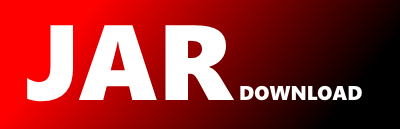
net.sf.mgp.javafx.property.NestedJavaBeanProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javafx-properties Show documentation
Show all versions of javafx-properties Show documentation
Utilities to create JavaFX properties for JavaBeans
The newest version!
package net.sf.mgp.javafx.property;
import javafx.beans.property.adapter.JavaBeanObjectPropertyBuilder;
import javafx.beans.property.adapter.JavaBeanProperty;
import javafx.beans.property.adapter.ReadOnlyJavaBeanObjectPropertyBuilder;
import javafx.beans.property.adapter.ReadOnlyJavaBeanProperty;
import javafx.beans.value.ObservableValue;
/**
* A helper class with utility functions to create nested JavaBean properties.
*
*/
public class NestedJavaBeanProperties {
private NestedJavaBeanProperties() {
}
/**
* Creates a nested property, such as a.b.c
. The value of the property will be c
, or null
if c
could not
* be reached (due to b
not having a c
property, b
being null
, or c
not being the right
* type etc.).
*
* All traversed classes and properties have to be public.
*
*
* @param the type of the final property
* @param root the root bean
* @param firstStep the property of the root
bean, the first property to reach the final property
* @param otherSteps the other properties to reach the final property. Start from the property accessed via the firstStep
property of the
* root
bean.
* @return the new {@link JavaBeanProperty}
*/
public static JavaBeanProperty select(Object root, String firstStep, String... otherSteps) {
try {
if (otherSteps.length == 0) {
return JavaBeanObjectPropertyBuilder.create().bean(root).name(firstStep).build();
}
ReadOnlyJavaBeanProperty> parent = ReadOnlyJavaBeanObjectPropertyBuilder.create().bean(root).name(firstStep).build();
return select(parent, otherSteps);
} catch (NoSuchMethodException e) {
throw new IllegalArgumentException("Illegal property " + firstStep, e);
}
}
/**
* Creates a nested property, such as a.b.c
. The value of the property will be c
, or null
if c
could not
* be reached (due to b
not having a c
property, b
being null
, or c
not being the right
* type etc.).
*
* All traversed classes and properties have to be public.
*
*
* @param the type of the final property
* @param root The root {@link ObservableValue}
* @param firstStep the property of the root
bean, the first property to reach the final property
* @param otherSteps The other property names to reach the final property. Start from the property accessed via the firstStep
property of the
* root
bean.
* @return the new {@link JavaBeanProperty}
*/
public static JavaBeanProperty select(ObservableValue> root, String firstStep, String... otherSteps) {
return select(new ObservableValueAdapter<>(root), concat(firstStep, otherSteps));
}
private static JavaBeanProperty select(ReadOnlyJavaBeanProperty> parent, String... steps) {
for (int i = 0; i < steps.length - 1; i++) {
parent = new NestedReadOnlyJavaBeanObjectProperty(parent, steps[i]);
}
return new NestedJavaBeanObjectProperty<>(parent, steps[steps.length - 1]);
}
/**
* Creates a read-only nested property, such as a.b.c
. The value of the property will be c
, or null
if c
* could not be reached (due to b
not having a c
property, b
being null
, or c
not being the
* right type etc.).
*
* All traversed classes and properties have to be public.
*
*
* @param the type of the final property
* @param root The root bean
* @param firstStep the property of the root
bean, the first property to reach the final property
* @param otherSteps The other property names to reach the final property. Start from the property accessed via the firstStep
property of the
* root
bean.
* @return the new {@link ReadOnlyJavaBeanProperty}
*/
public static ReadOnlyJavaBeanProperty selectReadOnly(Object root, String firstStep, String... otherSteps) {
try {
ReadOnlyJavaBeanProperty> current = ReadOnlyJavaBeanObjectPropertyBuilder.create().bean(root).name(firstStep).build();
return selectReadOnly(current, otherSteps);
} catch (NoSuchMethodException e) {
throw new IllegalArgumentException("Illegal property " + firstStep, e);
}
}
/**
* Creates a read-only nested property, such as a.b.c
. The value of the property will be c
, or null
if c
* could not be reached (due to b
not having a c
property, b
being null
, or c
not being the
* right type etc.).
*
* All traversed classes and properties have to be public.
*
*
* @param the type of the final property
* @param root The root {@link ObservableValue}
* @param firstStep the property of the root
bean, the first property to reach the final property
* @param otherSteps The other property names to reach the final property. Start from the property accessed via the firstStep
property of the
* root
bean.
* @return the new {@link ReadOnlyJavaBeanProperty}
*/
public static ReadOnlyJavaBeanProperty selectReadOnly(ObservableValue> root, String firstStep, String... otherSteps) {
return selectReadOnly(new ObservableValueAdapter<>(root), concat(firstStep, otherSteps));
}
private static ReadOnlyJavaBeanProperty selectReadOnly(ReadOnlyJavaBeanProperty> parent, String... steps) {
for (String step : steps) {
parent = new NestedReadOnlyJavaBeanObjectProperty(parent, step);
}
return (ReadOnlyJavaBeanProperty) parent;
}
private static String[] concat(String firstStep, String[] otherSteps) {
String[] result = new String[otherSteps.length + 1];
result[0] = firstStep;
System.arraycopy(otherSteps, 0, result, 1, otherSteps.length);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy