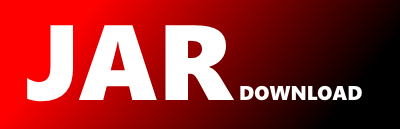
net.sf.okapi.common.resource.CodeMatches Maven / Gradle / Ivy
/*===========================================================================
Copyright (C) 2021 by the Okapi Framework contributors
-----------------------------------------------------------------------------
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
===========================================================================*/
package net.sf.okapi.common.resource;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
final public class CodeMatches {
public static int NO_MATCH = Integer.MIN_VALUE;
public static int ADDED_MATCH = Integer.MIN_VALUE+1;
public static int ANNOTATION_ONLY = Integer.MIN_VALUE+2;
public static CodeMatches NO_CODES = new CodeMatches(0, 0);
public static CodeMatches SAME_CODES = new CodeMatches(0, 0);
private final int[] fromMatches;
private final int[] toMatches;
private final boolean[] toIsolated;
private final boolean[] fromIsolated;
private CodeMatches(int fromSize, int toSize) {
fromMatches = new int[fromSize];
toMatches = new int[toSize];
toIsolated = new boolean[toSize];
fromIsolated = new boolean[fromSize];
Arrays.fill(fromMatches, NO_MATCH);
Arrays.fill(toMatches, NO_MATCH);
Arrays.fill(toIsolated, false);
Arrays.fill(fromIsolated, false);
}
public CodeMatches(TextFragment from, TextFragment to) {
this(from.codes.size(), to.codes.size());
// set ANNOTATION_ONLY and isolated flag
for (int i = 0; i < from.codes.size(); i++) {
Code c = from.getCode(i);
if (c.type.equals(Code.TYPE_ANNOTATION_ONLY)) {
fromMatches[i] = ANNOTATION_ONLY;
}
int pos = from.getCodePosition(i);
switch (from.charAt(pos)) {
case TextFragment.MARKER_ISOLATED:
fromIsolated[i] = true;
}
}
for (int i = 0; i < to.codes.size(); i++) {
Code c = to.getCode(i);
if (c.type.equals(Code.TYPE_ANNOTATION_ONLY)) {
toMatches[i] = ANNOTATION_ONLY;
}
int pos = to.getCodePosition(i);
switch (to.charAt(pos)) {
case TextFragment.MARKER_ISOLATED:
toIsolated[i] = true;
}
}
}
public int[] getFromMatches() {
return fromMatches;
}
public int[] getToMatches() {
return toMatches;
}
public void setFromMatch(int index, int id) {
fromMatches[index] = id;
}
public void setToMatch(int index, int id) {
toMatches[index] = id;
}
public int getToMatchIndex(int toIndex) {
if (toMatches[toIndex] == ANNOTATION_ONLY) {
return ANNOTATION_ONLY;
}
if (toMatches[toIndex] != NO_MATCH) {
return toMatches[toIndex];
}
return NO_MATCH;
}
public boolean isToIsolated(int index) {
return toIsolated[index];
}
public boolean isFromIsolated(int index) {
return fromIsolated[index];
}
public boolean hasFromMismatch() {
return hasFromMismatch(true);
}
public boolean hasFromMismatch(boolean includeAnnotation) {
for (int m : fromMatches) {
if (includeAnnotation && m == ANNOTATION_ONLY) {
return true;
}
if (m == NO_MATCH) {
return true;
}
}
return false;
}
public boolean hasToMismatch() {
return hasToMismatch(true);
}
public boolean hasToMismatch(boolean includeAnnotation) {
for (int m : toMatches) {
if (includeAnnotation && m == ANNOTATION_ONLY) {
return true;
}
if (m == NO_MATCH) {
return true;
}
}
return false;
}
public Iterable getFromMismatchIterator() {
return getMismatchIterator(fromMatches);
}
public Iterable getToMismatchIterator() {
return getMismatchIterator(toMatches);
}
private Iterable getMismatchIterator(int[] matches) {
List mismatchIndexes = new ArrayList<>();
int i = -1;
for (int m : matches) {
i++;
if (m == ANNOTATION_ONLY) {
continue;
}
if (m == NO_MATCH) {
mismatchIndexes.add(i);
}
}
return mismatchIndexes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy