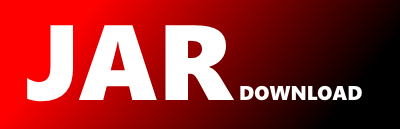
net.sf.mongodb_jdbc_driver.wrapper.MongoWrapperImpl Maven / Gradle / Ivy
/*
* Copyright (C) 2012 Rob Manning
* [email protected]
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package net.sf.mongodb_jdbc_driver.wrapper;
import java.net.UnknownHostException;
import java.util.Collection;
import java.util.List;
import com.mongodb.Bytes;
import com.mongodb.CommandResult;
import com.mongodb.DB;
import com.mongodb.DBAddress;
import com.mongodb.DBObject;
import com.mongodb.DBTCPConnector;
import com.mongodb.Mongo;
import com.mongodb.MongoException;
import com.mongodb.MongoOptions;
import com.mongodb.MongoURI;
import com.mongodb.ReadPreference;
import com.mongodb.ReplicaSetStatus;
import com.mongodb.ServerAddress;
import com.mongodb.WriteConcern;
/**
* A database connection with internal connection pooling. For most applications, you should have one Mongo
* instance for the entire JVM.
*
* The following are equivalent, and all connect to the local database running on the default port:
*
*
* Mongo mongo1 = new Mongo();
*
* Mongo mongo1 = new Mongo("localhost");
*
* Mongo mongo2 = new Mongo("localhost", 27017);
*
* Mongo mongo4 = new Mongo(new ServerAddress("localhost"));
*
*
* You can connect to a replica set using the
* Java driver by passing a ServerAddress list to the Mongo constructor. For example:
*
*
* Mongo mongo = new Mongo(Arrays.asList(new ServerAddress("localhost", 27017), new ServerAddress("localhost",
* 27018), new ServerAddress("localhost", 27019)));
*
*
* You can connect to a sharded cluster using the same constructor. Mongo will auto-detect whether the servers
* are a list of replica set members or a list of mongos servers.
*
* By default, all read and write operations will be made on the primary, but it's possible to read from
* secondaries by changing the read preference:
*
*
*
* mongo.setReadPreference(ReadPreference.secondary());
*
*
* By default, write operations will not throw exceptions on failure, but that is easily changed too:
*
*
*
* mongo.setWriteConcern(WriteConcern.SAFE);
*
*
* @see com.mongodb.ReadPreference
* @see com.mongodb.WriteConcern
*/
public class MongoWrapperImpl implements MongoWrapper
{
/** The wrapped instance of Mongo */
private final Mongo mongo;
/**
* Gets the major version of this library
*
* @return the major version, e.g. 2
*/
public static int getMajorVersion()
{
return Mongo.getMajorVersion();
}
/**
* Gets the minor version of this library
*
* @return the minor version, e.g. 8
*/
public static int getMinorVersion()
{
return Mongo.getMajorVersion();
}
/**
* returns a database object
*
* @param addr
* the database address
* @return
* @throws MongoException
*/
public static DB connect(DBAddress addr)
{
return new MongoWrapperImpl(addr).getDB(addr.getDBName());
}
/**
* @param mongo the instance of Mongo to wrap
*/
public MongoWrapperImpl(Mongo mongo)
{
this.mongo = mongo;
}
/**
* Creates a Mongo instance based on a (single) mongodb node (localhost, default port)
*
* @throws UnknownHostException
* @throws MongoException
*/
public MongoWrapperImpl() throws UnknownHostException
{
mongo = new Mongo();
}
/**
* Creates a Mongo instance based on a (single) mongodb node (default port)
*
* @param host
* server to connect to
* @throws UnknownHostException
* if the database host cannot be resolved
* @throws MongoException
*/
public MongoWrapperImpl(String host) throws UnknownHostException
{
mongo = new Mongo(host);
}
/**
* Creates a Mongo instance based on a (single) mongodb node (default port)
*
* @param host
* server to connect to
* @param options
* default query options
* @throws UnknownHostException
* if the database host cannot be resolved
* @throws MongoException
*/
public MongoWrapperImpl(String host, MongoOptions options) throws UnknownHostException
{
mongo = new Mongo(host, options);
}
/**
* Creates a Mongo instance based on a (single) mongodb node
*
* @param host
* the database's host address
* @param port
* the port on which the database is running
* @throws UnknownHostException
* if the database host cannot be resolved
* @throws MongoException
*/
public MongoWrapperImpl(String host, int port) throws UnknownHostException
{
mongo = new Mongo(host, port);
}
/**
* Creates a Mongo instance based on a (single) mongodb node
*
* @see com.mongodb.ServerAddress
* @param addr
* the database address
* @throws MongoException
*/
public MongoWrapperImpl(ServerAddress addr)
{
mongo = new Mongo(addr);
}
/**
* Creates a Mongo instance based on a (single) mongo node using a given ServerAddress
*
* @see com.mongodb.ServerAddress
* @param addr
* the database address
* @param options
* default query options
* @throws MongoException
*/
public MongoWrapperImpl(ServerAddress addr, MongoOptions options)
{
mongo = new Mongo(addr, options);
}
/**
* Creates a Mongo based on a list of replica set members or a list of mongos. It will find all members
* (the master will be used by default). If you pass in a single server in the list, the driver will still
* function as if it is a replica set. If you have a standalone server, use the Mongo(ServerAddress)
* constructor.
*
* If this is a list of mongos servers, it will pick the closest (lowest ping time) one to send all
* requests to, and automatically fail over to the next server if the closest is down.
*
* @see com.mongodb.ServerAddress
* @param seeds
* Put as many servers as you can in the list and the system will figure out the rest. This can
* either be a list of mongod servers in the same replica set or a list of mongos servers in the
* same sharded cluster.
* @throws MongoException
*/
public MongoWrapperImpl(List seeds)
{
mongo = new Mongo(seeds);
}
/**
* Creates a Mongo based on a list of replica set members or a list of mongos. It will find all members
* (the master will be used by default). If you pass in a single server in the list, the driver will still
* function as if it is a replica set. If you have a standalone server, use the Mongo(ServerAddress)
* constructor.
*
* If this is a list of mongos servers, it will pick the closest (lowest ping time) one to send all
* requests to, and automatically fail over to the next server if the closest is down.
*
* @see com.mongodb.ServerAddress
* @param seeds
* Put as many servers as you can in the list and the system will figure out the rest. This can
* either be a list of mongod servers in the same replica set or a list of mongos servers in the
* same sharded cluster.
* @param options
* for configuring this Mongo instance
* @throws MongoException
*/
public MongoWrapperImpl(List seeds, MongoOptions options)
{
mongo = new Mongo(seeds, options);
}
/**
* Creates a Mongo described by a URI. If only one address is used it will only connect to that node,
* otherwise it will discover all nodes.
*
* @param uri
* @see MongoURI
* examples:
*
mongodb://localhost
* mongodb://fred:foobar@localhost/
*
* @throws MongoException
* @throws UnknownHostException
* @dochub connections
*/
public MongoWrapperImpl(MongoURI uri) throws UnknownHostException
{
mongo = new Mongo(uri);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getDB(java.lang.String)
*/
@Override
public DB getDB(String dbname)
{
return mongo.getDB(dbname);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getUsedDatabases()
*/
@Override
public Collection getUsedDatabases()
{
return mongo.getUsedDatabases();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getDatabaseNames()
*/
@Override
public List getDatabaseNames()
{
return mongo.getDatabaseNames();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#dropDatabase(java.lang.String)
*/
@Override
public void dropDatabase(String dbName)
{
mongo.dropDatabase(dbName);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getVersion()
*/
@Override
public String getVersion()
{
return mongo.getVersion();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#debugString()
*/
@Override
public String debugString()
{
return mongo.debugString();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getConnectPoint()
*/
@Override
public String getConnectPoint()
{
return mongo.getConnectPoint();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getConnector()
*/
@Override
public DBTCPConnector getConnector()
{
return mongo.getConnector();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getReplicaSetStatus()
*/
@Override
public ReplicaSetStatus getReplicaSetStatus()
{
return mongo.getReplicaSetStatus();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getAddress()
*/
@Override
public ServerAddress getAddress()
{
return mongo.getAddress();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getAllAddress()
*/
@Override
public List getAllAddress()
{
return mongo.getAllAddress();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getServerAddressList()
*/
@Override
public List getServerAddressList()
{
return mongo.getServerAddressList();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#close()
*/
@Override
public void close()
{
mongo.close();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#setWriteConcern(com.mongodb.WriteConcern)
*/
@Override
public void setWriteConcern(WriteConcern concern)
{
mongo.setWriteConcern(concern);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getWriteConcern()
*/
@Override
public WriteConcern getWriteConcern()
{
return mongo.getWriteConcern();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#setReadPreference(com.mongodb.ReadPreference)
*/
@Override
public void setReadPreference(ReadPreference preference)
{
mongo.setReadPreference(preference);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getReadPreference()
*/
@Override
public ReadPreference getReadPreference()
{
return mongo.getReadPreference();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#slaveOk()
*/
@Override
@Deprecated
public void slaveOk()
{
addOption(Bytes.QUERYOPTION_SLAVEOK);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#addOption(int)
*/
@Override
public void addOption(int option)
{
mongo.addOption(option);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#setOptions(int)
*/
@Override
public void setOptions(int options)
{
mongo.setOptions(options);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#resetOptions()
*/
@Override
public void resetOptions()
{
mongo.resetOptions();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getOptions()
*/
@Override
public int getOptions()
{
return mongo.getOptions();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getMongoOptions()
*/
@Override
public MongoOptions getMongoOptions()
{
return mongo.getMongoOptions();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#getMaxBsonObjectSize()
*/
@Override
public int getMaxBsonObjectSize()
{
return mongo.getMaxBsonObjectSize();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#fsync(boolean)
*/
@Override
public CommandResult fsync(boolean async)
{
return mongo.fsync(async);
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#fsyncAndLock()
*/
@Override
public CommandResult fsyncAndLock()
{
return mongo.fsyncAndLock();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#unlock()
*/
@Override
public DBObject unlock()
{
return mongo.unlock();
}
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#isLocked()
*/
@Override
public boolean isLocked()
{
return mongo.isLocked();
}
// -------
/**
* @see net.sf.mongodb_jdbc_driver.wrapper.MongoWrapper#toString()
*/
@Override
public String toString()
{
return mongo.toString();
}
}