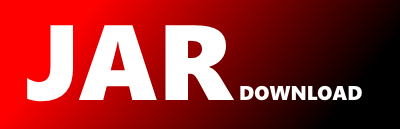
net.sf.mmm.util.nls.AbstractResourceBundle Maven / Gradle / Ivy
The newest version!
/* $Id: AbstractResourceBundle.java 402 2008-01-14 21:09:00Z hohwille $
* Copyright (c) The m-m-m Team, Licensed under the Apache License, Version 2.0
* http://www.apache.org/licenses/LICENSE-2.0 */
package net.sf.mmm.util.nls;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Map;
import java.util.ResourceBundle;
/**
* This is the abstract base class for {@link ResourceBundle} implementations
* using this NLS support. Create your {@link ResourceBundle}s by sub-classing
* this class and simply define some public static final fields that will be
* automatically added to the bundle using reflection (only from constructor).
* Please note that your sub-class must also be public or you need to set
* privileges in the security manager to allow this class reading the fields via
* reflection.
*
* @author Joerg Hohwiller (hohwille at users.sourceforge.net)
*/
public abstract class AbstractResourceBundle extends ResourceBundle {
/**
* The key value pairs; maps keys (String) to values (Object). No Map because
* Enumeration is required...
*/
private Hashtable bundle;
/** the inverse map of {@link #bundle} */
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy