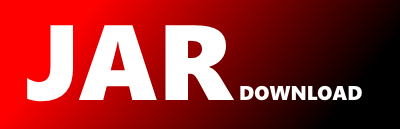
net.sf.mongodb_jdbc_driver.MongoDbConnection Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 Rob Manning
* [email protected]
*
* This file is part of MongoDB JDBC Driver.
*
* MongoDB JDBC Driver is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* MongoDB JDBC Driver is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with MongoDB JDBC Driver. If not, see .
*/
package net.sf.mongodb_jdbc_driver;
import java.net.UnknownHostException;
import java.sql.Array;
import java.sql.Blob;
import java.sql.CallableStatement;
import java.sql.Clob;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.NClob;
import java.sql.PreparedStatement;
import java.sql.SQLClientInfoException;
import java.sql.SQLException;
import java.sql.SQLWarning;
import java.sql.SQLXML;
import java.sql.Savepoint;
import java.sql.Statement;
import java.sql.Struct;
import java.util.Map;
import java.util.Properties;
import net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper;
import net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactory;
import net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactoryImpl;
import com.mongodb.DB;
import com.mongodb.MongoException;
/**
*
*
*/
public class MongoDbConnection implements Connection
{
private final String server;
private final String url;
private final int port;
private String dbName;
private MongoWrapper mongo;
private DB db;
private final Properties connectionProperties;
private MongoWrapperFactory mongoWrapperFactory = new MongoWrapperFactoryImpl();
private boolean isClosed = false;
private boolean isReadOnly = false;
/**
* @param server
* @param port
* @param dbName
* @param url
* @param connectionProperties
* @throws UnknownHostException
*/
public MongoDbConnection(String server, int port, String dbName, String url,
Properties connectionProperties) throws UnknownHostException
{
this.server = server;
this.port = port;
this.dbName = dbName;
this.url = url;
// TODO: We will need to define properties that tell us things such as read preference, write concern
// and replica set addresses, write timeout, connect timeout, etc.. See MongoOptions and the various
// constructors for Mongo.
this.connectionProperties = connectionProperties;
mongo = mongoWrapperFactory.getMongoWrapper(server, port, connectionProperties);
db = mongo.getDB(dbName);
}
/**
* @return
*/
public MongoWrapper getMongoWrapper()
{
return mongo;
}
public T unwrap(Class iface) throws SQLException
{
checkClosed();
// TODO Auto-generated method stub
return null;
}
public boolean isWrapperFor(Class> iface) throws SQLException
{
checkClosed();
// TODO Auto-generated method stub
return false;
}
/**
* @see java.sql.Connection#createStatement()
*/
public Statement createStatement() throws SQLException
{
checkClosed();
return new MongoDbStatement(this);
}
public PreparedStatement prepareStatement(String sql) throws SQLException
{
checkClosed();
// TODO Auto-generated method stub
return null;
}
public CallableStatement prepareCall(String sql) throws SQLException
{
checkClosed();
// TODO Auto-generated method stub
return null;
}
/**
* @see java.sql.Connection#nativeSQL(java.lang.String)
*/
public String nativeSQL(String sql) throws SQLException
{
checkClosed();
throw new UnsupportedOperationException("MongoDB does not support SQL natively.");
}
/**
* @see java.sql.Connection#setAutoCommit(boolean)
*/
public void setAutoCommit(boolean autoCommit) throws SQLException
{
checkClosed();
if (!autoCommit) {
throw new UnsupportedOperationException("MongoDB provides no support for transactions.");
}
}
/**
* @see java.sql.Connection#getAutoCommit()
*/
public boolean getAutoCommit() throws SQLException
{
checkClosed();
return true;
}
/**
* @see java.sql.Connection#commit()
*/
public void commit() throws SQLException
{
checkClosed();
throw new UnsupportedOperationException("MongoDB provides no support for transactions.");
}
/**
* @see java.sql.Connection#rollback()
*/
public void rollback() throws SQLException
{
checkClosed();
throw new UnsupportedOperationException("MongoDB provides no support for transactions.");
}
/**
* @see java.sql.Connection#close()
*/
public void close() throws SQLException
{
isClosed = true;
}
/**
* @see java.sql.Connection#isClosed()
*/
public boolean isClosed() throws SQLException
{
return isClosed;
}
/**
* @see java.sql.Connection#getMetaData()
*/
public DatabaseMetaData getMetaData() throws SQLException
{
checkClosed();
return new MongoDbMetaData(this);
}
/**
* @see java.sql.Connection#setReadOnly(boolean)
*/
public void setReadOnly(boolean readOnly) throws SQLException
{
checkClosed();
isReadOnly = readOnly;
}
/**
* @see java.sql.Connection#isReadOnly()
*/
public boolean isReadOnly() throws SQLException
{
checkClosed();
return isReadOnly;
}
/**
* @see java.sql.Connection#setCatalog(java.lang.String)
*/
public void setCatalog(String catalog) throws SQLException
{
checkClosed();
this.dbName = catalog;
this.db = mongo.getDB(dbName);
}
/**
* @see java.sql.Connection#getCatalog()
*/
public String getCatalog() throws SQLException
{
checkClosed();
return dbName;
}
/**
* @see java.sql.Connection#setTransactionIsolation(int)
*/
public void setTransactionIsolation(int level) throws SQLException
{
checkClosed();
// Since the only valid value for MongDB is Connection.TRANSACTION_NONE, and the javadoc for this method
// indicates that this is not a valid value for level here, throw unsupported operation exception.
throw new UnsupportedOperationException("MongoDB provides no support for transactions.");
}
/**
* @see java.sql.Connection#getTransactionIsolation()
*/
public int getTransactionIsolation() throws SQLException
{
checkClosed();
return Connection.TRANSACTION_NONE;
}
public SQLWarning getWarnings() throws SQLException
{
checkClosed();
// TODO Auto-generated method stub
return null;
}
public void clearWarnings() throws SQLException
{
checkClosed();
// TODO Auto-generated method stub
}
public Statement createStatement(int resultSetType, int resultSetConcurrency) throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public PreparedStatement prepareStatement(String sql, int resultSetType, int resultSetConcurrency)
throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public CallableStatement prepareCall(String sql, int resultSetType, int resultSetConcurrency)
throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public Map> getTypeMap() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public void setTypeMap(Map> map) throws SQLException
{
// TODO Auto-generated method stub
}
public void setHoldability(int holdability) throws SQLException
{
// TODO Auto-generated method stub
}
public int getHoldability() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public Savepoint setSavepoint() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public Savepoint setSavepoint(String name) throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public void rollback(Savepoint savepoint) throws SQLException
{
// TODO Auto-generated method stub
}
public void releaseSavepoint(Savepoint savepoint) throws SQLException
{
// TODO Auto-generated method stub
}
public Statement createStatement(int resultSetType, int resultSetConcurrency, int resultSetHoldability)
throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public PreparedStatement prepareStatement(String sql, int resultSetType, int resultSetConcurrency,
int resultSetHoldability) throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public CallableStatement prepareCall(String sql, int resultSetType, int resultSetConcurrency,
int resultSetHoldability) throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public PreparedStatement prepareStatement(String sql, int autoGeneratedKeys) throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public PreparedStatement prepareStatement(String sql, int[] columnIndexes) throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public PreparedStatement prepareStatement(String sql, String[] columnNames) throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public Clob createClob() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public Blob createBlob() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public NClob createNClob() throws SQLException
{
checkClosed();
// TODO Auto-generated method stub
return null;
}
public SQLXML createSQLXML() throws SQLException
{
checkClosed();
// TODO Auto-generated method stub
return null;
}
/**
* @see java.sql.Connection#isValid(int)
*/
public boolean isValid(int timeout) throws SQLException
{
checkClosed();
try {
mongo.getDatabaseNames();
} catch (MongoException e) {
return false;
}
return true;
}
/**
* @see java.sql.Connection#setClientInfo(java.lang.String, java.lang.String)
*/
public void setClientInfo(String name, String value) throws SQLClientInfoException
{
/* MongoDB does not support setting client information in the database. */
}
/**
* @see java.sql.Connection#setClientInfo(java.util.Properties)
*/
public void setClientInfo(Properties properties) throws SQLClientInfoException
{
/* MongoDB does not support setting client information in the database. */
}
/**
* @see java.sql.Connection#getClientInfo(java.lang.String)
*/
public String getClientInfo(String name) throws SQLException
{
checkClosed();
return null;
}
/**
* @see java.sql.Connection#getClientInfo()
*/
public Properties getClientInfo() throws SQLException
{
checkClosed();
return null;
}
public Array createArrayOf(String typeName, Object[] elements) throws SQLException
{
checkClosed();
// TODO Auto-generated method stub
return null;
}
/**
* @see java.sql.Connection#createStruct(java.lang.String, java.lang.Object[])
*/
public Struct createStruct(String typeName, Object[] attributes) throws SQLException
{
checkClosed();
// TODO Auto-generated method stub
return null;
}
/**
* @return
*/
public String getUrl()
{
return url;
}
/**
* @return
*/
public DB getDb()
{
return this.db;
}
/**
* Injection method.
*
* @param mongoWrapperFactory
*/
public void setMongoWrapperFactory(MongoWrapperFactory mongoWrapperFactory)
{
this.mongoWrapperFactory = mongoWrapperFactory;
}
private void checkClosed() throws SQLException
{
if (isClosed)
{
throw new SQLException("Statement was previously closed.");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy