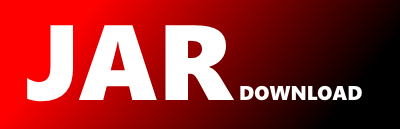
net.sf.nervalreports.generators.PDFTableInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdf-generator Show documentation
Show all versions of pdf-generator Show documentation
This is the PDF generator package of NervalReports (a lightweight report creation library),
used to generate a report directly to a .pdf file.
The newest version!
/** This file is part of nervalreports.
*
* nervalreports is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* nervalreports is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with nervalreports. If not, see . */
package net.sf.nervalreports.generators;
import java.io.IOException;
import java.util.LinkedList;
import org.apache.pdfbox.pdmodel.graphics.image.PDImageXObject;
import net.sf.nervalreports.core.ReportColor;
import net.sf.nervalreports.core.ReportTextAlignment;
import net.sf.nervalreports.generators.PDFTextSentence.PDFTextSentenceType;
/** Informations and construction state variables for current table definition for {@link PDFReportGenerator} */
/* default */ class PDFTableInfo extends PDFBlockInfo {
/** List with current active spans (row and column) in the table. */
private final LinkedList spanList;
/** Counter of the current number of rows in the table (used for color alternation). */
private int currentTableLineCounter;
/** Width information of all columns. When there's no column width information available,
* the array will be null
. */
private final int[] columnWidth;
/** Counter of row cell indexes [0,n]. */
private int currentTableRowElement;
/** Initial X position of the table. */
private final float initialX;
/** Initial Y position of the table. */
private final float initialY;
/** Total number of columns of the table. */
private final float totalColumns;
/** Table border width. */
private final Float borderWidth;
/** table border color. */
private final ReportColor borderColor;
/** Total width of table. */
private float width;
/** Current height of the table (to last finished row). */
private float height;
/** Current Y position for current on-edit row. */
private float curY;
/** Information about the current row construction. */
private final PDFTableRow currentRow;
/** Default constructor. */
PDFTableInfo(int totalColumns, float initialX, float initialY, float containerWidth, int[] columnWidth,
Float borderWidth, ReportColor borderColor) {
super(Type.PDF_TABLE_INFO);
this.initialX = initialX;
this.initialY = initialY;
this.curY = initialY;
this.totalColumns = totalColumns;
this.columnWidth = columnWidth;
this.spanList = new LinkedList();
this.borderColor = borderColor;
this.borderWidth = borderWidth;
this.currentTableLineCounter = 0;
this.currentTableRowElement = 0;
this.height = 0;
/* Apply resolution correction factor and calculate table width. */
if (this.columnWidth != null) {
this.width = 0;
for (int i = 0; i < this.columnWidth.length; i++) {
this.width += this.columnWidth[i];
}
} else {
this.width = containerWidth;
}
this.currentRow = new PDFTableRow(totalColumns, this.initialX, this.initialY, containerWidth, this.columnWidth);
}
/** @return {@link #columnWidth}. */
int[] getColumnWidth() {
return columnWidth;
}
/** @return if current row element is the first one. */
boolean isFirstTableRowElement() {
return currentTableRowElement == 0;
}
/** @return {@link #currentTableRowElement}. */
int getCurrentTableRowElement() {
return currentTableRowElement;
}
/** Reset {@link #currentTableRowElement} to 0.
* Note: it will clear the current row construction info also. */
void resetCurrentTableRowElement() {
this.currentTableRowElement = 0;
/* -=, since on pdfBox origin is at lower corner. */
this.curY -= this.currentRow.getNeededHeight();
this.currentRow.reset(this.curY);
}
/** Increment by 'n' the value of {@link #currentTableRowElement}.
* @param n amount to add to {@link #currentTableRowElement}. */
void incCurrentTableRowElement(int n) {
this.currentTableRowElement += n;
}
/** @return {@link #spanList}. */
LinkedList getSpanList() {
return spanList;
}
/** @return {@link #currentTableLineCounter}. */
int getCurrentTableLineCounter() {
return currentTableLineCounter;
}
/** Increment by one the value of {@link #currentTableLineCounter}. */
void incCurrentTableLineCounter() {
this.currentTableLineCounter++;
}
/** @return {@link #initialX}. */
float getInitialX() {
return initialX;
}
/** @return {@link #width}. */
float getWidth() {
return width;
}
/** @return {@link #initialY}. */
float getInitialY() {
return initialY;
}
/** @return the width of the current 'on-creation' column. */
float getCurrentColumnMaxWidth() {
return currentRow.getCell(currentTableRowElement).getMaxWidth();
}
/** {@inheritDoc} */
@Override
float getChildMaxWidth() {
return getCurrentColumnMaxWidth();
}
/** @return the initial X coordinate of the current 'on-creation' column. */
float getCurrentColumnInitialX() {
return currentRow.getCell(currentTableRowElement).getInitialX();
}
/** {@inheritDoc} */
@Override
float getCurrentInitialX() {
return getCurrentColumnInitialX();
}
/** Add an image to the cell, breaking lines if needed.
* @param image image to be used as sentence.
* @param width desired width to render the image at the sentence.
* @param height desired height to render the image at the sentence.
* @param colspan number of columns to use (1 is the default behavior for single column).
* @param textAlignment alignment to use. */
void addImage(PDImageXObject image, float width, float height, int colspan,
ReportTextAlignment textAlignment) {
currentRow.getCell(currentTableRowElement).addToSentenceImage(image, width, height,
calculateSpanedColsWidth(colspan), textAlignment);
}
/** Add text to the current table cell (or header), breaking lines if needed.
* @param type type of the sentence.
* @param text text of the sentence.
* @param color color to use.
* @param font font to use.
* @param fontSize size of the font.
* @param colspan number of columns to occupy (1 is the default behavior for single column).
* @param textAlignment alignment to use.
* @throws IOException in failure to determine width. */
void addText(PDFTextSentenceType type, String text, ReportColor color, PDFFontInfo font, int fontSize, int colspan,
ReportTextAlignment textAlignment) throws IOException {
currentRow.getCell(currentTableRowElement).addText(type, text, color, font, fontSize,
calculateSpanedColsWidth(colspan), textAlignment);
}
/** Add a line break to the current table cell (or header). */
void addLineBreak() {
currentRow.getCell(currentTableRowElement).addLineBreak();
}
/** @return sum of widths of all columns the current one expands into. */
private float calculateSpanedColsWidth(int colspan) {
if ((currentTableRowElement + colspan - 1) >= totalColumns) {
throw new IllegalArgumentException("Colspan should not exceed current remaining table columns.");
}
/* Calculate the spaned with of the column. */
float spanedColsWidth = 0.0f;
for (int i = 1; i < colspan; i++) {
spanedColsWidth += currentRow.getCell(currentTableRowElement + i).getMaxWidth();
}
/* Set the cell span */
currentRow.getCell(currentTableRowElement).setColspan(colspan);
return spanedColsWidth;
}
/** Get the X coordinate to start column's draw (note: only for defined column widths).
* @param columnIndex index of the column to get initial X coodinate.
* @return X coordinate of column's left corner. */
float getColumnInitialX(int columnIndex) {
return currentRow.getCell(columnIndex).getInitialX();
}
/** @return {@link #currentRow}. */
PDFTableRow getCurrentRow() {
return currentRow;
}
/** Increment the table needed height from current row. */
void incHeightFromCurrentRow() {
height += currentRow.getNeededHeight();
}
/** @return {@link #height}. */
float getHeight() {
return height;
}
/** @return {@link #borderWidth}. */
public Float getBorderWidth() {
return borderWidth;
}
/** @return {@link #borderColor}. */
public ReportColor getBorderColor() {
return borderColor;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy