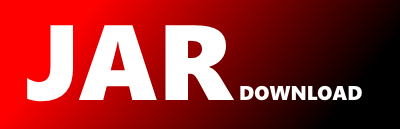
net.sf.okapi.common.skeleton.GenericSkeleton Maven / Gradle / Ivy
/*===========================================================================
Copyright (C) 2008-2011 by the Okapi Framework contributors
-----------------------------------------------------------------------------
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
===========================================================================*/
package net.sf.okapi.common.skeleton;
import java.util.ArrayList;
import java.util.List;
import net.sf.okapi.common.IResource;
import net.sf.okapi.common.ISkeleton;
import net.sf.okapi.common.LocaleId;
import net.sf.okapi.common.Util;
import net.sf.okapi.common.resource.INameable;
import net.sf.okapi.common.resource.IReferenceable;
import net.sf.okapi.common.resource.ITextUnit;
import net.sf.okapi.common.resource.TextFragment;
/**
* Simple generic implementation of the ISkeleton interface.
* This class implements a skeleton as a list of parts: some are simple text storage
* string corresponding to the original code of the input document, others are
* placeholders for the content the the text units, or the values of modifiable
* properties.
*/
public class GenericSkeleton implements ISkeleton {
private ArrayList list = new ArrayList<>();;
private boolean createNew = true;
private IResource parent;
/**
* Creates a new empty GenericSkeleton object.
*/
public GenericSkeleton () {
}
/**
* Creates a new GenericSkeleton object and append some data to it.
* @param data the data to append.
*/
public GenericSkeleton (String data) {
if ( data != null ) add(data);
}
/**
* Creates a new GenericSkeleton object and initialize it with the parts
* of an existing one passed as a parameter.
* @param skel the existing skeleton from which to copy the parts.
*/
public GenericSkeleton (GenericSkeleton skel) {
if (skel != null) {
list = new ArrayList<>(skel.list);
}
this.createNew = skel.createNew;
}
/**
* Indicates if this skeleton is empty or not.
* @return true if this skeleton is empty, false if it has at least one part.
*/
public boolean isEmpty () {
return (list.isEmpty());
}
/**
* Indicates if this skeleton is empty or not, considering the white-spaces
* or not.
* @param ignoreWhitespaces true to ignore the white-spaces.
* @return true if this skeleton is empty, false if it has at least one part.
*/
public boolean isEmpty (boolean ignoreWhitespaces) {
if ( ignoreWhitespaces ) {
for ( GenericSkeletonPart part : list ) {
for ( int i=0; i getParts () {
return list;
}
/*
* For serialization only
*/
protected void setParts(ArrayList list) {
this.list = list;
}
/**
* Gets a string representation of the content of all the part of the skeleton.
* This should be used for display only.
*/
@Override
public String toString() {
StringBuilder b = new StringBuilder();
for (GenericSkeletonPart part : list) {
b.append(part.toString());
}
return b.toString();
}
/**
* Gets the last part of this skeleton, or null if there are none.
* @return the last part of this skeleton, or null if there are none.
*/
public GenericSkeletonPart getLastPart () {
if ( list.size() == 0 ) return null;
else return list.get(list.size()-1);
}
/**
* Gets the first part of this skeleton, or null if there are none.
* @return the first part of this skeleton, or null if there are none.
*/
public GenericSkeletonPart getFirstPart () {
if ( list.size() == 0 ) return null;
else return list.get(0);
}
protected void copyFields(GenericSkeleton toSkel) {
toSkel.createNew = this.createNew;
toSkel.parent = this.parent;
if (toSkel.list == null) {
toSkel.list = new ArrayList<>();
}
for (GenericSkeletonPart part : list) {
GenericSkeletonPart newPart = new GenericSkeletonPart(part.data.toString(), part.parent, part.locId);
toSkel.list.add(newPart);
}
}
/**
* Clones this GenericSkeleton object. Shallow copy is provided as the cloned skeleton can be coupled with
* its original via the parent field. After the cloned skeleton is attached to a parent resource which implementation
* invokes ISkeleton.setParent(), the copy becomes deep as the parent fields are updated with new values thus decoupling
* the original and the clone.
* @return a new GenericSkeleton object that is a shallow copy of this object.
*/
@Override
public GenericSkeleton clone() {
GenericSkeleton newSkel = new GenericSkeleton();
copyFields(newSkel);
return newSkel;
}
@Override
public IResource getParent() {
return parent;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy