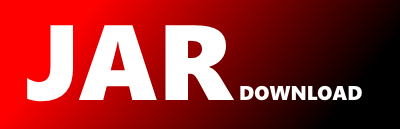
net.sf.nervalreports.generators.PDFTableRow Maven / Gradle / Ivy
/** This file is part of nervalreports.
*
* nervalreports is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* nervalreports is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with nervalreports. If not, see . */
package net.sf.nervalreports.generators;
import java.util.ArrayList;
/** Row construction information for {@link PDFReportGenerator}.
* Note: for simplicity, one row will never break in more than one page.
* @author farrer */
/* default */ class PDFTableRow {
/** Initial Y position of the row. Note that it won't reflect page breaks,
* so always continuous (absolute, not relative) to the current table initial position. */
private float initialY;
/** Current needed height (y) to contains all row cell's content. */
private float neededHeight;
/** if {@link #neededHeight} is currently defined, or to be calculated. */
private boolean definedNeededHeight;
/** Current row cells. */
private final ArrayList cells;
/** If some cell element of current row contains a table inside it (in this case,
* we must avoid filling it to not drawing over the already drawned table) */
private boolean containsTable;
/** Default constructor.
* @param totalColumns total columns of the table.
* @param initialX X position where row draw should start.
* @param initialY Y position where row draw should start.
* @param pageWidth current width of the page.
* @param columnWidth definition of width for the container of the table (usually the page itself, or another table's cell). */
PDFTableRow(int totalColumns, float initialX, float initialY, float pageWidth, int[] columnWidth) {
this.containsTable = false;
this.initialY = initialY;
int averageWidth = Math.round(pageWidth / totalColumns);
float curX = initialX;
/* Create the potential cells structure. */
cells = new ArrayList();
for (int i = 0; i < totalColumns; i++) {
int cellWidth = (columnWidth != null) ? columnWidth[i] : averageWidth;
cells.add(new PDFTableCell(curX, cellWidth));
curX += cellWidth;
}
definedNeededHeight = false;
neededHeight = 0.0f;
}
/** Constructor, copying structure (but not inner values) from another row.
* @param row row to use as reference. */
PDFTableRow(PDFTableRow row, float initialY) {
this.containsTable = false;
this.initialY = initialY;
cells = new ArrayList();
for (PDFTableCell cell : row.cells) {
cells.add(new PDFTableCell(cell.getInitialX(), cell.getMaxWidth()));
}
definedNeededHeight = false;
neededHeight = 0.0f;
}
/** Reset the values (and content) of each table. */
void reset(float initialY) {
for (PDFTableCell cell : cells) {
cell.reset();
}
this.definedNeededHeight = false;
this.containsTable = false;
this.neededHeight = 0;
this.initialY = initialY;
}
/** @return the needed height to contain all row cells content. Note that the value will only be calculated once,
* so subsequential calls (without reset) even on modified cells will return the same value. */
float getNeededHeight() {
if (!definedNeededHeight) {
neededHeight = 0;
definedNeededHeight = true;
for (PDFTableCell cell : cells) {
if (cell.getNeededHeight() > neededHeight) {
neededHeight = cell.getNeededHeight();
}
}
}
return neededHeight;
}
/** @return the cell of index */
PDFTableCell getCell(int index) {
if (index >= cells.size()) {
new IllegalArgumentException("Index beyond the total.");
}
return cells.get(index);
}
/** @return {@link #cells}. */
ArrayList getCells() {
return cells;
}
/** @return {@link #initialY}. */
float getInitialY() {
return initialY;
}
/** Define that the current cell contains a table inside it. */
void setContainsTable() {
containsTable = true;
}
/** @return {@link #containsTable}. */
boolean containsTable() {
return containsTable;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy