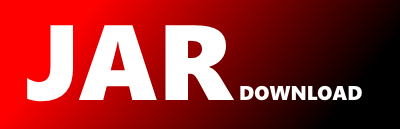
net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactory Maven / Gradle / Ivy
/*
* Copyright (C) 2012 Rob Manning
* [email protected]
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package net.sf.mongodb_jdbc_driver.wrapper;
import java.net.UnknownHostException;
import java.util.List;
import java.util.Properties;
import com.mongodb.Mongo;
import com.mongodb.MongoException;
import com.mongodb.MongoOptions;
import com.mongodb.MongoURI;
import com.mongodb.ServerAddress;
public interface MongoWrapperFactory
{
public abstract MongoWrapper getMongoWrapper(String server, int port, Properties connectionProperties)
throws UnknownHostException;
/**
* Already have a Mongo instance somehow? Wrap it up.
*
* @param mongo
* the Mongo instance to wrap.
* @return
*/
public abstract MongoWrapper getMongoWrapper(Mongo mongo);
/**
* Creates a Mongo instance based on a (single) mongodb node (localhost, default port)
*
* @throws UnknownHostException
* @throws MongoException
*/
public abstract MongoWrapper getMongoWrapper() throws UnknownHostException;
/**
* Creates a Mongo instance based on a (single) mongodb node (default port)
*
* @param host
* server to connect to
* @throws UnknownHostException
* if the database host cannot be resolved
* @throws MongoException
*/
public abstract MongoWrapper getMongoWrapper(String host) throws UnknownHostException;
/**
* Creates a Mongo instance based on a (single) mongo node using a given ServerAddress
*
* @see com.mongodb.ServerAddress
* @param addr
* the database address
* @param options
* default query options
* @throws MongoException
*/
public abstract MongoWrapper getMongoWrapper(ServerAddress addr, MongoOptions options);
/**
* Creates a Mongo based on a list of replica set members or a list of mongos. It will find all members
* (the master will be used by default). If you pass in a single server in the list, the driver will still
* function as if it is a replica set. If you have a standalone server, use the Mongo(ServerAddress)
* constructor.
*
* If this is a list of mongos servers, it will pick the closest (lowest ping time) one to send all
* requests to, and automatically fail over to the next server if the closest is down.
*
* @see com.mongodb.ServerAddress
* @param seeds
* Put as many servers as you can in the list and the system will figure out the rest. This can
* either be a list of mongod servers in the same replica set or a list of mongos servers in the
* same sharded cluster.
* @param options
* for configuring this Mongo instance
* @throws MongoException
*/
public abstract MongoWrapper getMongoWrapper(List seeds, MongoOptions options);
/**
* Creates a Mongo described by a URI. If only one address is used it will only connect to that node,
* otherwise it will discover all nodes.
*
* @param uri
* @see MongoURI
* examples:
*
mongodb://localhost
* mongodb://fred:foobar@localhost/
*
* @throws MongoException
* @throws UnknownHostException
* @dochub connections
*/
public abstract MongoWrapper getMongoWrapper(MongoURI uri) throws UnknownHostException;
}