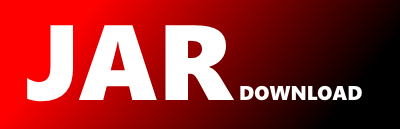
net.sf.okapi.lib.xliff2.URIPrefixes Maven / Gradle / Ivy
/*===========================================================================
Copyright (C) 2013-2014 by the Okapi Framework contributors
-----------------------------------------------------------------------------
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
===========================================================================*/
package net.sf.okapi.lib.xliff2;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
/**
* Represents the prefixes for modules and extensions in XLIFF URI fragment identifiers.
* This object is set to load automatically a default mapping and possibly a custom one
* the first time {@link #resolve(String)} is called.
*/
public class URIPrefixes {
// See https://lists.oasis-open.org/archives/xliff/201708/msg00078.html for more information
private final String REGISTRY_URL = "https://tools.oasis-open.org/version-control/browse/wsvn/xliff/trunk/xliff2-fragid/registry.txt?op=dl&isdir=0";
private Map> prefixes;
private File extraPrefixes;
/**
* Creates a simple URIPrefixes object.
*/
public URIPrefixes () {
// Nothing to do: The defaults are loaded if needed
}
/**
* Creates a URIPrefixes object with a given file where custom prefixes are stored.
* @param extraPrefixes the properties file where the custom prefixes are stored (can be null)
*/
public URIPrefixes (File extraPrefixes) {
// Set the path of the extra prefixes
this.extraPrefixes = extraPrefixes;
// Nothing more to do: The defaults and the extra are loaded if needed
}
/**
* Loads the default prefixes from the default properties file.
* This method is called automatically if needed in {@link #resolve(String)}.
*/
public void loadDefaults () {
prefixes = loadFromPropertiesFile(
getClass().getResourceAsStream("/net/sf/okapi/lib/xliff2/prefixes.properties"));
}
/**
* Loads a properties file and create the corresponding mapping from it.
* @param inputStream the input stream of the properties file to load.
* @return the mapping for the given properties file.
*/
private Map> loadFromPropertiesFile (InputStream inputStream) {
Map> prefixes = new LinkedHashMap<>();
Properties prop = new Properties();
try {
// Load the properties file
prop.load(new InputStreamReader(inputStream, StandardCharsets.UTF_8));
// Fill the map
for ( Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy