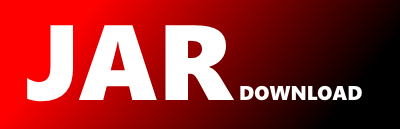
net.sf.mmm.util.pojo.base.GuessingPojoFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mmm-util-pojo Show documentation
Show all versions of mmm-util-pojo Show documentation
This project provides common utitlities to for POJOs.
The newest version!
/* Copyright (c) The m-m-m Team, Licensed under the Apache License, Version 2.0
* http://www.apache.org/licenses/LICENSE-2.0 */
package net.sf.mmm.util.pojo.base;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import net.sf.mmm.util.collection.api.CollectionFactoryManager;
import net.sf.mmm.util.reflect.api.InstantiationFailedException;
/**
* This is the default implementation of the {@link net.sf.mmm.util.pojo.api.PojoFactory} interface.
* In advance to {@link DefaultPojoFactory} it tries to find implementations for interfaces by guessing according to
* conventions:
* For an interface {@code some.package.api.Foo} it will try to find the following classes...
*
* - {@code some.package.api.FooImpl}
* - {@code some.package.api.impl.FooImpl}
* - {@code some.package.impl.FooImpl} (only if package of interface ends with ".api")
*
*
* @author Joerg Hohwiller (hohwille at users.sourceforge.net)
* @since 1.1.0
*/
public class GuessingPojoFactory extends DefaultPojoFactory {
private static final Logger LOG = LoggerFactory.getLogger(GuessingPojoFactory.class);
/** The conventional suffix for an implementation class. */
private static final String IMPL_CLASS_SUFFIX = "Impl";
/** The conventional suffix for an implementation package. */
private static final String IMPL_PKG_SUFFIX = "impl.";
/** The conventional suffix for an api package. */
private static final String API_PKG_SUFFIX = "api.";
/**
* The constructor.
*/
public GuessingPojoFactory() {
super();
}
/**
* The constructor.
*
* @param collectionFactoryManager is the {@link CollectionFactoryManager} instance used to create
* {@link java.util.Map}s and {@link java.util.Collection}s.
*/
public GuessingPojoFactory(CollectionFactoryManager collectionFactoryManager) {
super(collectionFactoryManager);
}
@Override
protected POJO newInstanceForInterface(Class pojoInterface) throws InstantiationFailedException {
POJO pojo = super.newInstanceForInterface(pojoInterface);
if (pojo == null) {
String packageName = pojoInterface.getPackage().getName() + ".";
String className = pojoInterface.getSimpleName() + IMPL_CLASS_SUFFIX;
Class> implementation = null;
try {
implementation = Class.forName(packageName + className);
} catch (ClassNotFoundException e) {
try {
implementation = Class.forName(packageName + IMPL_PKG_SUFFIX + className);
} catch (ClassNotFoundException e1) {
if (packageName.endsWith(API_PKG_SUFFIX)) {
String implPackageName = packageName.substring(0, packageName.length() - API_PKG_SUFFIX.length());
try {
implementation = Class.forName(implPackageName + className);
} catch (ClassNotFoundException e2) {
// ignore...
}
}
}
}
if (implementation != null) {
if (pojoInterface.isAssignableFrom(implementation)) {
pojo = pojoInterface.cast(newInstanceForClass(implementation));
} else {
LOG.warn("Class '" + implementation + "' does NOT implement '" + pojoInterface + "'!");
}
}
}
return pojo;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy