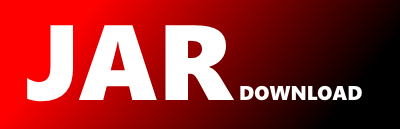
net.sf.okapi.lib.search.lucene.query.TmFuzzyQuery Maven / Gradle / Ivy
package net.sf.okapi.lib.search.lucene.query;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import net.sf.okapi.lib.search.lucene.scorer.TmFuzzyScorer;
import org.apache.lucene.index.IndexReader;
import org.apache.lucene.index.LeafReaderContext;
import org.apache.lucene.index.Term;
import org.apache.lucene.search.*;
@SuppressWarnings("serial")
public class TmFuzzyQuery extends Query {
float threshold;
List terms;
String termCountField;
public TmFuzzyQuery(float threshold, String termCountField) {
this.threshold = threshold;
this.terms = new ArrayList<>();
this.termCountField = termCountField;
}
public void add(Term term) {
terms.add(term);
}
@Override
public Weight createWeight(IndexSearcher searcher, ScoreMode scoreMode, float boost) throws IOException {
return new TmFuzzyWeight(this);
}
@Override
public Query rewrite(IndexReader reader) throws IOException {
return this; // Is this acceptable? This query isn't made of other Query objects...
}
// equals and hashCode were generated by Intellij Idea
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof TmFuzzyQuery)) return false;
TmFuzzyQuery that = (TmFuzzyQuery) o;
if (Float.compare(that.threshold, threshold) != 0) return false;
if (!terms.equals(that.terms)) return false;
return termCountField.equals(that.termCountField);
}
@Override
public int hashCode() {
int result = (threshold != +0.0f ? Float.floatToIntBits(threshold) : 0);
result = 31 * result + terms.hashCode();
result = 31 * result + termCountField.hashCode();
return result;
}
@Override
public String toString(String field) {
return terms.toString();
}
protected class TmFuzzyWeight extends Weight {
public TmFuzzyWeight(Query query) throws IOException {
super(query);
}
@Override
public void extractTerms(Set set) {
/*
* This is deprecated. Following the suggestion found in Weight#extractTerms(Set):
* Use Query.visit(QueryVisitor) with QueryVisitor.termCollector(Set)
*/
getQuery().visit(QueryVisitor.termCollector(set));
}
@Override
public Explanation explain(LeafReaderContext leafReaderContext, int i) throws IOException {
//TODO:Implement me
// Was: return new Explanation(getValue(), toString());
/* Hint. This is what explain looks like for TermQuery.TermWeight
TermScorer scorer = (TermScorer) scorer(context);
if (scorer != null) {
int newDoc = scorer.iterator().advance(doc);
if (newDoc == doc) {
float freq = scorer.freq();
LeafSimScorer docScorer = new LeafSimScorer(simScorer, context.reader(), term.field(), true);
Explanation freqExplanation = Explanation.match(freq, "freq, occurrences of term within document");
Explanation scoreExplanation = docScorer.explain(doc, freqExplanation);
return Explanation.match(
scoreExplanation.getValue(),
"weight(" + getQuery() + " in " + doc + ") ["
+ similarity.getClass().getSimpleName() + "], result of:",
scoreExplanation);
}
}
return Explanation.noMatch("no matching term");
*/
return Explanation.match(Float.valueOf(1.0f), "Sorry, TmFuzzyWeight.explain(...) not fully implemented");
}
@Override
public Scorer scorer(LeafReaderContext leafReaderContext) throws IOException {
// optimize zero-term or no match case
if (terms.size() == 0)
return null;
return new TmFuzzyScorer(threshold, this, terms, leafReaderContext.reader(), termCountField);
}
@Override
public boolean isCacheable(LeafReaderContext leafReaderContext) {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy