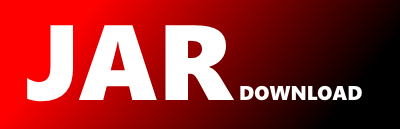
net.sf.nervalreports.generators.PDFTextSentence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdf-generator Show documentation
Show all versions of pdf-generator Show documentation
This is the PDF generator package of NervalReports (a lightweight report creation library),
used to generate a report directly to a .pdf file.
The newest version!
/** This file is part of nervalreports.
*
* nervalreports is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* nervalreports is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with nervalreports. If not, see . */
package net.sf.nervalreports.generators;
import java.io.IOException;
import org.apache.pdfbox.pdmodel.PDPageContentStream;
import net.sf.nervalreports.core.ReportColor;
/** Text construction info for {@link PDFReportGenerator}.
* @author farrer */
/* default */ class PDFTextSentence extends PDFSentence {
enum PDFTextSentenceType {
/** Just a defined fixed text. */
NORMAL,
/** Text that represents the current page number. */
CURRENT_PAGE,
/** Text that represents the total document pages. */
PAGE_COUNT
}
/** The sentence itself. */
private String text;
/** String builder to use, when text was added (instead of just a single text). */
private StringBuilder stringBuilder;
/** Type of the textual sentence. */
private final PDFTextSentenceType textType;
/** Color to use for the sentence */
private final ReportColor color;
/** Font size of the sentence. */
private final int fontSize;
/** Font used for the sentence. */
private final PDFFontInfo font;
/** Current ascend used by the font. */
private final float fontAscend;
/** Current descend used for font. */
private final float fontDescend;
/** Default constructor.
* @param type type of the textual sentence.
* @param text text of the sentence.
* @param color color to use.
* @param font font to use.
* @param fontSize size of the font.
* @param width width to display the sentence (as having a final space).
* @throws IOException in case of error retrieving the bounding box from font.
* @TODO add support for TrueType fonts with the PDType0Font PDFBox 2.0 class. */
PDFTextSentence(PDFTextSentenceType type, String text, ReportColor color, PDFFontInfo font, int fontSize, float width) throws IOException {
super(Type.PDF_TEXT_SENTENCE);
this.text = text;
this.fontSize = fontSize;
this.color = color;
this.font = font;
this.textType = type;
setWidth(width);
this.stringBuilder = null;
setHeight(font.getHeight(fontSize));
this.fontDescend = font.getDescend(fontSize);
this.fontAscend = font.getAscend(fontSize);
}
/** Add text to the current sentence.
* @param text text to add.
* @param @param widthForText width of the text we are adding (as with a final space).. */
void addText(String text, float widthForText) {
if (stringBuilder == null) {
/* Yet no string builder, let's create it, appending current defined text */
stringBuilder = new StringBuilder();
stringBuilder.append(this.text);
}
/* Append new text, prefixed with space */
stringBuilder.append(" ");
stringBuilder.append(text);
setWidth(getWidth() + widthForText);
/* Make sure will recalculate the text from StringBuilder when needed. */
this.text = null;
}
/** @return if current sentence is compatible with another one. */
boolean isCompatibleWith(PDFTextSentenceType type, ReportColor color, PDFFontInfo font, int fontSize) {
return this.textType.equals(type) && this.color.equals(color) && this.font.equals(font) && (this.fontSize == fontSize);
}
/** @return {@link #text}. */
String getText() {
if ((text == null) && (stringBuilder != null)) {
/* Text must be built from builder. */
text = stringBuilder.toString();
}
return text;
}
/** Replace the current {@link #text}, keeping its style. */
void replaceText(String text, float width) {
this.text = text;
this.stringBuilder = null;
setWidth(width);
}
/** @return {@link #color}. */
ReportColor getColor() {
return color;
}
/** @return {@link #fontSize}. */
int getFontSize() {
return fontSize;
}
/** @return {@link #font}. */
PDFFontInfo getFont() {
return font;
}
/** @return {@link #type}. */
PDFTextSentenceType getTextType() {
return textType;
}
/** {@inheritDoc} */
@Override
void flush(PDPageContentStream pageContentStream, float x, float y) throws Exception {
pageContentStream.beginText();
pageContentStream.setFont(getFont().getFont(), getFontSize());
pageContentStream.setNonStrokingColor(color.getRed(), color.getGreen(), color.getBlue());
/* Note: XXX seems like a pdfbox bug: the starting point of render for default fonts is different from the
* render of embedded .ttf fonts. */
/* Note: fontDescend is relative to font origin, so we use inverted signals to convert to our coordinates. */
if(font.isDefaultFont()) {
pageContentStream.newLineAtOffset(x, y - getHeight() + fontAscend + fontDescend - 1);
} else {
pageContentStream.newLineAtOffset(x, y - getHeight() + ((fontAscend + fontDescend - 1) / 2.0f));
}
pageContentStream.showText(getText());
pageContentStream.endText();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy