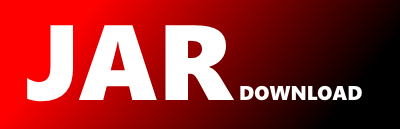
net.sf.mardao.domain.Entity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mardao-maven-plugin Show documentation
Show all versions of mardao-maven-plugin Show documentation
Mardao is the Architect's Java DAO generator for use with Maven
package net.sf.mardao.domain;
/*
* #%L
* net.sf.mardao:mardao-maven-plugin
* %%
* Copyright (C) 2010 - 2014 Wadpam
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.TreeMap;
import java.util.TreeSet;
import net.sf.mardao.plugin.ProcessDomainMojo;
/**
* The domain object for Entities in the class graph.
*
* @author f94os
*
*/
public class Entity implements Comparable {
private Class clazz;
private String tableName;
private Field parent;
private Field pk;
private final Set fields = new TreeSet();
private final Set oneToOnes = new TreeSet();
private final Set manyToOnes = new TreeSet();
private final Set manyToManys = new TreeSet();
private final List> uniqueConstraints = new ArrayList>();
private final Map mappedBy = new HashMap();
private final Set dependsOn = new TreeSet();
private List ancestors = new ArrayList();
private List parents = new ArrayList();
private final Set children = new TreeSet();
private Field geoLocation;
private Field createdDate;
private Field createdBy;
private Field updatedDate;
private Field updatedBy;
public Class getClazz() {
return clazz;
}
public void setClazz(Class clazz) {
this.clazz = clazz;
}
public String getClassName() {
return clazz.getName();
}
public String getSimpleName() {
return clazz.getSimpleName();
}
public String getSimpleLower() {
return ProcessDomainMojo.firstToLower(clazz.getSimpleName());
}
public Field getPk() {
return pk;
}
public void setPk(Field pk) {
this.pk = pk;
}
public Set getFields() {
return fields;
}
public Set getManyToOnes() {
return manyToOnes;
}
public List> getUniqueConstraints() {
return uniqueConstraints;
}
public boolean isUnique(String fieldName) {
for(Field f : getOneToOnes()) {
if (f.getName().equals(fieldName)) {
return true;
}
}
for(Set uniqueConstraint : uniqueConstraints) {
if (1 == uniqueConstraint.size() && uniqueConstraint.contains(fieldName)) {
return true;
}
}
return false;
}
public Map getAllFields() {
final Map returnValue = new TreeMap();
for(Field f : getFields()) {
returnValue.put(f.getName(), f);
}
for(Field f : getOneToOnes()) {
returnValue.put(f.getName(), f);
}
for(Field f : getManyToOnes()) {
returnValue.put(f.getName(), f);
}
for(Field f : getManyToManys()) {
returnValue.put(f.getName(), f);
}
return returnValue;
}
public List> getUniqueFieldsSets() {
final List> returnValue = new ArrayList>();
Map allFields = getAllFields();
for(Set uniqueConstraint : uniqueConstraints) {
if (1 < uniqueConstraint.size()) {
Set fieldsSet = new TreeSet();
for(String fieldName : uniqueConstraint) {
for(Field column : allFields.values()) {
if (fieldName.equals(column.getColumnName())) {
fieldsSet.add(column);
break;
}
}
if (null != parent && parent.getName().equals(fieldName)) {
fieldsSet.add(parent);
}
}
returnValue.add(fieldsSet);
}
}
return returnValue;
}
public Field getFirstUniqueField() {
Field returnValue = null;
for(Entry entry : getAllFields().entrySet()) {
if (isUnique(entry.getKey())) {
returnValue = entry.getValue();
break;
}
}
return returnValue;
}
public Set getManyToManys() {
return manyToManys;
}
public Map getMappedBy() {
return mappedBy;
}
@Override
public String toString() {
return getClassName() + "<" + getSimpleName() + ">";
}
public void setTableName(String tableName) {
this.tableName = tableName;
}
/**
* If there is no @@Table(name)
annotation, just return the simpleName
*
* @return
*/
public String getTableName() {
if (null == tableName) {
return getSimpleName();
}
return tableName;
}
public Set getOneToOnes() {
return oneToOnes;
}
public Set getDependsOn() {
return dependsOn;
}
@Override
public int compareTo(Entity other) {
return this.getClassName().compareTo(other.getClassName());
}
public void setParent(Field parent) {
this.parent = parent;
}
public Field getParent() {
return parent;
}
public void setAncestors(List ancestors) {
this.ancestors = ancestors;
}
public List getAncestors() {
return ancestors;
}
public void setParents(List parents) {
this.parents = parents;
}
public List getParents() {
return parents;
}
public Set getChildren() {
return children;
}
public final Field getCreatedDate() {
return createdDate;
}
public final void setCreatedDate(Field createdDate) {
this.createdDate = createdDate;
}
public final Field getCreatedBy() {
return createdBy;
}
public final void setCreatedBy(Field createdBy) {
this.createdBy = createdBy;
}
public final Field getUpdatedDate() {
return updatedDate;
}
public final void setUpdatedDate(Field updatedDate) {
this.updatedDate = updatedDate;
}
public final Field getUpdatedBy() {
return updatedBy;
}
public final void setUpdatedBy(Field updatedBy) {
this.updatedBy = updatedBy;
}
public Field getGeoLocation() {
return geoLocation;
}
public void setGeoLocation(Field geoLocation) {
this.geoLocation = geoLocation;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy