescription lastName =
new CharacterFieldDescription(new AS400Text(8, ccsid_, system_), "LSTNAM");
CharacterFieldDescription initials =
new CharacterFieldDescription(new AS400Text(3, ccsid_, system_), "INIT");
CharacterFieldDescription street =
new CharacterFieldDescription(new AS400Text(13, ccsid_, system_), "STREET");
CharacterFieldDescription city =
new CharacterFieldDescription(new AS400Text(6, ccsid_, system_), "CITY");
CharacterFieldDescription state =
new CharacterFieldDescription(new AS400Text(2, ccsid_, system_), "STATE");
ZonedDecimalFieldDescription zipCode =
new ZonedDecimalFieldDescription(new AS400ZonedDecimal(5,0),
"ZIPCOD");
ZonedDecimalFieldDescription creditLimit =
new ZonedDecimalFieldDescription(new AS400ZonedDecimal(4,0),
"CDTLMT");
ZonedDecimalFieldDescription chargeCode =
new ZonedDecimalFieldDescription(new AS400ZonedDecimal(1,0),
"CHGCOD");
ZonedDecimalFieldDescription balanceDue =
new ZonedDecimalFieldDescription(new AS400ZonedDecimal(6,2),
"BALDUE");
ZonedDecimalFieldDescription creditDue =
new ZonedDecimalFieldDescription(new AS400ZonedDecimal(6,2),
"CDTDUE");
// assign constants from FieldDescription class
int justLeft = FieldDescription.ALIGN_LEFT;
int justRight = FieldDescription.ALIGN_RIGHT;
// set the length and alignment attributes for writing the fields
// The length indicates how many characters the field is, and
// justification indicates where in the layout field the data
// should be placed.
customerNumber.setLayoutAttributes(10,justLeft);
lastName.setLayoutAttributes(10,justLeft);
initials.setLayoutAttributes(4,justLeft);
street.setLayoutAttributes(15,justLeft);
city.setLayoutAttributes(10,justLeft);
state.setLayoutAttributes(3,justLeft);
zipCode.setLayoutAttributes(5,justLeft);
creditLimit.setLayoutAttributes(10,justRight);
chargeCode.setLayoutAttributes(3,justRight);
balanceDue.setLayoutAttributes(10,justRight);
creditDue.setLayoutAttributes(10,justRight);
// set the record format ID
String d = "CUSTRECID";
qcustcdt.setRecordFormatID(d);
// if this were a variable field length record,
// we would set the type and delimiter accordingly. We
// also would not have needed to specify layoutLength and
// layoutAlignment values.
// qcustcdt.setRecordFormatType(RecordFormat.VARIABLE_LAYOUT_LENGTH);
// qcustcdt.setDelimiter(';');
// set the record type to fixed field length
qcustcdt.setRecordFormatType(RecordFormat.FIXED_LAYOUT_LENGTH);
// add the field descriptions to the record format.
qcustcdt.addFieldDescription(customerNumber);
qcustcdt.addFieldDescription(lastName);
qcustcdt.addFieldDescription(initials);
qcustcdt.addFieldDescription(street);
qcustcdt.addFieldDescription(city);
qcustcdt.addFieldDescription(state);
qcustcdt.addFieldDescription(zipCode);
qcustcdt.addFieldDescription(creditLimit);
qcustcdt.addFieldDescription(chargeCode);
qcustcdt.addFieldDescription(balanceDue);
qcustcdt.addFieldDescription(creditDue);
return qcustcdt;
}
/**
** Creates the actual record with data
**/
public static void createRecord(Record record)
{
record.setField("CUSNUM", new BigDecimal(323));
record.setField("LSTNAM", "Johnson");
record.setField("INIT", "B E");
record.setField("STREET", "5234 Elm St");
record.setField("CITY", "Rchstr");
record.setField("STATE", "MN");
record.setField("ZIPCOD", new BigDecimal(55901));
record.setField("CDTLMT", new BigDecimal(5000.00));
record.setField("CHGCOD", new BigDecimal(3));
record.setField("BALDUE", new BigDecimal(25.00));
record.setField("CDTDUE", new BigDecimal(0.00));
}
public static void main(String[]args) {
// create an instance of the system
system_ = new AS400("SYSTEMA", "JOE", "PGMR");
// create a ccsid
ccsid_ = system_.getCcsid();
// create output queue and specify spooled file data to be *LINE
OutputQueue outQ = new OutputQueue(system_, "/QSYS.LIB/QUSRSYS.LIB/LDRW.OUTQ");
PrintParameterList parms = new PrintParameterList();
parms.setParameter(PrintObject.ATTR_PRTDEVTYPE, "*LINE");
parms.setParameter(PrintObject.ATTR_PAGDFN,"/QSYS.LIB.QUSRSYS.LIB/LDRW.PAGDFN");
parms.setParameter(PrintObject.ATTR_CONVERT_LINEDATA,"*YES");
// initialize the record format for writing data
RecordFormat recfmt = initializeRecordFormat();
// create a record and assign data to be printed...
Record record = new Record(recfmt);
createRecord(record);
SpooledFileOutputStream os = null;
try {
// create the output spooled file to hold the record data
os = new SpooledFileOutputStream(system_, parms, null, outQ);
}
if (os != null) { // Output stream was created successfully!
LineDataRecordWriter ldw;
try {
// create the line data record writer
ldw = new LineDataRecordWriter(os, ccsid_, system_);
// write the record of data
ldw.writeRecord(record);
}
catch (IOException e) {
System.out.println("Error occurred writing record data");
}
// close the output stream (spooled file)
try {
os.close();
}
catch (Exception e) {
System.out.println("Error occurred closing output stream.");
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy