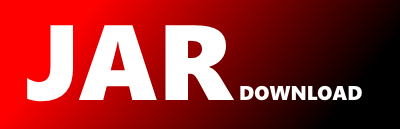
meka.experiment.evaluators.RepeatedRuns Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of meka Show documentation
Show all versions of meka Show documentation
The MEKA project provides an open source implementation of methods for multi-label classification and evaluation. It is based on the WEKA Machine Learning Toolkit. Several benchmark methods are also included, as well as the pruned sets and classifier chains methods, other methods from the scientific literature, and a wrapper to the MULAN framework.
The newest version!
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* RepeatedRuns.java
* Copyright (C) 2015-2017 University of Waikato, Hamilton, NZ
*/
package meka.experiment.evaluators;
import meka.classifiers.multilabel.MultiLabelClassifier;
import meka.core.OptionUtils;
import meka.core.ThreadLimiter;
import meka.core.ThreadUtils;
import meka.events.LogListener;
import meka.experiment.evaluationstatistics.EvaluationStatistics;
import weka.core.Instances;
import weka.core.Option;
import weka.core.Randomizable;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
import java.util.Vector;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.RejectedExecutionException;
import java.util.concurrent.TimeUnit;
/**
* Repeatedly executes the base evaluator.
*
* @author FracPete (fracpete at waikato dot ac dot nz)
* @version $Revision$
*/
public class RepeatedRuns
extends AbstractMetaEvaluator
implements ThreadLimiter {
private static final long serialVersionUID = -1230107553603089463L;
/** the key for the run number. */
public final static String KEY_RUN = "Run";
/** the lower number of runs (included). */
protected int m_LowerRuns = getDefaultLowerRuns();
/** the upper number of runs (included). */
protected int m_UpperRuns = getDefaultUpperRuns();
/** the number of threads to use for parallel execution. */
protected int m_NumThreads = getDefaultNumThreads();
/** the actual number of threads to use. */
protected int m_ActualNumThreads;
/** the executor service to use for parallel execution. */
protected transient ExecutorService m_Executor;
/**
* Description to be displayed in the GUI.
*
* @return the description
*/
public String globalInfo() {
return "Performs repeated runs of the base evaluator. If the base evaluator is randomizable, "
+ "the run number is used as seed. The base evaluator gets initialized before each "
+ "run.";
}
/**
* Returns the default evaluator to use.
*
* @return the default
*/
@Override
protected Evaluator getDefaultEvaluator() {
return new CrossValidation();
}
/**
* Returns the default lower number of runs to perform.
*
* @return the default
*/
protected int getDefaultLowerRuns() {
return 1;
}
/**
* Sets the lower number of runs to perform (included).
*
* @param value the number of runs
*/
public void setLowerRuns(int value) {
m_LowerRuns = value;
}
/**
* Returns the lower number of runs to perform (included).
*
* @return the number of runs
*/
public int getLowerRuns() {
return m_LowerRuns;
}
/**
* Describes this property.
*
* @return the description
*/
public String lowerRunsTipText() {
return "The lower number of runs to perform (included).";
}
/**
* Returns the default upper number of runs to perform.
*
* @return the default
*/
protected int getDefaultUpperRuns() {
return 10;
}
/**
* Sets the upper number of runs to perform (included).
*
* @param value the number of runs
*/
public void setUpperRuns(int value) {
m_UpperRuns = value;
}
/**
* Returns the upper number of runs to perform (included).
*
* @return the number of runs
*/
public int getUpperRuns() {
return m_UpperRuns;
}
/**
* Describes this property.
*
* @return the description
*/
public String upperRunsTipText() {
return "The upper number of runs to perform (included).";
}
/**
* Returns the default number of threads to use.
*
* @return the number of threads: -1 = # of CPUs/cores; 0/1 = sequential execution
*/
protected int getDefaultNumThreads() {
return ThreadUtils.SEQUENTIAL;
}
/**
* Sets the number of threads to use.
*
* @param value the number of threads: -1 = # of CPUs/cores; 0/1 = sequential execution
*/
public void setNumThreads(int value) {
if (value >= -1) {
m_NumThreads = value;
}
else {
log("Number of threads must be >= -1, provided: " + value);
}
}
/**
* Returns the number of threads to use.
*
* @return the number of threads: -1 = # of CPUs/cores; 0/1 = sequential execution
*/
public int getNumThreads() {
return m_NumThreads;
}
/**
* Returns the tip text for this property.
*
* @return tip text for this property suitable for
* displaying in the GUI or for listing the options.
*/
public String numThreadsTipText() {
return "The number of threads to use ; -1 = number of CPUs/cores; 0 or 1 = sequential execution.";
}
/**
* Returns an enumeration of all the available options..
*
* @return an enumeration of all available options.
*/
@Override
public Enumeration
© 2015 - 2025 Weber Informatics LLC | Privacy Policy