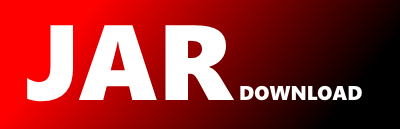
net.sf.okapi.common.ui.InputDocumentPanel Maven / Gradle / Ivy
/*===========================================================================
Copyright (C) 2010-2012 by the Okapi Framework contributors
-----------------------------------------------------------------------------
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
===========================================================================*/
package net.sf.okapi.common.ui;
import java.nio.charset.Charset;
import java.util.List;
import net.sf.okapi.common.FileUtil;
import net.sf.okapi.common.LocaleId;
import net.sf.okapi.common.Util;
import net.sf.okapi.common.filters.FilterConfiguration;
import net.sf.okapi.common.filters.IFilterConfigurationListEditor;
import net.sf.okapi.common.filters.IFilterConfigurationMapper;
import net.sf.okapi.common.ui.filters.FilterConfigurationEditor;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.layout.GridData;
import org.eclipse.swt.layout.GridLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Text;
public class InputDocumentPanel extends Composite {
private Label stDocument;
private Text edDocument;
private Label stConfigId;
private Text edConfigId;
private Button btGetFile;
private Button btGetConfigId;
private Label stEncoding;
private Text edEncoding;
private Label stSourceLocale;
private Text edSourceLocale;
private Label stTargetLocale;
private Text edTargetLocale;
private String title;
private String filterNames;
private String filterExtensions;
private IFilterConfigurationMapper fcMapper;
public InputDocumentPanel (Composite parent,
int flags,
int horizontalSpan,
String documentLabel,
String getDocumentLabel,
IFilterConfigurationMapper fcMapper)
{
super(parent, flags);
createContent(horizontalSpan, documentLabel, getDocumentLabel, fcMapper);
}
private void createContent (int horizontalSpan,
String docLabel,
String getDocLabel,
IFilterConfigurationMapper fcMapper)
{
this.fcMapper = fcMapper;
title = "Select a File";
GridLayout layTmp = new GridLayout(3, false);
layTmp.marginHeight = 0;
layTmp.marginWidth = 0;
setLayout(layTmp);
GridData gdTmp = new GridData(GridData.FILL_HORIZONTAL);
gdTmp.horizontalSpan = horizontalSpan;
setLayoutData(gdTmp);
//--- Document path
if ( docLabel != null ) {
stDocument = new Label(this, SWT.NONE);
stDocument.setText(docLabel);
gdTmp = new GridData();
gdTmp.horizontalSpan = 3;
stDocument.setLayoutData(gdTmp);
}
edDocument = new Text(this, SWT.BORDER);
gdTmp = new GridData(GridData.FILL_HORIZONTAL);
gdTmp.horizontalSpan = 2;
edDocument.setLayoutData(gdTmp);
btGetFile = new Button(this, SWT.PUSH);
if ( getDocLabel == null ) btGetFile.setText("...");
else btGetFile.setText(getDocLabel);
btGetFile.addSelectionListener(new SelectionAdapter() {
public void widgetSelected(SelectionEvent e) {
browsePath();
};
});
//--- Configuration id
stConfigId = new Label(this, SWT.NONE);
stConfigId.setText("Configuration:");
edConfigId = new Text(this, SWT.BORDER);
edConfigId.setLayoutData(new GridData(GridData.FILL_HORIZONTAL));
btGetConfigId = new Button(this, SWT.PUSH);
btGetConfigId.setText("...");
btGetConfigId.addSelectionListener(new SelectionAdapter() {
public void widgetSelected(SelectionEvent e) {
browseConfiguration();
};
});
//--- Encoding
stEncoding = new Label(this, SWT.NONE);
stEncoding.setText("Encoding:");
edEncoding = new Text(this, SWT.BORDER);
edEncoding.setLayoutData(new GridData(GridData.FILL_HORIZONTAL));
new Label(this, SWT.NONE); // Fill extra cell rather than stretching edit field
//--- Source locale
stSourceLocale = new Label(this, SWT.NONE);
stSourceLocale.setText("Source locale:");
edSourceLocale = new Text(this, SWT.BORDER);
edSourceLocale.setLayoutData(new GridData(GridData.FILL_HORIZONTAL));
new Label(this, SWT.NONE); // Fill extra cell rather than stretching edit field
//--- Target locale
stTargetLocale = new Label(this, SWT.NONE);
stTargetLocale.setText("Target locale:");
edTargetLocale = new Text(this, SWT.BORDER);
edTargetLocale.setLayoutData(new GridData(GridData.FILL_HORIZONTAL));
new Label(this, SWT.NONE); // Fill extra cell rather than stretching edit field
}
public String getDocumentPath () {
return edDocument.getText();
}
public void setDocumentPath (String path) {
edDocument.setText(path == null ? "" : path);
}
public String getFilterConfigurationId () {
return edConfigId.getText();
}
public void setFilterConfigurationId (String configId) {
edConfigId.setText(configId == null ? "" : configId);
}
public String getEncoding () {
return edEncoding.getText();
}
public void setEncoding (String encoding) {
edEncoding.setText(encoding == null ? "" : encoding);
}
public LocaleId getSourceLocale () {
if ( edSourceLocale.getText().isEmpty() ) return LocaleId.EMPTY;
return LocaleId.fromString(edSourceLocale.getText());
}
public void setSourceLocale (LocaleId sourceLocale) {
edSourceLocale.setText(sourceLocale == null ? "" : sourceLocale.toString());
}
public LocaleId getTargetLocale () {
if ( edTargetLocale.getText().isEmpty() ) return LocaleId.EMPTY;
return LocaleId.fromString(edTargetLocale.getText());
}
public void setTargetLocale (LocaleId targetLocale) {
edTargetLocale.setText(targetLocale == null ? "" :targetLocale.toString());
}
public void guessConfiguration () {
String ext = Util.getExtension(edDocument.getText());
FilterConfiguration fc = fcMapper.getDefaultConfigurationFromExtension(ext);
if ( fc != null ) {
edConfigId.setText(fc.configId);
}
}
public void guessLocales () {
// Guess the languages if possible
if ( edSourceLocale.getEditable() ) {
List list = FileUtil.guessLanguages(edDocument.getText());
if ( list.size() > 0 ) {
edSourceLocale.setText(list.get(0));
}
if ( list.size() == 2 ) {
edTargetLocale.setText(list.get(1));
}
else if ( list.size() > 2 ) {
StringBuilder tmp = new StringBuilder(list.get(1));
for ( int i=2; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy