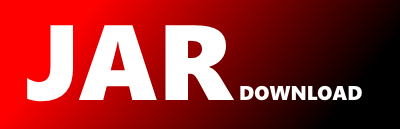
net.sf.nervalreports.generators.PDFFontInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdf-generator Show documentation
Show all versions of pdf-generator Show documentation
This is the PDF generator package of NervalReports (a lightweight report creation library),
used to generate a report directly to a .pdf file.
The newest version!
/** This file is part of nervalreports.
*
* nervalreports is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* nervalreports is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with nervalreports. If not, see . */
package net.sf.nervalreports.generators;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.HashMap;
import java.util.Map;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.font.PDFont;
import org.apache.pdfbox.pdmodel.font.PDType0Font;
import net.sf.nervalreports.core.ReportGenerationException;
/** Information about a font for PDF generator use.
* @author farrer */
public class PDFFontInfo {
private final PDFont font;
private final boolean defaultFont;
private final Map codeToWidthMap;
/** Constructor for default PDF font usage.
* @param font default PDF font. */
PDFFontInfo(PDFont font) {
this.font = font;
this.defaultFont = true;
this.codeToWidthMap = new HashMap();
}
/** Constructor, for .ttf font usage.
* @param document document where the font will be used.
* @param font {@link InputStream} for the font file.
* @throws ReportGenerationException in case of font load error. */
public PDFFontInfo(PDDocument document, InputStream font) throws ReportGenerationException {
try {
this.font = PDType0Font.load(document, font);
this.defaultFont = false;
this.codeToWidthMap = new HashMap();
} catch (IOException e) {
throw new ReportGenerationException(e);
}
}
/** @return the {@link PDFont} of this font. */
PDFont getFont() {
return font;
}
/** @return if the font is a default PDF font (true
) or loaded .ttf one (false
). */
boolean isDefaultFont() {
return defaultFont;
}
/** Calculate the LowerLeft Y coordinate of the font at an specific font size.
* @param size font size to use.
* @return calculated LowerLeft Y.
* @throws IOException in case of failure retrieving font information. */
float getLowerLeftY(int size) throws IOException {
return convertValueToScale(font.getBoundingBox().getLowerLeftY(), size);
}
/** Calculate the UpperRight Y coordinate of the font at an specific font size.
* @param size font size to use.
* @return calculated UpperRight Y.
* @throws IOException in case of failure retrieving font information. */
float getUpperRightY(int size) throws IOException {
return convertValueToScale(font.getBoundingBox().getUpperRightY(), size);
}
/** Calculate current ascend for a given font size.
* @param size font size to use.
* @return ascend value for the font size. */
float getAscend(int size) {
return convertValueToScale(font.getFontDescriptor().getAscent(), size);
}
/** Calculate current descend for a given font size.
* @param size font size to use.
* @return descend value for the font size. */
float getDescend(int size) {
return convertValueToScale(font.getFontDescriptor().getDescent(), size);
}
/** Calculate the height for a given font size.
* @param size font size to get its height.
* @return calculated height for this font size.
* @throws IOException in case of failure retrieving font information. */
float getHeight(int size) throws IOException {
return getUpperRightY(size) - getLowerLeftY(size);
}
/** Calculate the space width of the font.
* @param size space width.
* @return width of the space.
* @throws IOException in case of error retrieving. */
float getSpaceWidth(int size) throws IOException {
Float spaceWidth = codeToWidthMap.get(32);
if(spaceWidth == null) {
/* Not yet calculated, let's get it, and cache it. */
spaceWidth = font.getWidthFromFont(32);
if(!isDefaultFont()) {
//XXX adjust, as seems that the space got from font and its rendered value are different.
spaceWidth *= 0.6f;
}
codeToWidthMap.put(32, spaceWidth);
}
return convertValueToScale(spaceWidth, size);
}
/** Calculate the width necessary to render a string with a defined font size.
* @param size size of the font.
* @param str {@link String} to render.
* @return needed width.
* @throws IOException in case of missing element for calculating. */
float getStringWidth(int size, String str) throws IOException {
/* Convert to a byte array for parsing it. */
byte[] bytes = font.encode(str);
ByteArrayInputStream in = new ByteArrayInputStream(bytes);
float width = 0;
while(in.available() > 0) {
/* Get next Unicode glyph */
int code = font.readCode(in);
/* Check if it is already on map (cached) */
Float charWidth = codeToWidthMap.get(code);
if(charWidth != null) {
width += charWidth;
} else {
/* Not yet calculated, let's get it, and cache it. */
charWidth = font.getWidthFromFont(code);
codeToWidthMap.put(code, charWidth);
width += charWidth;
}
}
return convertValueToScale(width, size);
}
/** Convert from UNITS to font size.
* @param value value to convert.
* @param size size of the font (in pts).
* @return converted value. */
private float convertValueToScale(float value, int size) {
return (value / 1000) * size;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy