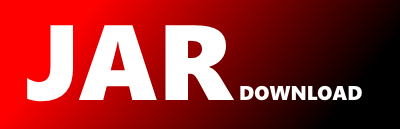
net.sf.mpxj.mpp.CustomFieldValueItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mpxj Show documentation
Show all versions of mpxj Show documentation
Library that provides facilities to allow project information to be manipulated in Java and .Net. Supports a range of data formats: Microsoft Project Exchange (MPX), Microsoft Project (MPP,MPT), Microsoft Project Data Interchange (MSPDI XML), Microsoft Project Database (MPD), Planner (XML), Primavera (PM XML, XER, and database), Asta Powerproject (PP, MDB), Asta Easyplan (PP), Phoenix Project Manager (PPX), FastTrack Schedule (FTS), and the Standard Data Exchange Format (SDEF).
/*
* file: CustomFieldValueItem.java
* author: Jari Niskala
* copyright: (c) Packwood Software 2008
* date: 17/01/2008
*/
/*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by the
* Free Software Foundation; either version 2.1 of the License, or (at your
* option) any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this library; if not, write to the Free Software Foundation, Inc.,
* 59 Temple Place, Suite 330, Boston, MA 02111-1307, USA.
*/
package net.sf.mpxj.mpp;
import java.util.UUID;
import net.sf.mpxj.CustomFieldValueDataType;
/**
* Instances of this type represent a possible value for a custom field that is
* using value lists.
*/
public final class CustomFieldValueItem
{
/**
* Constructor.
*
* @param uniqueID item unique ID
*/
public CustomFieldValueItem(Integer uniqueID)
{
m_uniqueID = uniqueID;
}
/**
* Get the unique id for this item.
*
* @return item unique ID
*/
public Integer getUniqueID()
{
return m_uniqueID;
}
/**
* Set the value of this item.
*
* @param value item value
*/
public void setValue(Object value)
{
m_value = value;
}
/**
* Get the value of this item.
*
* @return item value
*/
public Object getValue()
{
return m_value;
}
/**
* Set the description for this item.
*
* @param description item description
*/
public void setDescription(String description)
{
m_description = description;
}
/**
* Get the description for this item.
*
* @return item description
*/
public String getDescription()
{
return m_description;
}
/**
* Set an Unknown property for this item.
*
* @param unknown unknown data block
*/
public void setUnknown(byte[] unknown)
{
m_unknown = unknown;
}
/**
* Get an unknown property for this item.
*
* @return unknown data block
*/
public byte[] getUnknown()
{
return m_unknown;
}
/**
* Set the parent ID.
*
* @param id parent ID
*/
public void setParent(Integer id)
{
m_parentId = id;
}
/**
* Retrieve the parent ID.
*
* @return parent IDs
*/
public Integer getParent()
{
return m_parentId;
}
/**
* Retrieve the GUID for this value.
*
* @return value GUID
*/
public UUID getGUID()
{
return m_guid;
}
/**
* Set the GUID for this value.
*
* @param guid value GUID
*/
public void setGUID(UUID guid)
{
m_guid = guid;
}
/**
* Retrieve the value type.
*
* @return value type
*/
public CustomFieldValueDataType getType()
{
return m_type;
}
/**
* Set the value type.
*
* @param type value type
*/
public void setType(CustomFieldValueDataType type)
{
m_type = type;
}
/**
* Retrieve the collapsed flag.
*
* @return collapsed flag
*/
public boolean getCollapsed()
{
return m_collapsed;
}
/**
* Set the collapsed flag.
*
* @param collapsed collapsed flag
*/
public void setCollapsed(boolean collapsed)
{
m_collapsed = collapsed;
}
/**
* {@inheritDoc}
*/
@Override public String toString()
{
return String.format("[CustomFieldValueItem uniqueID=%d guid=%s parentId=%d value=%s description=%s]", m_uniqueID, m_guid, m_parentId, String.valueOf(m_value), m_description);
}
private Integer m_uniqueID;
private UUID m_guid;
private Object m_value;
private String m_description;
private byte[] m_unknown;
private Integer m_parentId;
private CustomFieldValueDataType m_type;
private boolean m_collapsed;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy