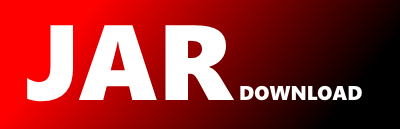
net.sf.okapi.lib.verification.Issue Maven / Gradle / Ivy
/*===========================================================================
Copyright (C) 2010-2013 by the Okapi Framework contributors
-----------------------------------------------------------------------------
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
===========================================================================*/
package net.sf.okapi.lib.verification;
import java.net.URI;
import java.util.List;
import net.sf.okapi.common.LocaleId;
import net.sf.okapi.common.Util;
import net.sf.okapi.common.annotation.GenericAnnotation;
import net.sf.okapi.common.annotation.GenericAnnotationType;
import net.sf.okapi.common.annotation.IssueType;
import net.sf.okapi.common.resource.Code;
import net.sf.okapi.common.resource.ITextUnit;
import net.sf.okapi.common.resource.TextContainer;
public class Issue extends GenericAnnotation {
public static final int DISPSEVERITY_LOW = 0;
public static final int DISPSEVERITY_MEDIUM = 1;
public static final int DISPSEVERITY_HIGH = 2;
public static final double SEVERITY_LOW = 1.0;
public static final double SEVERITY_MEDIUM = 50.0;
public static final double SEVERITY_HIGH = 100.0;
private URI docURI;
private String subDocId;
private IssueType issueType;
private String tuId;
private String segId;
private String tuName;
private int trgStart;
private int trgEnd;
private List codes;
private String source;
private String target;
private int dispSeverity;
private TextContainer srcContainer;
private TextContainer trgContainer;
public Issue (URI docId,
String subDocId,
IssueType issueType,
String tuId,
String segId,
String message,
int srcStart,
int srcEnd,
int trgStart,
int trgEnd,
double severity,
String tuName)
{
super(GenericAnnotationType.LQI);
this.docURI = docId;
this.subDocId = subDocId;
this.issueType = issueType;
this.tuId = tuId;
this.segId = segId;
setString(GenericAnnotationType.LQI_COMMENT, message);
setInteger(GenericAnnotationType.LQI_XSTART, srcStart);
setInteger(GenericAnnotationType.LQI_XEND, srcEnd);
this.trgStart = trgStart;
this.trgEnd = trgEnd;
this.dispSeverity = severityToDisplaySeverity(severity);
setDouble(GenericAnnotationType.LQI_SEVERITY, severity);
this.tuName = tuName;
setBoolean(GenericAnnotationType.LQI_ENABLED, true);
}
public URI getDocumentURI () {
return docURI;
}
public String getSubDocumentId () {
return subDocId;
}
public IssueType getIssueType () {
return issueType;
}
public String getITSType () {
return getString(GenericAnnotationType.LQI_TYPE);
}
public String getTuId () {
return tuId;
}
public String getTuName () {
return tuName;
}
public String getSegId () {
return segId;
}
public int getSourceStart () {
return getInteger(GenericAnnotationType.LQI_XSTART);
}
public int getSourceEnd () {
return getInteger(GenericAnnotationType.LQI_XEND);
}
public int getTargetStart () {
return trgStart;
}
public int getTargetEnd () {
return trgEnd;
}
public boolean getEnabled () {
return getBoolean(GenericAnnotationType.LQI_ENABLED);
}
public void setEnabled (boolean enabled) {
setBoolean(GenericAnnotationType.LQI_ENABLED, enabled);
}
public int getDisplaySeverity () {
return dispSeverity;
}
public double getSeverity () {
return getDouble(GenericAnnotationType.LQI_SEVERITY);
}
public String getMessage () {
return getString(GenericAnnotationType.LQI_COMMENT);
}
public List getCodes () {
return codes;
}
public void setCodes (List codes) {
this.codes = codes;
}
public String getSource () {
return source;
}
public void setSource (String source) {
this.source = source;
}
public String getTarget () {
return target;
}
public void setTarget (String target) {
this.target = target;
}
public void setContainers (ITextUnit tu,
LocaleId trgLoc)
{
srcContainer = tu.getSource();
trgContainer = null;
if ( tu.hasTarget(trgLoc) ) {
trgContainer = tu.getTarget(trgLoc);
}
}
public TextContainer getSourceContainer () {
return srcContainer;
}
public TextContainer getTargetContainer () {
return trgContainer;
}
String getSignature () {
return String.format("%s-%s-%s-%s-%d-%s", docURI, subDocId, tuId,
(segId==null) ? "" : segId, getInteger(GenericAnnotationType.LQI_XSTART), issueType);
}
/**
* Gets the string representation of the issue.
* TEST ONLY: The representation in raw XML (ITS 2.0 QA error element).
*/
@Override
public String toString () {
StringBuilder tmp = new StringBuilder();
tmp.append(""+Util.escapeToXML(getString(GenericAnnotationType.LQI_COMMENT), 0, false, null)+"");
tmp.append(" ");
return tmp.toString();
}
public static int severityToDisplaySeverity (double value) {
if ( value < 33.33 ) return DISPSEVERITY_LOW;
if (( value >= 33.33 ) && ( value < 66.33 )) return DISPSEVERITY_MEDIUM;
return DISPSEVERITY_HIGH;
}
public static double displaySeverityToSeverity (int value) {
if (value == DISPSEVERITY_LOW) return SEVERITY_LOW;
if (value == DISPSEVERITY_MEDIUM) return SEVERITY_MEDIUM;
return SEVERITY_HIGH;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy