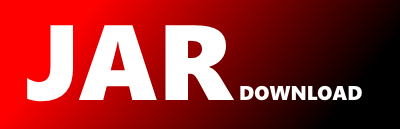
net.sf.okapi.common.IParameters Maven / Gradle / Ivy
/*===========================================================================
Copyright (C) 2008-2010 by the Okapi Framework contributors
-----------------------------------------------------------------------------
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
===========================================================================*/
package net.sf.okapi.common;
import java.io.InputStream;
import java.net.URL;
/**
* Common way for a component to expose its
* parameters in a generic way.
*/
public interface IParameters {
/**
* Reset the parameters to their default values.
*/
public void reset ();
/**
* Converts the parameters into a string.
* @return the string holding all the parameters.
*/
public String toString ();
/**
* Parses the parameters stored into a string back into the object.
* @param data the string holding the parameters. It must be formatted as
* the string generated by toString(). Line-breaks must be normalized to '\n'.
* It can also be null or empty, in such case the parameters are left with
* their current values. Use reset() to reset the parameters to their defaults.
*/
public void fromString (String data);
/**
* Loads the parameters from a file.
* @param inputURL URL of the parameters file to load.
* @param ignoreErrors true if the load should ignore any error
* such as file not found. If an error occurs and this is set to true,
* the method should create the parameters object with its default values.
*/
public void load (URL inputURL,
boolean ignoreErrors);
/**
* Loads the parameters from a file.
* @param inStream input stream with the parameters to load.
* @param ignoreErrors true if the load should ignore any error
* such as file not found. If an error occurs and this is set to true,
* the method should create the parameters object with its default values.
*/
public void load (InputStream inStream,
boolean ignoreErrors);
/**
* Saves the parameters to a file.
* @param filePath the full path of the parameters file to save.
*/
public void save (String filePath);
/**
* Gets the full path of the last file where the parameters where loaded
* from or saved to.
* @return the full path of the last load() or save(), or null if object
* was not loaded nor saved.
*/
public String getPath ();
/**
* Sets the full path of the file where the parameters are to be
* saved to.
* @param filePath the full path to set.
*/
public void setPath (String filePath);
/**
* Gets the boolean value for the given parameter name.
* @param name the name of the boolean parameter to retrieve.
* @return the value of the given parameter, or false if it is not defined.
*/
public boolean getBoolean (String name);
/**
* Sets the boolean value for a given parameter name.
* @param name the name of the parameter to set.
* @param value the new value to set.
*/
public void setBoolean (String name,
boolean value);
/**
* Gets the string value for a given parameter name.
* @param name the name of the string parameter to retrieve.
* @return the value of the given parameter, or null if it is not defined.
*/
public String getString (String name);
/**
* Sets the string value for a given parameter name.
* @param name the name of the parameter to set.
* @param value the new value to set.
*/
public void setString (String name,
String value);
/**
* Gets the integer value for a given parameter name.
* @param name the name of the integer parameter to retrieve.
* @return the value of the given parameter, or 0 if it is not defined.
*/
public int getInteger (String name);
/**
* Sets the integer value for a given parameter name.
* @param name the name of the parameter to set.
* @param value the new value to set.
*/
public void setInteger (String name,
int value);
/**
* Gets the description of the parameters.
* @return the ParametersDescription object for this set of parameters,
* or null if none is provided.
*/
public ParametersDescription getParametersDescription ();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy