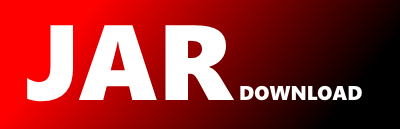
net.sf.mongodb_jdbc_driver.ParserHelper Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 Rob Manning
* [email protected]
*
* This file is part of MongoDB JDBC Driver.
*
* MongoDB JDBC Driver is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* MongoDB JDBC Driver is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with MongoDB JDBC Driver. If not, see .
*/
package net.sf.mongodb_jdbc_driver;
import java.io.ByteArrayInputStream;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
import java.util.Vector;
import net.sf.mongodb_jdbc_driver.zql.ParseException;
import net.sf.mongodb_jdbc_driver.zql.ZDelete;
import net.sf.mongodb_jdbc_driver.zql.ZFromItem;
import net.sf.mongodb_jdbc_driver.zql.ZInsert;
import net.sf.mongodb_jdbc_driver.zql.ZQuery;
import net.sf.mongodb_jdbc_driver.zql.ZSelectItem;
import net.sf.mongodb_jdbc_driver.zql.ZUpdate;
import net.sf.mongodb_jdbc_driver.zql.ZqlParser;
public class ParserHelper
{
private final ZqlParser zqlParser = new ZqlParser();
public boolean isQueryWithJoin(String sql) throws ParseException
{
initParser(sql);
ZQuery q = (ZQuery)zqlParser.readStatement();
Vector v = q.getFrom();
return (v.size() > 1);
}
public boolean isSelectQuery(String sql) throws ParseException
{
initParser(sql);
return (zqlParser.readStatement() instanceof ZQuery);
}
public boolean isUpdateQuery(String sql) throws ParseException {
initParser(sql);
return (zqlParser.readStatement() instanceof ZUpdate);
}
public boolean isInsertQuery(String sql) throws ParseException {
initParser(sql);
return (zqlParser.readStatement() instanceof ZInsert);
}
public boolean isDeleteQuery(String sql) throws ParseException {
initParser(sql);
return (zqlParser.readStatement() instanceof ZDelete);
}
public String getSelectTable(String sql) throws ParseException, SQLException
{
initParser(sql);
ZQuery q = (ZQuery)zqlParser.readStatement();
Vector v = q.getFrom();
if (v.size() > 1) {
throw new SQLException("MongoDB does not support queries with joins");
}
if (v.size() < 1) {
throw new SQLException("The specified query ("+sql+") does not contain a from clause");
}
return ((ZFromItem) v.get(0)).getTable();
}
public List getSelectColumns(String sql) throws ParseException, SQLException {
ArrayList result = new ArrayList();
initParser(sql);
ZQuery q = (ZQuery)zqlParser.readStatement();
Vector v = q.getSelect();
Enumeration e = v.elements();
while (e.hasMoreElements()) {
ZSelectItem selectElement = e.nextElement();
if (selectElement.getAggregate() != null) {
result.add(selectElement.getAggregate().toLowerCase() + "(" + selectElement.getColumn() + ")");
} else {
result.add(selectElement.getColumn().toLowerCase());
}
}
return result;
}
private void initParser(String sql) {
if (sql.endsWith(";")) {
zqlParser.initParser(new ByteArrayInputStream(sql.getBytes()));
} else {
zqlParser.initParser(new ByteArrayInputStream((sql + ";").getBytes()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy