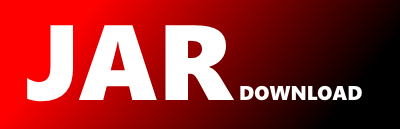
net.sf.kdgcommons.collections.CombiningIterable Maven / Gradle / Ivy
Show all versions of kdgcommons Show documentation
// Copyright Keith D Gregory
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package net.sf.kdgcommons.collections;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.NoSuchElementException;
/**
* Produces an iterator that will iterate over an array of Iterable
s.
*
* The concurrent modification behavior of the iterators produced by this class is
* undefined. You should not rely on being able to modify a collection prior to
* its place in the sequence of iterators.
*
* Removal via the produced iterators is dependent on the underlying collection's
* iterator. It is possible to mix collections that support removal with those
* that do not; the combined iterator will throw for part of the iteration, and
* not throw for another part. It's best not to use remove()
unless
* you know that all underlying collections support it.
*
* @since 1.0.1
*/
public class CombiningIterable
implements Iterable
{
private List> iterables = new ArrayList>();
/**
* Constructs an instance from zero or more explicit iterable objects.
*/
public CombiningIterable(Iterable ... iterables)
{
for (Iterable iterable : iterables)
{
this.iterables.add(iterable);
}
}
/**
* Constructs an instance from an iterable of iterables.
*
* @since 1.0.18
*/
public CombiningIterable(Iterable> iterables)
{
for (Iterable iterable : iterables)
{
this.iterables.add(iterable);
}
}
public Iterator iterator()
{
LinkedList> iterators = new LinkedList>();
for (Iterable iterable : iterables)
iterators.add(iterable.iterator());
return new CombiningIterator(iterators);
}
/**
* Combines a list of iterators into a single iterator. Exposed for those
* callers that don't want to stick to Iterable
s.
*/
public static class CombiningIterator
implements Iterator
{
private LinkedList> _iterators;
private Iterator _curItx;
public CombiningIterator(Iterator... iterators)
{
_iterators = new LinkedList>();
for (Iterator itx : iterators)
_iterators.add(itx);
}
public CombiningIterator(LinkedList> iterators)
{
_iterators = iterators;
}
public boolean hasNext()
{
if ((_curItx != null) && _curItx.hasNext())
return true;
if (_iterators.size() == 0)
return false;
_curItx = _iterators.removeFirst();
return hasNext();
}
public E next()
{
if (hasNext())
return _curItx.next();
else
throw new NoSuchElementException();
}
public void remove()
{
if (_curItx != null)
_curItx.remove();
}
}
}