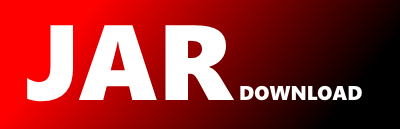
net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactoryImpl Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 Rob Manning
* [email protected]
*
* This file is part of MongoDB JDBC Driver.
*
* MongoDB JDBC Driver is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* MongoDB JDBC Driver is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with MongoDB JDBC Driver. If not, see .
*/
package net.sf.mongodb_jdbc_driver.wrapper;
import java.net.UnknownHostException;
import java.util.List;
import java.util.Properties;
import net.sf.mongodb_jdbc_driver.DriverPropertyInfoHelper;
import com.mongodb.Mongo;
import com.mongodb.MongoOptions;
import com.mongodb.MongoURI;
import com.mongodb.ServerAddress;
/**
* Implementation of MongoWrapperFactory.
*/
public class MongoWrapperFactoryImpl implements MongoWrapperFactory
{
private DriverPropertyInfoHelper propInfoHelper = new DriverPropertyInfoHelper();
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactory#getMongoWrapper(java.lang.String, int,
* java.util.Properties)
*/
@Override
public MongoWrapper getMongoWrapper(String server, int port, Properties connectionProperties)
throws UnknownHostException
{
// TODO: We will need to define properties that tell us things such as read preference, write concern,
// replica set addresses, write timeout, connect timeout, etc.. See MongoOptions and the various
// constructors for Mongo.
MongoOptions mongoOptions = propInfoHelper.getMongoOptions(connectionProperties);
ServerAddress addr = new ServerAddress(server, port);
return new MongoWrapperImpl(addr, mongoOptions);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactory#getMongoWrapper(com.mongodb.Mongo)
*/
@Override
public MongoWrapper getMongoWrapper(Mongo mongo)
{
return new MongoWrapperImpl(mongo);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactory#getMongoWrapper()
*/
@Override
public MongoWrapper getMongoWrapper() throws UnknownHostException
{
return new MongoWrapperImpl();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactory#getMongoWrapper(java.lang.String)
*/
@Override
public MongoWrapper getMongoWrapper(String host) throws UnknownHostException
{
return new MongoWrapperImpl(host);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactory#getMongoWrapper(com.mongodb.ServerAddress,
* com.mongodb.MongoOptions)
*/
@Override
public MongoWrapper getMongoWrapper(ServerAddress addr, MongoOptions options)
{
if (options == null)
{
return new MongoWrapperImpl(addr);
}
return new MongoWrapperImpl(addr, options);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactory#getMongoWrapper(java.util.List,
* com.mongodb.MongoOptions)
*/
@Override
public MongoWrapper getMongoWrapper(List seeds, MongoOptions options)
{
if (options == null)
{
return new MongoWrapperImpl(seeds);
}
return new MongoWrapperImpl(seeds, options);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperFactory#getMongoWrapper(com.mongodb.MongoURI)
*/
@Override
public MongoWrapper getMongoWrapper(MongoURI uri) throws UnknownHostException
{
return new MongoWrapperImpl(uri);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy