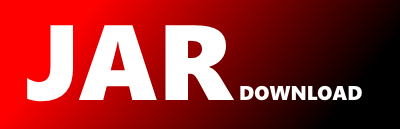
net.sf.okapi.lib.xliff2.reader.URIContext Maven / Gradle / Ivy
/*===========================================================================
Copyright (C) 2013-2014 by the Okapi Framework contributors
-----------------------------------------------------------------------------
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
===========================================================================*/
package net.sf.okapi.lib.xliff2.reader;
import java.util.AbstractMap.SimpleEntry;
import java.util.List;
import net.sf.okapi.lib.xliff2.Const;
import net.sf.okapi.lib.xliff2.URIParser;
import net.sf.okapi.lib.xliff2.Util;
import net.sf.okapi.lib.xliff2.core.CTag;
import net.sf.okapi.lib.xliff2.core.ExtAttribute;
import net.sf.okapi.lib.xliff2.core.ExtAttributes;
import net.sf.okapi.lib.xliff2.core.ExtChildType;
import net.sf.okapi.lib.xliff2.core.ExtElement;
import net.sf.okapi.lib.xliff2.core.ExtElements;
import net.sf.okapi.lib.xliff2.core.IExtChild;
import net.sf.okapi.lib.xliff2.core.IWithExtElements;
import net.sf.okapi.lib.xliff2.core.IWithNotes;
import net.sf.okapi.lib.xliff2.core.Note;
import net.sf.okapi.lib.xliff2.core.Part;
import net.sf.okapi.lib.xliff2.core.Tag;
import net.sf.okapi.lib.xliff2.core.Tags;
import net.sf.okapi.lib.xliff2.core.Unit;
import net.sf.okapi.lib.xliff2.glossary.GlossEntry;
import net.sf.okapi.lib.xliff2.glossary.Translation;
import net.sf.okapi.lib.xliff2.matches.Match;
/**
* Represents the context for URI fragment identifier resolution in XLIFF.
*/
public class URIContext implements Cloneable {
private String fileId;
private String groupId;
private String unitId;
@Override
public URIContext clone () {
URIContext tmp = new URIContext();
tmp.setFileId(fileId);
tmp.setGroupId(groupId);
tmp.setUnitId(unitId);
return tmp;
}
/**
* Gets the id of the file selector.
* @return the id of the file selector (can be null).
*/
public String getFileId () {
return fileId;
}
/**
* Sets the the id of the file selector.
* @param fileId the new id of the file selector (can be null).
*/
public void setFileId (String fileId) {
this.fileId = fileId;
}
/**
* Gets the id of the group selector.
* @return the id of the group selector (can be null).
*/
public String getGroupId () {
return groupId;
}
/**
* Sets the id of the group selector.
* @param groupId the new id of the group selector (can be null).
*/
public void setGroupId (String groupId) {
this.groupId = groupId;
}
/**
* Gets the id of the unit selector.
* @return the id of the unit selector (can be null).
*/
public String getUnitId () {
return unitId;
}
/**
* Sets the id of the unit selector.
* @param unitId the new id of the unit selector (can be null).
*/
public void setUnitId (String unitId) {
this.unitId = unitId;
}
public Object matches (Event event,
URIParser up)
{
String scope = up.getScope();
if ( scope.isEmpty() ) return null;
char refType = up.getRefType();
Object obj = null;
for ( int i=0; i> extensionInfo)
{
Object obj;
// Search the Translation Candidates module
if ( unit.hasMatch() ) {
for ( Match match : unit.getMatches() ) {
// Check Translation Candidates namespace
for ( String searchNs : extensionInfo.getValue() ) {
if ( searchNs.equals(Const.NS_XLIFF_MATCHES20) ) {
if ( Util.compareAllowingNull(match.getId(), extensionInfo.getKey()) == 0 ) {
return match;
}
}
}
// Or extensions
obj = searchExtensions(match, extensionInfo);
if ( obj != null ) return obj;
}
}
// Search the Glossary module
if ( unit.hasGlossEntry() ) {
for ( GlossEntry entry : unit.getGlossary() ) {
// Check the Glossary namespace
for ( String searchNs : extensionInfo.getValue() ) {
if ( searchNs.equals(Const.NS_XLIFF_GLOSSARY20) ) {
// Check glossEntry
if ( Util.compareAllowingNull(entry.getId(), extensionInfo.getKey()) == 0 ) {
return entry;
}
// Check translation objects
for ( Translation trans : entry ) {
if ( Util.compareAllowingNull(trans.getId(), extensionInfo.getKey()) == 0 ) {
return trans;
}
}
}
}
// Or extensions
obj = searchExtensions(entry, extensionInfo);
if ( obj != null ) return obj;
}
}
// // Search the ITS data
// if ( unit.hasITSGroup() ) {
// for ( DataCategoryGroup> dcg : unit.getITSGroups() ) {
// for ( String searchNs : extensionInfo.getValue() ) {
// if ( searchNs.equals(Const.NS_ITS) ) {
// if ( dcg.getGroupId().equals(extensionInfo.getKey()) ) {
// return dcg;
// }
// }
// }
// }
// }
return searchExtensions(unit, extensionInfo);
}
/**
* Searches recursively an {@link IWithExtElements} object for an extension object with a given id.
* @param parent the object where to search.
* @param extensionInfo the information for the object to search for.
* @return the object if found or null if not found.
*/
static public Object searchExtensions (IWithExtElements parent,
SimpleEntry> extensionInfo)
{
// Else: search just the extensions
ExtElements elems = parent.getExtElements();
for ( ExtElement elem : elems ) {
Object obj = searchMatch(elem, extensionInfo);
if ( obj != null ) return obj; // Found it
}
return null;
}
static public Object searchMatch (ExtElement elem,
SimpleEntry> extensionInfo)
{
// Search for an id in this element
for ( String searchNs : extensionInfo.getValue() ) {
if ( searchNs.equals(elem.getQName().getNamespaceURI()) ) {
ExtAttributes attrs = elem.getExtAttributes();
// Try the current namespace
ExtAttribute attr = attrs.getAttribute("", "id");
if ( attr != null ) {
if ( extensionInfo.getKey().equals(attr.getValue()) ) return elem;
}
// Try xml:id
attr = attrs.getAttribute(Const.NS_XML, "id");
if ( attr != null ) {
if ( extensionInfo.getKey().equals(attr.getValue()) ) return elem;
}
}
}
// Then move to children
for ( IExtChild child : elem.getChildren() ) {
if ( child.getType() == ExtChildType.ELEMENT ) {
Object obj = searchMatch((ExtElement)child, extensionInfo);
if ( obj != null ) return obj; // Found it
}
}
return null;
}
/**
* Searches an original data object in a given unit.
* @param unit the unit where to search.
* @param dataId the id of the data to search for.
* @return the object if found or null if not found.
*/
static public Object searchData (Unit unit,
String dataId)
{
Tags markers = unit.getStore().getSourceTags();
for ( Tag m : markers ) {
if ( m.isMarker() ) continue;
CTag cm = (CTag)m;
if ( cm.getDataRef().equals(dataId) ) {
return cm;
}
}
markers = unit.getStore().getTargetTags();
for ( Tag m : markers ) {
if ( m.isMarker() ) continue;
CTag cm = (CTag)m;
if ( cm.getDataRef().equals(dataId) ) {
return cm;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy