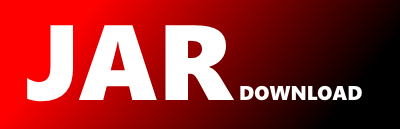
net.sf.mongodb_jdbc_driver.MongoDbDriver Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 Rob Manning
* [email protected]
*
* This file is part of MongoDB JDBC Driver.
*
* MongoDB JDBC Driver is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* MongoDB JDBC Driver is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with MongoDB JDBC Driver. If not, see .
*/
package net.sf.mongodb_jdbc_driver;
import java.net.UnknownHostException;
import java.sql.Connection;
import java.sql.Driver;
import java.sql.DriverManager;
import java.sql.DriverPropertyInfo;
import java.sql.SQLException;
import java.util.Properties;
import net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactory;
import net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactoryImpl;
import org.apache.commons.lang3.math.NumberUtils;
/**
* Driver implementation that provides minimal JDBC implementation for accessing MongoDB instances. The jdbc
* driver url spec is as follows: jdbc:mongodb://[:27017]/ See getPropertyInfo method for
* driver properties that can be specified when getting a Connection to alter the semantics of that
* Connection.
*/
public class MongoDbDriver implements Driver
{
private DriverPropertyInfoHelper propertyInfoHelper = new DriverPropertyInfoHelper();
static
{
try
{
DriverManager.registerDriver(new MongoDbDriver());
}
catch (SQLException e)
{
e.printStackTrace();
}
}
private MongoWrapperFactory mongoWrapperFactory = new MongoWrapperFactoryImpl();
private static final String URL_SPEC = "jdbc:mongodb://[:27017]/";
/**
* @see java.sql.Driver#connect(java.lang.String, java.util.Properties)
*/
public Connection connect(String url, Properties info) throws SQLException
{
final String[] urlParts = url.split(":");
if (urlParts.length < 4)
{
// throw new SQLException("URL specified was not of the form: " + URL_SPEC);
// JDBC JavaDoc for this method says the driver should return null if it is passed the wrong URL
return null;
}
final String serverName = urlParts[2].substring(2);
String dbParts[] = urlParts[3].split("/");
String dbName = null;
int port = 27017;
if (dbParts.length == 1)
{
// We believe this is a database name, and the optional port was omitted.
dbName = dbParts[0];
}
else
{
port = NumberUtils.toInt(dbParts[0], 27017);
dbName = dbParts[1];
}
MongoDbConnection result = null;
try
{
result = new MongoDbConnection(serverName, port, dbName, url, info);
result.setMongoWrapperFactory(mongoWrapperFactory);
}
catch (UnknownHostException e)
{
throw new SQLException("Unexpected exception: " + e.getMessage(), e);
}
return result;
}
/**
* URLs accepted are of the form: jdbc:mongodb://[:27017]/
*
* @see java.sql.Driver#acceptsURL(java.lang.String)
*/
public boolean acceptsURL(String url) throws SQLException
{
System.err.println("Inside acceptsUrl: " + url);
if (url.startsWith("jdbc:mongodb://"))
{
return true;
}
return false;
}
/**
* @see java.sql.Driver#getPropertyInfo(java.lang.String, java.util.Properties)
*/
public DriverPropertyInfo[] getPropertyInfo(String url, Properties info) throws SQLException
{
return propertyInfoHelper.getPropertyInfo();
}
/**
* @see java.sql.Driver#getMajorVersion()
*/
public int getMajorVersion()
{
return 1;
}
/**
* @see java.sql.Driver#getMinorVersion()
*/
public int getMinorVersion()
{
return 0;
}
/**
* @see java.sql.Driver#jdbcCompliant()
*/
public boolean jdbcCompliant()
{
return true;
}
/**
* Injection method.
*
* @param mongoWrapperFactory
*/
public void setMongoWrapperFactory(MongoWrapperFactory mongoWrapperFactory) {
this.mongoWrapperFactory = mongoWrapperFactory;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy