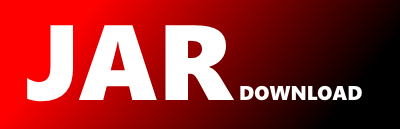
net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 Rob Manning
* [email protected]
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package net.sf.mongodb_jdbc_driver.wrapper;
import java.util.Collection;
import java.util.List;
import com.mongodb.CommandResult;
import com.mongodb.DB;
import com.mongodb.DBObject;
import com.mongodb.DBTCPConnector;
import com.mongodb.MongoOptions;
import com.mongodb.ReadPreference;
import com.mongodb.ReplicaSetStatus;
import com.mongodb.ServerAddress;
import com.mongodb.WriteConcern;
public interface MongoWrapper
{
/**
* gets a database object
*
* @param dbname
* the database name
* @return
*/
DB getDB(String dbname);
/**
* gets a collection of DBs used by the driver since this Mongo instance was created. This may include DBs
* that exist in the client but not yet on the server.
*
* @return
*/
Collection getUsedDatabases();
/**
* gets a list of all database names present on the server
*
* @return
* @throws MongoException
*/
List getDatabaseNames();
/**
* Drops the database if it exists.
*
* @param dbName
* name of database to drop
* @throws MongoException
*/
void dropDatabase(String dbName);
/**
* gets this driver version
*
* @return the full version string of this driver, e.g. "2.8.0"
*/
String getVersion();
/**
* returns a string representing the hosts used in this Mongo instance
*
* @return
*/
String debugString();
/**
* Gets the current master's hostname
*
* @return
*/
String getConnectPoint();
/**
* Gets the underlying TCP connector
*
* @return
*/
DBTCPConnector getConnector();
/**
* Gets the replica set status object
*
* @return
*/
ReplicaSetStatus getReplicaSetStatus();
/**
* Gets the address of the current master
*
* @return the address
*/
ServerAddress getAddress();
/**
* Gets a list of all server addresses used when this Mongo was created
*
* @return
*/
List getAllAddress();
/**
* Gets the list of server addresses currently seen by the connector. This includes addresses
* auto-discovered from a replica set.
*
* @return
* @throws MongoException
*/
List getServerAddressList();
/**
* closes the underlying connector, which in turn closes all open connections. Once called, this Mongo
* instance can no longer be used.
*/
void close();
/**
* Sets the write concern for this database. Will be used as default for writes to any collection in any
* database. See the documentation for {@link WriteConcern} for more information.
*
* @param concern
* write concern to use
*/
void setWriteConcern(WriteConcern concern);
/**
* Gets the default write concern
*
* @return
*/
WriteConcern getWriteConcern();
/**
* Sets the read preference for this database. Will be used as default for reads from any collection in any
* database. See the documentation for {@link ReadPreference} for more information.
*
* @param preference
* Read Preference to use
*/
void setReadPreference(ReadPreference preference);
/**
* Gets the default read preference
*
* @return
*/
ReadPreference getReadPreference();
/**
* makes it possible to run read queries on secondary nodes
*
* @deprecated Replaced with {@code ReadPreference.secondaryPreferred()}
* @see ReadPreference#secondaryPreferred()
*/
@Deprecated
void slaveOk();
/**
* adds a default query option
*
* @param option
*/
void addOption(int option);
/**
* sets the default query options
*
* @param options
*/
void setOptions(int options);
/**
* reset the default query options
*/
void resetOptions();
/**
* gets the default query options
*
* @return
*/
int getOptions();
/**
* Returns the mongo options.
*/
MongoOptions getMongoOptions();
/**
* Gets the maximum size for a BSON object supported by the current master server. Note that this value may
* change over time depending on which server is master. If the size is not known yet, a request may be
* sent to the master server
*
* @return the maximum size
* @throws MongoException
*/
int getMaxBsonObjectSize();
/**
* Forces the master server to fsync the RAM data to disk This is done automatically by the server at
* intervals, but can be forced for better reliability.
*
* @param async
* if true, the fsync will be done asynchronously on the server.
* @return
* @throws MongoException
*/
CommandResult fsync(boolean async);
/**
* Forces the master server to fsync the RAM data to disk, then lock all writes. The database will be
* read-only after this command returns.
*
* @return
* @throws MongoException
*/
CommandResult fsyncAndLock();
/**
* Unlocks the database, allowing the write operations to go through. This command may be asynchronous on
* the server, which means there may be a small delay before the database becomes writable.
*
* @return
* @throws MongoException
*/
DBObject unlock();
/**
* Returns true if the database is locked (read-only), false otherwise.
*
* @return
* @throws MongoException
*/
boolean isLocked();
/**
* @return string representation of the wrapped mongo instance.
*/
String toString();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy