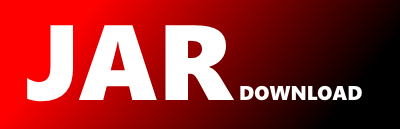
edu.uci.ics.jung.algorithms.util.KMeansClusterer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jung-algorithms Show documentation
Show all versions of jung-algorithms Show documentation
Algorithms for the JUNG project
/*
* Copyright (c) 2003, The JUNG Authors
*
* All rights reserved.
*
* This software is open-source under the BSD license; see either
* "license.txt" or
* https://github.com/jrtom/jung/blob/master/LICENSE for a description.
*/
/*
* Created on Aug 9, 2004
*
*/
package edu.uci.ics.jung.algorithms.util;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Map;
import java.util.Random;
import java.util.Set;
/**
* Groups items into a specified number of clusters, based on their proximity in
* d-dimensional space, using the k-means algorithm. Calls to
* cluster
will terminate when either of the two following
* conditions is true:
*
* - the number of iterations is >
max_iterations
* - none of the centroids has moved as much as
convergence_threshold
* since the previous iteration
*
*
* @author Joshua O'Madadhain
*/
public class KMeansClusterer
{
protected int max_iterations;
protected double convergence_threshold;
protected Random rand;
/**
* Creates an instance which will terminate when either the maximum number of
* iterations has been reached, or all changes are smaller than the convergence threshold.
* @param max_iterations the maximum number of iterations to employ
* @param convergence_threshold the smallest change we want to track
*/
public KMeansClusterer(int max_iterations, double convergence_threshold)
{
this.max_iterations = max_iterations;
this.convergence_threshold = convergence_threshold;
this.rand = new Random();
}
/**
* Creates an instance with max iterations of 100 and convergence threshold
* of 0.001.
*/
public KMeansClusterer()
{
this(100, 0.001);
}
/**
* @return the maximum number of iterations
*/
public int getMaxIterations()
{
return max_iterations;
}
/**
* @param max_iterations the maximum number of iterations
*/
public void setMaxIterations(int max_iterations)
{
if (max_iterations < 0)
throw new IllegalArgumentException("max iterations must be >= 0");
this.max_iterations = max_iterations;
}
/**
* @return the convergence threshold
*/
public double getConvergenceThreshold()
{
return convergence_threshold;
}
/**
* @param convergence_threshold the convergence threshold
*/
public void setConvergenceThreshold(double convergence_threshold)
{
if (convergence_threshold <= 0)
throw new IllegalArgumentException("convergence threshold " +
"must be > 0");
this.convergence_threshold = convergence_threshold;
}
/**
* Returns a Collection
of clusters, where each cluster is
* represented as a Map
of Objects
to locations
* in d-dimensional space.
* @param object_locations a map of the items to cluster, to
* double
arrays that specify their locations in d-dimensional space.
* @param num_clusters the number of clusters to create
* @return a clustering of the input objects in d-dimensional space
* @throws NotEnoughClustersException if {@code num_clusters} is larger than the number of
* distinct points in object_locations
*/
@SuppressWarnings("unchecked")
public Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy