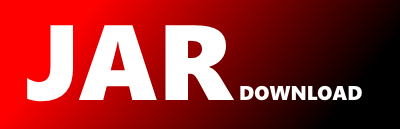
edu.uci.ics.jung.visualization.util.DefaultChangeEventSupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jung-visualization Show documentation
Show all versions of jung-visualization Show documentation
Core visualization support for the JUNG project
The newest version!
/*
* Copyright (c) 2005, The JUNG Authors
* All rights reserved.
*
* This software is open-source under the BSD license; see either "license.txt"
* or https://github.com/jrtom/jung/blob/master/LICENSE for a description.
*
* Created on Aug 18, 2005
*/
package edu.uci.ics.jung.visualization.util;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import javax.swing.event.EventListenerList;
/**
* Basic implementation of ChangeEventSupport, using
* standard jdk classes
*
* @author Tom Nelson - [email protected]
*
*/
public class DefaultChangeEventSupport implements ChangeEventSupport {
Object eventSource;
/**
* holds the registered listeners
*/
protected EventListenerList listenerList = new EventListenerList();
/**
* Only one ChangeEvent
is needed
* instance since the
* event's only state is the source property. The source of events
* generated is always "this".
*/
protected transient ChangeEvent changeEvent;
public DefaultChangeEventSupport(Object eventSource) {
this.eventSource = eventSource;
}
public void addChangeListener(ChangeListener l) {
listenerList.add(ChangeListener.class, l);
}
public void removeChangeListener(ChangeListener l) {
listenerList.remove(ChangeListener.class, l);
}
public ChangeListener[] getChangeListeners() {
return listenerList.getListeners(ChangeListener.class);
}
/**
* Notifies all listeners that have registered interest for
* notification on this event type. The event instance
* is lazily created.
* The primary listeners will be views that need to be repainted
* because of changes in this model instance
* @see EventListenerList
*/
public void fireStateChanged() {
// Guaranteed to return a non-null array
Object[] listeners = listenerList.getListenerList();
// Process the listeners last to first, notifying
// those that are interested in this event
for (int i = listeners.length-2; i>=0; i-=2) {
if (listeners[i]==ChangeListener.class) {
// Lazily create the event:
if (changeEvent == null)
changeEvent = new ChangeEvent(eventSource);
((ChangeListener)listeners[i+1]).stateChanged(changeEvent);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy