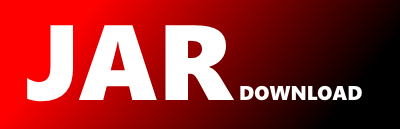
net.sf.mongodb_jdbc_driver.MongoDbMetaData Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 Rob Manning
* [email protected]
*
* This file is part of MongoDB JDBC Driver.
*
* MongoDB JDBC Driver is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* MongoDB JDBC Driver is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with MongoDB JDBC Driver. If not, see .
*/
package net.sf.mongodb_jdbc_driver;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.ResultSet;
import java.sql.RowIdLifetime;
import java.sql.SQLException;
import java.sql.Types;
import java.util.List;
import java.util.Set;
import net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper;
import com.mongodb.CommandResult;
import com.mongodb.DB;
/**
* Mongo databases are equivalent to catalogs for this driver. Schemas aren't used. Mongo collections are
* equivalent to tables, in that each collection is a table.
*/
public class MongoDbMetaData implements DatabaseMetaData
{
private final MongoDbConnection con;
private final MongoWrapper mongo;
private final static MongoDbResultSet EMPTY_RESULT_SET = new MongoDbResultSet();
public final static String OBJECT_ID_TYPE_NAME = "OBJECT_ID";
public final static String DOCUMENT_TYPE_NAME = "DOCUMENT";
public MongoDbMetaData(MongoDbConnection con)
{
this.con = con;
mongo = con.getMongoWrapper();
}
public T unwrap(Class iface) throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public boolean isWrapperFor(Class> iface) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean allProceduresAreCallable() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean allTablesAreSelectable() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
/**
* @see java.sql.DatabaseMetaData#getURL()
*/
public String getURL() throws SQLException
{
return con.getUrl();
}
public String getUserName() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public boolean isReadOnly() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean nullsAreSortedHigh() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean nullsAreSortedLow() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean nullsAreSortedAtStart() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean nullsAreSortedAtEnd() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
/**
* @see java.sql.DatabaseMetaData#getDatabaseProductName()
*/
public String getDatabaseProductName() throws SQLException
{
return "Mongo DB";
}
/**
* @see java.sql.DatabaseMetaData#getDatabaseProductVersion()
*/
public String getDatabaseProductVersion() throws SQLException
{
MongoWrapper mongo = this.con.getMongoWrapper();
CommandResult result = mongo.getDB("local").doEval("db.version()", (Object[]) null);
String[] jsonParts = result.toString().split(",");
String[] keyVal = jsonParts[1].split(":");
return keyVal[1].substring(2, keyVal[1].length() - 2);
}
/**
* @see java.sql.DatabaseMetaData#getDriverName()
*/
public String getDriverName() throws SQLException
{
return "MongoDB JDBC Driver";
}
/**
* @see java.sql.DatabaseMetaData#getDriverVersion()
*/
public String getDriverVersion() throws SQLException
{
return "1.0";
}
/**
* @see java.sql.DatabaseMetaData#getDriverMajorVersion()
*/
public int getDriverMajorVersion()
{
return 1;
}
/**
* @see java.sql.DatabaseMetaData#getDriverMinorVersion()
*/
public int getDriverMinorVersion()
{
return 0;
}
public boolean usesLocalFiles() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean usesLocalFilePerTable() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsMixedCaseIdentifiers() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean storesUpperCaseIdentifiers() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean storesLowerCaseIdentifiers() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean storesMixedCaseIdentifiers() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsMixedCaseQuotedIdentifiers() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean storesUpperCaseQuotedIdentifiers() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean storesLowerCaseQuotedIdentifiers() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean storesMixedCaseQuotedIdentifiers() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public String getIdentifierQuoteString() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public String getSQLKeywords() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public String getNumericFunctions() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public String getStringFunctions() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public String getSystemFunctions() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public String getTimeDateFunctions() throws SQLException
{
return "date";
}
public String getSearchStringEscape() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public String getExtraNameCharacters() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
/**
* @see java.sql.DatabaseMetaData#supportsAlterTableWithAddColumn()
*/
public boolean supportsAlterTableWithAddColumn() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsAlterTableWithDropColumn()
*/
public boolean supportsAlterTableWithDropColumn() throws SQLException
{
return false;
}
public boolean supportsColumnAliasing() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean nullPlusNonNullIsNull() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsConvert() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsConvert(int fromType, int toType) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsTableCorrelationNames() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsDifferentTableCorrelationNames() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsExpressionsInOrderBy() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsOrderByUnrelated() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsGroupBy() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsGroupByUnrelated() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsGroupByBeyondSelect() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsLikeEscapeClause() throws SQLException
{
return true;
}
/**
* @see java.sql.DatabaseMetaData#supportsMultipleResultSets()
*/
public boolean supportsMultipleResultSets() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsMultipleTransactions()
*/
public boolean supportsMultipleTransactions() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsNonNullableColumns()
*/
public boolean supportsNonNullableColumns() throws SQLException
{
return true;
}
/**
* @see java.sql.DatabaseMetaData#supportsMinimumSQLGrammar()
*/
public boolean supportsMinimumSQLGrammar() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsCoreSQLGrammar()
*/
public boolean supportsCoreSQLGrammar() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsExtendedSQLGrammar()
*/
public boolean supportsExtendedSQLGrammar() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsANSI92EntryLevelSQL()
*/
public boolean supportsANSI92EntryLevelSQL() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsANSI92IntermediateSQL()
*/
public boolean supportsANSI92IntermediateSQL() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsANSI92FullSQL()
*/
public boolean supportsANSI92FullSQL() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsIntegrityEnhancementFacility()
*/
public boolean supportsIntegrityEnhancementFacility() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsOuterJoins()
*/
public boolean supportsOuterJoins() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsFullOuterJoins()
*/
public boolean supportsFullOuterJoins() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsLimitedOuterJoins()
*/
public boolean supportsLimitedOuterJoins() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#getSchemaTerm()
*/
public String getSchemaTerm() throws SQLException
{
return null;
}
/**
* @see java.sql.DatabaseMetaData#getProcedureTerm()
*/
public String getProcedureTerm() throws SQLException
{
return null;
}
/**
* @see java.sql.DatabaseMetaData#getCatalogTerm()
*/
public String getCatalogTerm() throws SQLException
{
return "database";
}
/**
* @see java.sql.DatabaseMetaData#isCatalogAtStart()
*/
public boolean isCatalogAtStart() throws SQLException
{
return true;
}
/**
* @see java.sql.DatabaseMetaData#getCatalogSeparator()
*/
public String getCatalogSeparator() throws SQLException
{
return ".";
}
/**
* @see java.sql.DatabaseMetaData#supportsSchemasInDataManipulation()
*/
public boolean supportsSchemasInDataManipulation() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsSchemasInProcedureCalls()
*/
public boolean supportsSchemasInProcedureCalls() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsSchemasInTableDefinitions()
*/
public boolean supportsSchemasInTableDefinitions() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsSchemasInIndexDefinitions()
*/
public boolean supportsSchemasInIndexDefinitions() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsSchemasInPrivilegeDefinitions()
*/
public boolean supportsSchemasInPrivilegeDefinitions() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsCatalogsInDataManipulation()
*/
public boolean supportsCatalogsInDataManipulation() throws SQLException
{
return true;
}
/**
* @see java.sql.DatabaseMetaData#supportsCatalogsInProcedureCalls()
*/
public boolean supportsCatalogsInProcedureCalls() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsCatalogsInTableDefinitions()
*/
public boolean supportsCatalogsInTableDefinitions() throws SQLException
{
return false;
}
public boolean supportsCatalogsInIndexDefinitions() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsCatalogsInPrivilegeDefinitions()
*/
public boolean supportsCatalogsInPrivilegeDefinitions() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsPositionedDelete()
*/
public boolean supportsPositionedDelete() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsPositionedUpdate()
*/
public boolean supportsPositionedUpdate() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsSelectForUpdate()
*/
public boolean supportsSelectForUpdate() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsStoredProcedures()
*/
public boolean supportsStoredProcedures() throws SQLException
{
return false;
}
public boolean supportsSubqueriesInComparisons() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsSubqueriesInExists() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsSubqueriesInIns() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsSubqueriesInQuantifieds() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsCorrelatedSubqueries() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsUnion() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsUnionAll() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsOpenCursorsAcrossCommit() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsOpenCursorsAcrossRollback() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsOpenStatementsAcrossCommit() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsOpenStatementsAcrossRollback() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public int getMaxBinaryLiteralLength() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxCharLiteralLength() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxColumnNameLength() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxColumnsInGroupBy() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxColumnsInIndex() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxColumnsInOrderBy() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxColumnsInSelect() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxColumnsInTable() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
/**
* @see java.sql.DatabaseMetaData#getMaxConnections()
*/
public int getMaxConnections() throws SQLException
{
return 0;
}
public int getMaxCursorNameLength() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxIndexLength() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxSchemaNameLength() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxProcedureNameLength() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxCatalogNameLength() throws SQLException
{
return 0;
}
public int getMaxRowSize() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public boolean doesMaxRowSizeIncludeBlobs() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public int getMaxStatementLength() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
public int getMaxStatements() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
/**
* @see java.sql.DatabaseMetaData#getMaxTableNameLength()
*/
public int getMaxTableNameLength() throws SQLException
{
/*
* The maximum size of a collection name is 128 characters (including the name of the db and indexes).
* It is probably best to keep it under 80/90 chars.
*/
return 90;
}
/**
* @see java.sql.DatabaseMetaData#getMaxTablesInSelect()
*/
public int getMaxTablesInSelect() throws SQLException
{
// MongoDB collections are represented as SQL tables in this driver. Mongo doesn't support joins.
return 1;
}
public int getMaxUserNameLength() throws SQLException
{
// TODO Auto-generated method stub
return 0;
}
/**
* @see java.sql.DatabaseMetaData#getDefaultTransactionIsolation()
*/
public int getDefaultTransactionIsolation() throws SQLException
{
return Connection.TRANSACTION_NONE;
}
/**
* MongoDB doesn't support transactions, but document updates are atomic.
*
* @see java.sql.DatabaseMetaData#supportsTransactions()
*/
public boolean supportsTransactions() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsTransactionIsolationLevel(int)
*/
public boolean supportsTransactionIsolationLevel(int level) throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsDataDefinitionAndDataManipulationTransactions()
*/
public boolean supportsDataDefinitionAndDataManipulationTransactions() throws SQLException
{
return false;
}
public boolean supportsDataManipulationTransactionsOnly() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean dataDefinitionCausesTransactionCommit() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean dataDefinitionIgnoredInTransactions() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
/**
* @see java.sql.DatabaseMetaData#getProcedures(java.lang.String, java.lang.String, java.lang.String)
*/
public ResultSet getProcedures(String catalog, String schemaPattern, String procedureNamePattern)
throws SQLException
{
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "PROCEDURE_CAT", "PROCEDURE_SCHEM", "PROCEDURE_NAME", "REMARKS",
"PROCEDURE_TYPE", "SPECIFIC_NAME" });
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#getProcedureColumns(java.lang.String, java.lang.String, java.lang.String,
* java.lang.String)
*/
public ResultSet getProcedureColumns(String catalog, String schemaPattern, String procedureNamePattern,
String columnNamePattern) throws SQLException
{
return EMPTY_RESULT_SET;
}
/**
* @see java.sql.DatabaseMetaData#getTables(java.lang.String, java.lang.String, java.lang.String,
* java.lang.String[])
*/
public ResultSet getTables(String catalog, String schemaPattern, String tableNamePattern, String[] types)
throws SQLException
{
if (catalog != null)
{
return privateGetTables(catalog, schemaPattern, tableNamePattern, types);
}
ResultSet catalogNameResult = getCatalogs();
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TABLE_CAT", "TABLE_SCHEM", "TABLE_NAME", "TABLE_TYPE", "REMARKS",
"TYPE_CAT", "TYPE_SCHEM", "TYPE_NAME", "SELF_REFERENCING_COL_NAME", "REF_GENERATION" });
while (catalogNameResult.next())
{
String catalogStr = catalogNameResult.getString(1);
ResultSet tableNameResultSet = privateGetTables(catalogStr, schemaPattern, tableNamePattern, types);
retVal.addResultSet((MongoDbResultSet) tableNameResultSet);
}
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#getTables(java.lang.String, java.lang.String, java.lang.String,
* java.lang.String[])
*/
public ResultSet privateGetTables(String catalog, String schemaPattern, String tableNamePattern,
String[] types) throws SQLException
{
System.err.println("Attempting to get tables for catalog: " + catalog);
final DB db = con.getMongoWrapper().getDB(catalog);
Set collectionNames = db.getCollectionNames();
String[][] data = new String[collectionNames.size()][10];
int i = 0;
for (String collectionName : db.getCollectionNames())
{
data[i][0] = catalog; // TABLE_CAT
data[i][1] = ""; // TABLE_SCHEM
data[i][2] = collectionName; // TABLE_NAME
data[i][3] = "TABLE"; // TABLE_TYPE
data[i][4] = ""; // REMARKS
data[i][5] = ""; // TYPE_CAT
data[i][6] = ""; // TYPE_SCHEM
data[i][7] = ""; // TYPE_NAME
data[i][8] = ""; // SELF_REFERENCING_COL_NAME
data[i][9] = ""; // REF_GENERATION
i++;
}
return new MongoDbResultSet(data, new String[] { "TABLE_CAT", "TABLE_SCHEM", "TABLE_NAME",
"TABLE_TYPE", "REMARKS", "TYPE_CAT", "TYPE_SCHEM", "TYPE_NAME", "SELF_REFERENCING_COL_NAME",
"REF_GENERATION" });
}
/**
* @see java.sql.DatabaseMetaData#getSchemas()
*/
public ResultSet getSchemas() throws SQLException
{
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TABLE_SCHEM", "TABLE_CATALOG" });
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#getCatalogs()
*/
public ResultSet getCatalogs() throws SQLException
{
List mongoDbs = con.getMongoWrapper().getDatabaseNames();
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TABLE_CAT" });
for (String mongoDb : mongoDbs)
{
retVal.addRow(new String[] { mongoDb });
}
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#getTableTypes()
*/
public ResultSet getTableTypes() throws SQLException
{
MongoDbResultSet result = new MongoDbResultSet();
result.addRow(new String[] { "COLLECTION" });
return result;
}
/**
* @see java.sql.DatabaseMetaData#getColumns(java.lang.String, java.lang.String, java.lang.String,
* java.lang.String)
*/
public ResultSet getColumns(String catalog, String schemaPattern, String tableNamePattern,
String columnNamePattern) throws SQLException
{
// As far as this driver implementation goes, every "table" in MongoDB is actually a collection, and
// every collection "table" has two columns - "_id" column which is the primary key, and a "document"
// column which is the JSON document corresponding to the "_id". An "_id" value can be specified on
// insert, or it can be omitted, in which case MongoDB generates a unique value.
MongoDbResultSet result = new MongoDbResultSet();
result.setColumnNames(new String[] { "TABLE_CAT", "TABLE_SCHEM", "TABLE_NAME", "COLUMN_NAME",
"DATA_TYPE", "TYPE_NAME", "COLUMN_SIZE", "BUFFER_LENGTH", "DECIMAL_DIGITS", "NUM_PREC_RADIX",
"NULLABLE", "REMARKS", "COLUMN_DEF", "SQL_DATA_TYPE", "SQL_DATETIME_SUB", "CHAR_OCTET_LENGTH",
"ORDINAL_POSITION", "IS_NULLABLE", "SCOPE_CATLOG", "SCOPE_SCHEMA", "SCOPE_TABLE",
"SOURCE_DATA_TYPE", "IS_AUTOINCREMENT" });
result.addRow(new String[] { catalog, // "TABLE_CAT",
null, // "TABLE_SCHEM",
tableNamePattern, // "TABLE_NAME", (i.e. MongoDB Collection Name)
"_id", // "COLUMN_NAME",
"" + Types.VARCHAR, // "DATA_TYPE",
OBJECT_ID_TYPE_NAME, // "TYPE_NAME",
"800", // "COLUMN_SIZE",
"0", // "BUFFER_LENGTH", (not used)
"0", // "DECIMAL_DIGITS",
"10", // "NUM_PREC_RADIX",
"" + columnNoNulls, // "NULLABLE",
"", // "REMARKS",
"", // "COLUMN_DEF",
"0", // "SQL_DATA_TYPE", (not used)
"0", // "SQL_DATETIME_SUB", (not used)
"800", // "CHAR_OCTET_LENGTH",
"1", // "ORDINAL_POSITION",
"NO", // "IS_NULLABLE",
null, // "SCOPE_CATLOG", (not a REF type)
null, // "SCOPE_SCHEMA", (not a REF type)
null, // "SCOPE_TABLE", (not a REF type)
null, // "SOURCE_DATA_TYPE", (not a DISTINCT or REF type)
"NO" // "IS_AUTOINCREMENT" (can be auto-generated, but can also be specified)
});
result.addRow(new String[] { catalog, // "TABLE_CAT",
null, // "TABLE_SCHEM",
tableNamePattern, // "TABLE_NAME", (i.e. MongoDB Collection Name
"document", // "COLUMN_NAME",
"" + Types.VARCHAR, // "DATA_TYPE",
DOCUMENT_TYPE_NAME, // "TYPE_NAME",
"16777216", // "COLUMN_SIZE",
"0", // "BUFFER_LENGTH", (not used)
"0", // "DECIMAL_DIGITS",
"10", // "NUM_PREC_RADIX",
"" + columnNoNulls, // "NULLABLE",
"", // "REMARKS",
"", // "COLUMN_DEF",
"0", // "SQL_DATA_TYPE", (not used)
"0", // "SQL_DATETIME_SUB", (not used)
"16777216", // "CHAR_OCTET_LENGTH",
"2", // "ORDINAL_POSITION",
"NO", // "IS_NULLABLE",
null, // "SCOPE_CATLOG", (not a REF type)
null, // "SCOPE_SCHEMA", (not a REF type)
null, // "SCOPE_TABLE", (not a REF type)
null, // "SOURCE_DATA_TYPE", (not a DISTINCT or REF type)
"NO" // "IS_AUTOINCREMENT" (can be auto-generated, but can also be specified)
});
return result;
}
public ResultSet getColumnPrivileges(String catalog, String schema, String table, String columnNamePattern)
throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public ResultSet getTablePrivileges(String catalog, String schemaPattern, String tableNamePattern)
throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public ResultSet getBestRowIdentifier(String catalog, String schema, String table, int scope,
boolean nullable) throws SQLException
{
// TODO Auto-generated method stub
return null;
}
/**
* @see java.sql.DatabaseMetaData#getVersionColumns(java.lang.String, java.lang.String, java.lang.String)
*/
public ResultSet getVersionColumns(String catalog, String schema, String table) throws SQLException
{
return EMPTY_RESULT_SET;
}
/**
* @see java.sql.DatabaseMetaData#getPrimaryKeys(java.lang.String, java.lang.String, java.lang.String)
*/
public ResultSet getPrimaryKeys(String catalog, String schema, String table) throws SQLException
{
/*
* TABLE_CAT String => table catalog (may be null
)
* TABLE_SCHEM String => table schema (may be null
)
* TABLE_NAME String => table name
* COLUMN_NAME String => column name
* KEY_SEQ short => sequence number within primary key( a value
* of 1 represents the first column of the primary key, a value of 2 would
* represent the second column within the primary key).
* PK_NAME Stri
*
*/
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TABLE_CAT", "TABLE_SCHEM", "TABLE_NAME", "COLUMN_NAME",
"KEY_SEQ", "PK_NAME" });
retVal.addRow(new String[] { catalog, schema, table, "_id", "1", table + "-pk" });
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#getImportedKeys(java.lang.String, java.lang.String, java.lang.String)
*/
public ResultSet getImportedKeys(String catalog, String schema, String table) throws SQLException
{
return EMPTY_RESULT_SET;
}
/**
* @see java.sql.DatabaseMetaData#getExportedKeys(java.lang.String, java.lang.String, java.lang.String)
*/
public ResultSet getExportedKeys(String catalog, String schema, String table) throws SQLException
{
return EMPTY_RESULT_SET;
}
/**
* @see java.sql.DatabaseMetaData#getCrossReference(java.lang.String, java.lang.String, java.lang.String,
* java.lang.String, java.lang.String, java.lang.String)
*/
public ResultSet getCrossReference(String parentCatalog, String parentSchema, String parentTable,
String foreignCatalog, String foreignSchema, String foreignTable) throws SQLException
{
return EMPTY_RESULT_SET;
}
/**
* @see java.sql.DatabaseMetaData#getTypeInfo()
*/
public ResultSet getTypeInfo() throws SQLException
{
/*
* Each type description has the following columns:
*
* - TYPE_NAME String => Type name
*
- DATA_TYPE int => SQL data type from java.sql.Types
*
- PRECISION int => maximum precision
*
- LITERAL_PREFIX String => prefix used to quote a literal
* (may be
null
)
* - LITERAL_SUFFIX String => suffix used to quote a literal
(may be
null
)
* - CREATE_PARAMS String => parameters used in creating
* the type (may be
null
)
* - NULLABLE short => can you use NULL for this type.
*
* - typeNoNulls - does not allow NULL values
*
- typeNullable - allows NULL values
*
- typeNullableUnknown - nullability unknown
*
* - CASE_SENSITIVE boolean=> is it case sensitive.
*
- SEARCHABLE short => can you use "WHERE" based on this type:
*
* - typePredNone - No support
*
- typePredChar - Only supported with WHERE .. LIKE
*
- typePredBasic - Supported except for WHERE .. LIKE
*
- typeSearchable - Supported for all WHERE ..
*
* - UNSIGNED_ATTRIBUTE boolean => is it unsigned.
*
- FIXED_PREC_SCALE boolean => can it be a money value.
*
- AUTO_INCREMENT boolean => can it be used for an
* auto-increment value.
*
- LOCAL_TYPE_NAME String => localized version of type name
* (may be
null
)
* - MINIMUM_SCALE short => minimum scale supported
*
- MAXIMUM_SCALE short => maximum scale supported
*
- SQL_DATA_TYPE int => unused
*
- SQL_DATETIME_SUB int => unused
*
- NUM_PREC_RADIX int => usually 2 or 10
*
*/
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TYPE_NAME", "DATA_TYPE", "PRECISION", "LITERAL_PREFIX",
"LITERAL_SUFFIX", "CREATE_PARAMS", "NULLABLE", "CASE_SENSITIVE", "SEARCHABLE",
"UNSIGNED_ATTRIBUTE", "FIXED_PREC_SCALE", "AUTO_INCREMENT", "LOCAL_TYPE_NAME", "MINIMUM_SCALE",
"MAXIMUM_SCALE", "SQL_DATA_TYPE", "SQL_DATETIME_SUB", "NUM_PREC_RADIX" });
retVal.addRow(new String[] { OBJECT_ID_TYPE_NAME, // "TYPE_NAME",
"" + Types.VARCHAR, // "DATA_TYPE",
"800", // "PRECISION",
"'", // "LITERAL_PREFIX",
"'", // "LITERAL_SUFFIX",
null, // "CREATE_PARAMS",
"" + typeNullable, // "NULLABLE",
"true", // "CASE_SENSITIVE",
"" + typeSearchable, // "SEARCHABLE",
"false", // "UNSIGNED_ATTRIBUTE",
"false", // "FIXED_PREC_SCALE",
"false", // "AUTO_INCREMENT",
OBJECT_ID_TYPE_NAME, // "LOCAL_TYPE_NAME",
"0", // "MINIMUM_SCALE",
"0", // "MAXIMUM_SCALE",
null, // "SQL_DATA_TYPE", (not used)
null, // "SQL_DATETIME_SUB", (not used)
"10", // "NUM_PREC_RADIX" (javadoc says usually 2 or 10)
});
retVal.addRow(new String[] { DOCUMENT_TYPE_NAME, // "TYPE_NAME",
"" + Types.CLOB, // "DATA_TYPE",
"16777216", // "PRECISION",
"'", // "LITERAL_PREFIX",
"'", // "LITERAL_SUFFIX",
null, // "CREATE_PARAMS",
"" + typeNullable, // "NULLABLE",
"true", // "CASE_SENSITIVE",
"" + typeSearchable, // "SEARCHABLE",
"false", // "UNSIGNED_ATTRIBUTE",
"false", // "FIXED_PREC_SCALE",
"false", // "AUTO_INCREMENT",
DOCUMENT_TYPE_NAME, // "LOCAL_TYPE_NAME",
"0", // "MINIMUM_SCALE",
"0", // "MAXIMUM_SCALE",
null, // "SQL_DATA_TYPE", (not used)
null, // "SQL_DATETIME_SUB", (not used)
"10", // "NUM_PREC_RADIX" (javadoc says usually 2 or 10)
});
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#getIndexInfo(java.lang.String, java.lang.String, java.lang.String,
* boolean, boolean)
*/
public ResultSet getIndexInfo(String catalog, String schema, String table, boolean unique,
boolean approximate) throws SQLException
{
/*
* *
* - TABLE_CAT String => table catalog (may be
null
)
* - TABLE_SCHEM String => table schema (may be
null
)
* - TABLE_NAME String => table name
*
- NON_UNIQUE boolean => Can index values be non-unique.
* false when TYPE is tableIndexStatistic
*
- INDEX_QUALIFIER String => index catalog (may be
null
);
* null
when TYPE is tableIndexStatistic
* - INDEX_NAME String => index name;
null
when TYPE is
* tableIndexStatistic
* - TYPE short => index type:
*
* - tableIndexStatistic - this identifies table statistics that are
* returned in conjuction with a table's index descriptions
*
- tableIndexClustered - this is a clustered index
*
- tableIndexHashed - this is a hashed index
*
- tableIndexOther - this is some other style of index
*
* - ORDINAL_POSITION short => column sequence number
* within index; zero when TYPE is tableIndexStatistic
*
- COLUMN_NAME String => column name;
null
when TYPE is
* tableIndexStatistic
* - ASC_OR_DESC String => column sort sequence, "A" => ascending,
* "D" => descending, may be
null
if sort sequence is not supported;
* null
when TYPE is tableIndexStatistic
* - CARDINALITY int => When TYPE is tableIndexStatistic, then
* this is the number of rows in the table; otherwise, it is the
* number of unique values in the index.
*
- PAGES int => When TYPE is tableIndexStatisic then
* this is the number of pages used for the table, otherwise it
* is the number of pages used for the current index.
*
- FILTER_CONDITION String => Filter condition, if any.
* (may be
null
)
*
*/
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TABLE_CAT", "TABLE_SCHEM", "TABLE_NAME", "NON_UNIQUE",
"INDEX_QUALIFIER", "INDEX_NAME", "TYPE", "ORDINAL_POSITION", "COLUMN_NAME", "ASC_OR_DESC",
"CARDINALITY", "PAGES", "FILTER_CONDITION" });
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#supportsResultSetType(int)
*/
public boolean supportsResultSetType(int type) throws SQLException
{
if (type == ResultSet.TYPE_FORWARD_ONLY)
{
return true;
}
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsResultSetConcurrency(int, int)
*/
public boolean supportsResultSetConcurrency(int type, int concurrency) throws SQLException
{
return false;
}
public boolean ownUpdatesAreVisible(int type) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean ownDeletesAreVisible(int type) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean ownInsertsAreVisible(int type) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean othersUpdatesAreVisible(int type) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean othersDeletesAreVisible(int type) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean othersInsertsAreVisible(int type) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean updatesAreDetected(int type) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean deletesAreDetected(int type) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean insertsAreDetected(int type) throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public boolean supportsBatchUpdates() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
/**
* @see java.sql.DatabaseMetaData#getUDTs(java.lang.String, java.lang.String, java.lang.String, int[])
*/
public ResultSet getUDTs(String catalog, String schemaPattern, String typeNamePattern, int[] types)
throws SQLException
{
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TYPE_CAT", "TYPE_SCHEM", "TYPE_NAME", "CLASS_NAME", "DATA_TYPE",
"REMARKS", "BASE_TYPE", });
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#getConnection()
*/
public Connection getConnection() throws SQLException
{
return con;
}
/**
* @see java.sql.DatabaseMetaData#supportsSavepoints()
*/
public boolean supportsSavepoints() throws SQLException
{
return false;
}
public boolean supportsNamedParameters() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsMultipleOpenResults()
*/
public boolean supportsMultipleOpenResults() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsGetGeneratedKeys()
*/
public boolean supportsGetGeneratedKeys() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#getSuperTypes(java.lang.String, java.lang.String, java.lang.String)
*/
public ResultSet getSuperTypes(String catalog, String schemaPattern, String typeNamePattern)
throws SQLException
{
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TYPE_CAT", "TYPE_SCHEM", "TYPE_NAME", "SUPERTYPE_CAT",
"SUPERTYPE_SCHEM", "SUPERTYPE_NAME" });
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#getSuperTables(java.lang.String, java.lang.String, java.lang.String)
*/
public ResultSet getSuperTables(String catalog, String schemaPattern, String tableNamePattern)
throws SQLException
{
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TABLE_CAT", "TABLE_SCHEM", "TABLE_NAME", "SUPERTABLE_NAME" });
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#getAttributes(java.lang.String, java.lang.String, java.lang.String,
* java.lang.String)
*/
public ResultSet getAttributes(String catalog, String schemaPattern, String typeNamePattern,
String attributeNamePattern) throws SQLException
{
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TYPE_CAT", "TYPE_SCHEM", "TYPE_NAME", "ATTR_NAME", "DATA_TYPE",
"ATTR_TYPE_NAME", "ATTR_SIZE", "DECIMAL_DIGITS", "NUM_PREC_RADIX", "NULLABLE", "REMARKS",
"ATTR_DEF", "SQL_DATA_TYPE", "SQL_DATETIME_SUB", "CHAR_OCTET_LENGTH", "ORDINAL_POSITION",
"IS_NULLABLE", "SCOPE_CATALOG", "SCOPE_SCHEMA", "SCOPE_TABLE", "SOURCE_DATA_TYPE" });
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#supportsResultSetHoldability(int)
*/
public boolean supportsResultSetHoldability(int holdability) throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#getResultSetHoldability()
*/
public int getResultSetHoldability() throws SQLException
{
return ResultSet.HOLD_CURSORS_OVER_COMMIT;
}
/**
* @see java.sql.DatabaseMetaData#getDatabaseMajorVersion()
*/
public int getDatabaseMajorVersion() throws SQLException
{
return Integer.parseInt((getDatabaseProductVersion().split("\\."))[0]);
}
/**
* @see java.sql.DatabaseMetaData#getDatabaseMinorVersion()
*/
public int getDatabaseMinorVersion() throws SQLException
{
return Integer.parseInt((getDatabaseProductVersion().split("\\."))[1]);
}
/**
* @see java.sql.DatabaseMetaData#getJDBCMajorVersion()
*/
public int getJDBCMajorVersion() throws SQLException
{
return 1;
}
/**
* @see java.sql.DatabaseMetaData#getJDBCMinorVersion()
*/
public int getJDBCMinorVersion() throws SQLException
{
return 0;
}
/**
* @see java.sql.DatabaseMetaData#getSQLStateType()
*/
public int getSQLStateType() throws SQLException
{
return DatabaseMetaData.sqlStateXOpen;
}
public boolean locatorsUpdateCopy() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
/**
* @see java.sql.DatabaseMetaData#supportsStatementPooling()
*/
public boolean supportsStatementPooling() throws SQLException
{
return false;
}
/**
* @see java.sql.DatabaseMetaData#getRowIdLifetime()
*/
public RowIdLifetime getRowIdLifetime() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
/**
* @see java.sql.DatabaseMetaData#getSchemas(java.lang.String, java.lang.String)
*/
public ResultSet getSchemas(String catalog, String schemaPattern) throws SQLException
{
MongoDbResultSet retVal = new MongoDbResultSet();
retVal.setColumnNames(new String[] { "TABLE_SCHEM", "TABLE_CATALOG" });
return retVal;
}
/**
* @see java.sql.DatabaseMetaData#supportsStoredFunctionsUsingCallSyntax()
*/
public boolean supportsStoredFunctionsUsingCallSyntax() throws SQLException
{
return false;
}
public boolean autoCommitFailureClosesAllResultSets() throws SQLException
{
// TODO Auto-generated method stub
return false;
}
public ResultSet getClientInfoProperties() throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public ResultSet getFunctions(String catalog, String schemaPattern, String functionNamePattern)
throws SQLException
{
// TODO Auto-generated method stub
return null;
}
public ResultSet getFunctionColumns(String catalog, String schemaPattern, String functionNamePattern,
String columnNamePattern) throws SQLException
{
// TODO Auto-generated method stub
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy